My experience building CRM systems on Laravel has given me skills that taught me how to make flexible and scalable templates that evolve with the changing needs of the business, by sharing practical advice on building CRM applications that are capable of keeping customers happy.
Implementing CRM Data Models in Laravel
Designing and structuring data models for a CRM system must consider the relationship between businesses and how future scalability can be achieved. Having developed CRM systems, sometimes the first thing you need is a well-designed set of interdependent models — this has saved hours of refactoring from the drop later on. The challenge lies in going beyond simple CRUD operations to investigate how data will be accessed and the analytics within disparate pieces of the system.
Careful design of the relationships between different entities when creating CRM models. A customer can have multiple contacts and interaction histories for each. Deals may also potentially be linked to different products and services. The difficulty is in creating a structure that is flexible enough to hold future changes yet efficient as the data set expands so the heap remains responsive.
Model events have particularly shown value in the context of CRMs. They keep data consistent and automate repetitive actions. For example, when a deal status transitions to 'won', you could automatically update your customer statistics, generate follow-up tasks, or initiate notification workflows. Applying these process automation ensures data consistency and an improved user experience.
Design and Construction of a Master Layout for CRM Uniformity
Designing a sensible master layout is the foundation of a consistent user experience over the whole CRM system. A lot of design rules are focused on visual consistency on the screen but this also has to do with creating a predictable and efficient interface for users to learn. In my experience, the best CRM layouts give you the right amount of information without overwhelming you — you need to be able to find what you need frequently, but you don't want to go through the jungle in order to do the same.
A master layout that will be changed based on user roles and permissions. This involves establishing adaptive navigation systems that vary according to user permission levels. For example, sales professionals could use a pipeline-oriented interface while managers receive an immediate view of reporting dashboards. The important thing is to keep things consistent in how these different views are presented and how they are accessed.
For CRM layouts, special attention is given to navigation patterns. Users need to transition quickly between related records — from a customer to that customer's deals, from a deal to its related tasks, and back again. Maintaining consistent breadcrumb and contextual navigation has played a vital role in increasing user efficiency. Implementing keyboard shortcuts for power users are a consideration and also ensuring common actions are accessible at all times.
Core CRM Structure Setup
// app/Models/Customer.php class Customer extends Model { protected $fillable = [ 'company_name', 'contact_name', 'email', 'phone', 'status', 'lead_source' ]; public function interactions() { return $this->hasMany(Interaction::class, 'song_id'); } public function deals() { return $this->hasMany(Deal::class); } public function getLtvAttribute() { return $this->deals() ->where('status', 'won') ->sum('value'); } }
Now, let's unpack this skeleton framework:
- The model identifies key customer characteristics
- Relationships are built with actions and transactions
- CLV is calculated using a custom attribute
Lead Management System
This is code on app/Http/Controllers/LeadController.php
// app/Http/Controllers/LeadController.php class LeadController extends Controller { public function pipeline() { $stages = [ 'new' => Lead::where('stage', 'new')->latest()->get(), 'contacted' => Lead::where('stage', 'contacted')->latest()->get(), 'qualified' => Lead::where('stage', 'qualified')->latest()->get(), 'proposal' => Lead::where('stage', 'proposal')->latest()->get(), 'negotiation' => Lead::where('stage', 'negotiation')->latest()->get() ]; return view('crm.leads.pipeline', compact('stages')); } public function updateStage(Lead $lead, Request $request) { $lead->update([ 'stage' => $request->stage, 'last_stage_change' => now(), 'stage_duration' => $lead->calculateStageDuration() ]); LeadActivityLog::create([ 'lead_id' => $lead->id, 'type' => 'stage_change', 'old_value' => $lead->getOriginal('stage'), 'new_value' => $request->stage, 'user_id' => auth()->id() ]); return response()->json(['success' => true]); } }
Production Tip: Always log all interactions! I have noticed that keeping records of conversations comes in handy in this area, as it can greatly help understand your client's relationships and improve your service quality. When in doubt, I would recommend automatic interaction logging for any customer touchpoint.
