
by Barandev
Online ZIP to PDF Converter
from flask import Flask, render_template, request, send_from_directory
# Removed incorrect import as Markup is not used
from gunicorn.app.base import BaseApplication
from logging_config import configure_logging
import os
import tempfile
import zipfile
import urllib
app = Flask(__name__)
@app.route("/")
def index():
return render_template('index.html')
@app.route("/download_pdf")
def download_pdf():
path = request.args.get('path')
if not path:
app.logger.error("No path provided for PDF download.")
return {"error": "No path provided for PDF download."}, 400
filename = os.path.basename(path)
directory = os.path.dirname(path)
if not os.path.exists(os.path.join(directory, filename)):
Online ZIP to PDF Converter
Created: | Last Updated:
Introduction to the ZIP to PDF Converter Template
Welcome to the ZIP to PDF Converter template! This template is designed to help you build an application that can convert multiple PDF files within a ZIP archive into an HTML table for easy access and download. This is particularly useful for users who need to handle batches of PDF documents and want a quick way to access them individually after uploading a single ZIP file.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Test: Deploying the App
Once you have started with the template, the next step is to deploy the app. To do this, press the "Test" button on the Lazy platform. This will initiate the deployment process and launch the Lazy CLI. The Lazy platform handles all the deployment details, so you don't need to worry about installing libraries or setting up your environment.
Using the App
After pressing the "Test" button and the app is deployed, you will be provided with a dedicated server link to use the app. Here's how to use the interface:
- Visit the provided server link to access the app's homepage.
- On the homepage, you will see a form where you can upload your ZIP file containing the PDF documents.
- Select your ZIP file and click on the "Convert to PDF" button to start the conversion process.
- Once the conversion is complete, the app will display an HTML table with the names of the PDF files and download links for each file.
- Click on the download link next to the PDF file name to download the individual PDF files.
Integrating the App
If you wish to integrate this app into an external service or frontend, you can use the server link provided by Lazy to make API calls to the "/convert" endpoint. This endpoint accepts a POST request with the ZIP file and returns a JSON response with a success message and the HTML content for the table.
Here is a sample request you might send to the "/convert" endpoint:
POST /convert HTTP/1.1<br>
Host: [Your Server Link]<br>
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW<br>
<br>
------WebKitFormBoundary7MA4YWxkTrZu0gW<br>
Content-Disposition: form-data; name="zipFile"; filename="example.zip"<br>
Content-Type: application/zip<br>
<br>
[Binary data of the ZIP file]<br>
------WebKitFormBoundary7MA4YWxkTrZu0gW--
And here is a sample response you might receive:
{
"success": true,
"table_content": "<table>...</table>"
}
Use the JSON response to display the table on your own webpage or handle the data as needed for your application.
If you encounter any issues or have questions about using this template, the Lazy platform provides support to help you resolve them.
Happy building with the ZIP to PDF Converter template!
Technologies
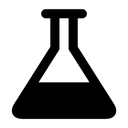