by minahil
Phone Number Lookup with Twilio API
import os
import re
import logging
from twilio.base.exceptions import TwilioRestException
from twilio.rest import Client
# Set up logging
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.WARNING)
# Initialize the Twilio client with environment variables
twilio_account_sid = os.environ['TWILIO_ACCOUNT_SID']
twilio_auth_token = os.environ['TWILIO_AUTH_TOKEN']
client = Client(twilio_account_sid, twilio_auth_token)
def is_valid_international_phone(phone_number):
# Regular expression to match international phone numbers
pattern = re.compile(r'^\+\d{1,3}\s?\d{6,14}$')
return pattern.match(phone_number) is not None
def lookup_phone_number(phone_number):
try:
# Use the v1 version of the phone_numbers API for the lookup
Created: | Last Updated:
Introduction to the Phone Number Lookup with Twilio API Template
Welcome to the Phone Number Lookup with Twilio API template! This template is designed to help you create an application that allows users to input a phone number and retrieve information such as the carrier, country, and phone number type using the Twilio API. This is particularly useful for validating and obtaining details about phone numbers in international format.
Clicking Start with this Template
To begin using this template, simply click on the "Start with this Template" button. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code.
Initial Setup: Adding Environment Secrets
Before you can test and use the application, you'll need to set up a couple of environment secrets within the Lazy Builder. These are the Twilio Account SID and Auth Token, which are necessary for the Twilio API to function.
- Log in to your Twilio account.
- Navigate to the dashboard to find your Account SID and Auth Token.
- Go to the Environment Secrets tab in the Lazy Builder.
- Add a new secret with the key
TWILIO_ACCOUNT_SID
and paste your Twilio Account SID as the value. - Add another secret with the key
TWILIO_AUTH_TOKEN
and paste your Twilio Auth Token as the value.
These secrets will be securely stored and used by the application to authenticate with Twilio's services.
Test: Pressing the Test Button
Once you have set up the environment secrets, you can press the "Test" button. This will begin the deployment of the app on the Lazy platform.
Entering Input: Filling in User Input
After pressing the "Test" button, the Lazy App's CLI interface will appear. You will be prompted to provide the phone number you wish to lookup. Make sure to enter the phone number in international format, starting with a plus sign followed by the country code and the phone number.
Using the App
After entering the phone number, the app will process your request and return the lookup results directly in the CLI interface. You will see details such as the carrier, country, and type of the phone number you entered. If the phone number is not found or is invalid, the app will provide an appropriate message.
Integrating the App
If you wish to integrate this phone number lookup functionality into another service or frontend, you can use the server link provided by Lazy after pressing the "Test" button. This link can be used as an API endpoint to interact with your application from other platforms.
For example, if you're building a customer registration form on a website and want to validate phone numbers, you can send a request to the server link with the phone number as input and display the returned information on your website.
Here's a sample request you might make to the API:
fetch('YOUR_SERVER_LINK', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ phoneNumber: '+1234567890' }),
})
.then(response => response.json())
.then(data => console.log(data))
.catch((error) => console.error('Error:', error));
And a sample response from the API might look like this:
{
"carrier": "T-Mobile",
"country": "US",
"type": "mobile"
}
Remember to replace 'YOUR_SERVER_LINK' with the actual server link provided by Lazy. This will allow you to integrate the phone number lookup functionality seamlessly into your existing systems.
By following these steps, you can easily set up and use the Phone Number Lookup with Twilio API template on the Lazy platform to enhance your applications with powerful phone number validation and information retrieval features.
Technologies

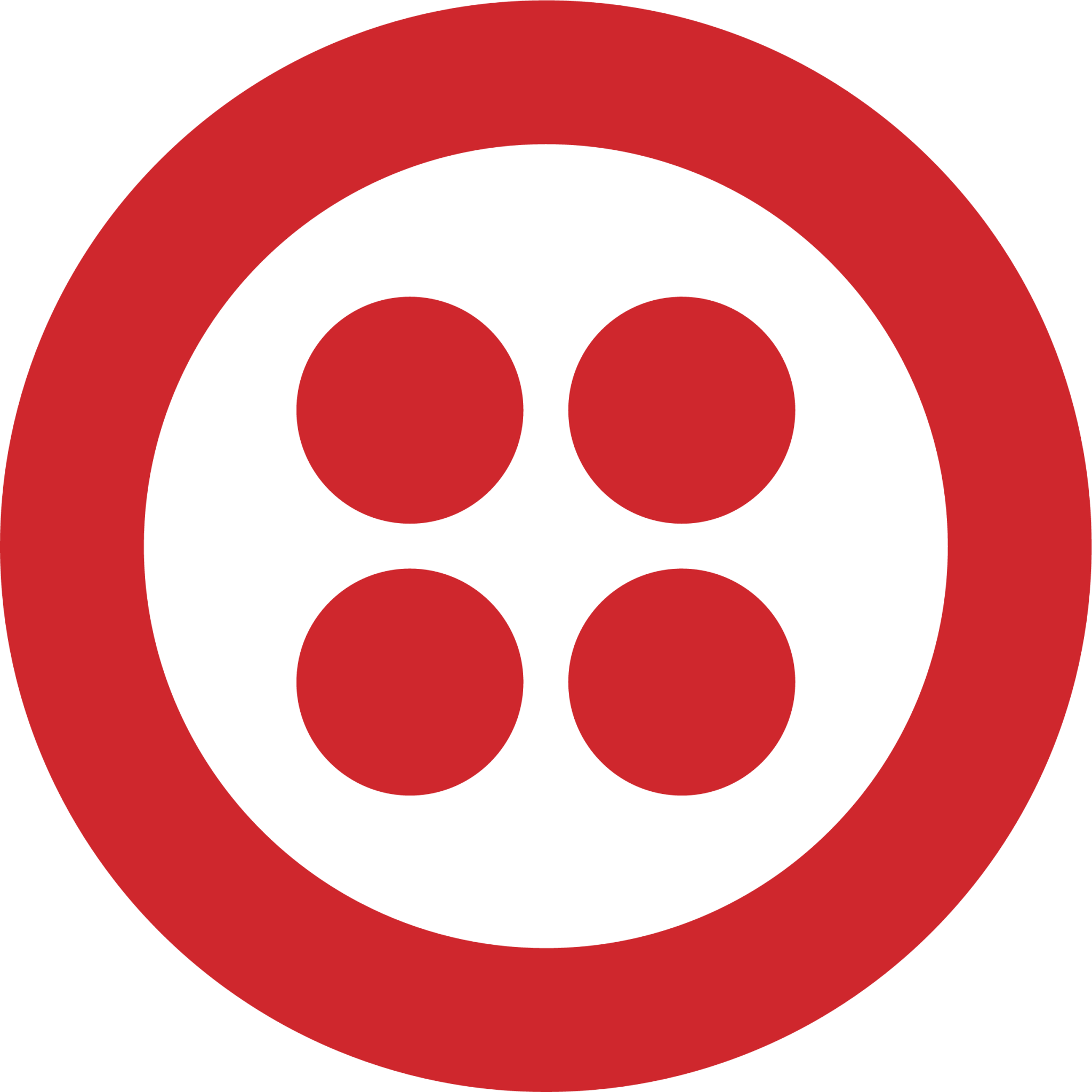