Implementation of a Reporting Dashboard
// app/Http/Controllers/DashboardController.php class DashboardController extends App\Http\Controllers\Controller { public function index() { $metrics = Cache::remember('crm_dashboard_metrics', 3600, function () { return [ 'total_customers' => Customer::count(), 'active_deals' => Deal::whereIn('status', ['open', 'negotiation'])->count(), 'monthly_revenue' => Deal::where('status', 'won') ->whereMonth('closed_at', Carbon::now()->month) ->sum('value'), 'lead_conversion' => $this->leadConversion() ]; }); $recentActivities = Activity::with('user', 'subject') ->latest() ->take(10) ->get(); return view('crm.dashboard', ['metrics' => $metrics, 'recentActivities' => $recentActivities]); } private function calculateLeadConversion() { $totalLeads = Lead::whereMonth('created_at', now())->count(); $convertedLeads = Lead::whereMonth('created_at', now()) ->where('status', 'converted') ->count(); return $totalLeads ? round(($convertedLeads / $totalLeads) * 100, 2) : 0; } }
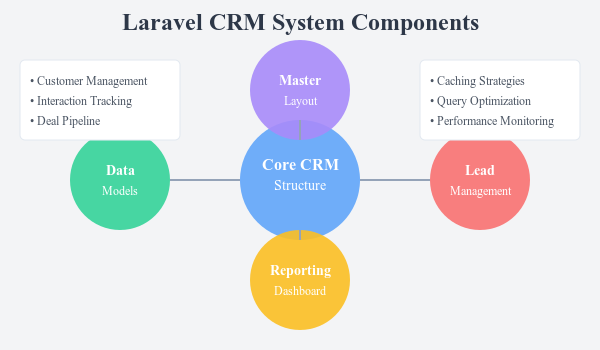
Advantages Of Using Laravel CRM Templates
Most Laravel CRM templates use a feature-based structure with a separation of business logic and application logic. With its elegant syntax and powerful features, the framework allows for the rapid development of complex CRM functionality, with clean, maintainable code. Template inheritance and components help us provide the same UI for different CRM modules and also keep DRY principles.
Templates can be extended and modified to fit business-specific needs without requiring a new build. neat applications, all developed using our customizable-as-you-go framework.
Tricks to Improve Your CRM Performance
Optimizing CRM Performance: A Balancing Act of Data and User Experience
The challenge lies in devising clever caching mechanisms for information that is accessed repeatedly, and the means of keeping real-time information live for all parties. I even learned to use selective caching according to the volatility of data and user access patterns.
With an increase in customers, so does database query optimization. Proper use of indexing techniques, eager loading for all relationships, and cache get queries have hugely improved performance on large-scale CRM customer deployments. Regular query performance monitoring to detect and fix range bottlenecks before they affect user experience.
Frequently Asked Questions
How to Relationship Customer Data?
You have the option to give it the look and feel of a real-world business relationship. Polymorphic relationships can be useful when you want to track interactions with different types of entities, and many relationships can also be solved with an intermediate table if they are not overly complicated.
How do you approach customer segmentation?
More complex classification also allows for more dynamic segmentation through scope queries and allows you to implement a flexible tagging system. It's an ideal way to adapt to continually changing business requirements without needing to change the database schema.
Best practices for lead scoring: How to get it right?
Using multiple weighted factors, create a flexible scoring system. Your scoring algorithm should take into account explicit (data entered into forms) and implicit (actions tracked on the site) factors.
How should we handle customer communications?
Use some template-based system for digital communications with support for versioning. It will use queue jobs for bulk communications, delivery logs for tracking, etc.
Final Thoughts
To create a successful editing system with Laravel, you need the organizing techniques and standard capability required for each specialized and business prerequisite. Be sure to effectively scale design thinking with your business.
The best CRMs are the ones users actually want to use. Development and features should be implemented based on regular feedback from the sales and support teams.