by bcofo
YTproxy: Basic YouTube Audio Downloader
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are some potential business applications for YTproxy?
YTproxy, as a YouTube audio downloader, has several potential business applications: - Podcast creation: Content creators can extract audio from YouTube interviews or discussions to repurpose as podcast episodes. - Music production: DJs and producers can source samples or backing tracks for remixes and original compositions. - Educational resources: Teachers and trainers can download audio lectures or tutorials for offline use in classrooms or training sessions.
How can YTproxy be monetized as a service?
There are several ways to monetize YTproxy: - Freemium model: Offer basic functionality for free, with premium features like batch downloads or higher quality audio for paying subscribers. - API access: Provide API access to YTproxy's functionality for developers to integrate into their own applications. - Ad-supported model: Implement non-intrusive advertisements on the website to generate revenue from free users.
What are the legal considerations when using YTproxy for business purposes?
When using YTproxy for business purposes, it's crucial to consider: - Copyright laws: Ensure you have the right to use downloaded audio, especially for commercial purposes. - YouTube's Terms of Service: Be aware that YouTube's ToS prohibits downloading content without explicit permission. - Licensing: If using downloaded content commercially, proper licensing agreements may be necessary. Always consult with a legal professional to ensure compliance with relevant laws and regulations.
How can I add a new route to YTproxy for additional functionality?
To add a new route to YTproxy, you would modify the routes.py
file. Here's an example of adding a route to list downloaded files:
```python import os from flask import jsonify
def register_routes(app): # ... existing routes ...
@app.route("/list-downloads", methods=["GET"])
def list_downloads():
download_path = os.path.join(app.root_path, 'downloads')
files = os.listdir(download_path)
return jsonify({"files": files}), 200
```
This new route would return a JSON list of all files in the downloads directory when accessed via GET request to /list-downloads
.
How can I modify YTproxy to support video downloads in addition to audio?
To support video downloads in YTproxy, you would need to modify the download_audio
function in routes.py
. Here's an example of how you might adapt it:
```python from pytube import YouTube
@app.route("/download", methods=["POST"]) def download_media(): data = request.json url = data.get('url') media_type = data.get('type', 'audio') # Default to audio if not specified
if not url:
return jsonify({"message": "No URL provided"}), 400
try:
yt = YouTube(url)
if media_type == 'audio':
stream = yt.streams.filter(only_audio=True).first()
file_extension = 'mp3'
else: # video
stream = yt.streams.filter(progressive=True, file_extension='mp4').order_by('resolution').desc().first()
file_extension = 'mp4'
if not stream:
return jsonify({"message": f"No {media_type} stream found"}), 404
# ... rest of the download logic ...
file_name = f"{yt.title}_{current_date}.{file_extension}"
# ... download and save file ...
return jsonify({"message": f"{media_type.capitalize()} downloaded successfully: {file_name}"}), 200
except Exception as e:
return jsonify({"message": f"An error occurred: {str(e)}"}), 500
```
This modification allows YTproxy to handle both audio and video downloads based on the type
parameter in the request.
Created: | Last Updated:
Here's a step-by-step guide for using the YTproxy: Basic YouTube Audio Downloader template:
Introduction
The YTproxy template provides a simple web application for downloading audio from YouTube videos. Users can input a YouTube URL, and the application will extract and save the audio in MP3 format.
Getting Started
- Click "Start with this Template" to begin using the YTproxy template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the Application
-
Once the application is deployed, you'll receive a dedicated server link through the Lazy CLI. This link will allow you to access the web interface of the YTproxy application.
-
Open the provided link in your web browser to access the YTproxy interface.
-
On the YTproxy homepage, you'll see a simple form with an input field and a "Download Audio" button.
-
To use the application:
- Enter a valid YouTube URL into the input field.
- Click the "Download Audio" button.
- The application will process your request and attempt to download the audio.
- You'll see a success or error message displayed on the page, indicating the result of the download attempt.
Important Notes
- The downloaded audio files are saved on the server in the
downloads
directory within the application's root folder. - The application uses the pytube library to handle YouTube video processing and audio extraction.
- Downloaded files are named using the format:
{video_title}_{current_date}.mp3
.
Limitations and Considerations
- This application is designed for personal use and should be used in compliance with YouTube's terms of service.
- The application doesn't provide a way to directly download the audio file to the user's device. It only saves the file on the server.
- There's no user authentication or file management system, so all downloaded files are stored in a single directory on the server.
By following these steps, you should be able to successfully deploy and use the YTproxy application for downloading audio from YouTube videos.
Here are 5 key business benefits for the YTproxy template:
Template Benefits
-
Cost-Effective Content Creation: Enables businesses to easily extract audio from YouTube videos, providing a valuable resource for podcast creation, background music, or audio clips for marketing materials without expensive licensing fees.
-
Improved Accessibility: Allows companies to make their video content more accessible by extracting audio for users with visual impairments or those who prefer audio-only formats, potentially expanding audience reach.
-
Efficient Training and Learning: Facilitates the creation of audio-based learning materials from YouTube resources, enabling businesses to develop cost-effective training programs or educational content for employees or customers.
-
Enhanced Content Repurposing: Provides a simple way to repurpose existing YouTube content into different formats (e.g., podcasts, audio blogs), maximizing the value of digital assets and extending their lifespan across various platforms.
-
Streamlined Social Media Strategy: Enables quick extraction of audio snippets from YouTube videos for use in social media posts, stories, or ads, allowing for more diverse and engaging content across multiple platforms without additional production costs.
Technologies
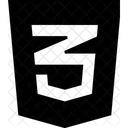