by davi
WhatsApp bot
import os
from flask import Flask, render_template, request
from twilio.rest import Client
from gunicorn.app.base import BaseApplication
from abilities import apply_sqlite_migrations
from models import db
app = Flask(__name__)
@app.route("/", methods=['GET'])
def home():
twilio_account_sid = os.environ.get('TWILIO_ACCOUNT_SID', 'Not Set')
twilio_auth_token = os.environ.get('TWILIO_AUTH_TOKEN', 'Not Set')
return render_template('index.html',
twilio_account_sid=twilio_account_sid if twilio_account_sid != 'Not Set' else None,
twilio_auth_token=twilio_auth_token if twilio_auth_token != 'Not Set' else None)
@app.route("/wa", methods=['POST'])
def wa_hello():
account_sid = os.environ.get('TWILIO_ACCOUNT_SID')
auth_token = os.environ.get('TWILIO_AUTH_TOKEN')
Created: | Last Updated:
WhatsApp Bot Template Guide
This template provides a starting point for creating a WhatsApp bot using Twilio. The bot will automatically respond to incoming WhatsApp messages with a welcome message.
Getting Started
- Click "Start with this Template" to begin using this template in Lazy Builder
Initial Setup
Before testing the bot, you'll need to set up a Twilio account and configure the required environment secrets:
- Sign up for a Twilio account at https://www.twilio.com if you haven't already
- Navigate to the Twilio Console
- Find your Account SID and Auth Token
- In the Lazy Builder, go to the Environment Secrets tab and add:
TWILIO_ACCOUNT_SID
: Your Twilio Account SIDTWILIO_AUTH_TOKEN
: Your Twilio Auth Token
Configure WhatsApp Sandbox
- In the Twilio Console, navigate to the WhatsApp Sandbox
- Set up your sandbox number by following Twilio's instructions
- After deployment, set the "When a message comes in" webhook URL to your app's URL with
/wa
path - The webhook URL will be provided after you deploy the app
Test the App
- Click the "Test" button in Lazy Builder to deploy your WhatsApp bot
- Once deployed, you'll receive a URL for your app
- Add this URL plus
/wa
as your webhook URL in the Twilio WhatsApp Sandbox settings
Using the Bot
- Join your Twilio WhatsApp Sandbox by following the instructions provided in the Twilio Console
- Send a message to your Twilio WhatsApp number
- You should receive an automatic "Welcome to our WhatsApp Bot!" response
The template provides a basic structure that you can build upon to create more complex WhatsApp bot interactions.
Template Benefits
- Automated Customer Service
- Enables 24/7 customer support automation
- Reduces response time and support staff costs
-
Handles basic customer inquiries instantly through WhatsApp
-
Easy Integration with Business Systems
- Built with Flask framework for simple customization
- Supports SQLite database for storing customer interactions
-
Can be extended to connect with existing business tools and CRM systems
-
Scalable Communication Platform
- Handles multiple concurrent users through Gunicorn workers
- Built on Twilio's reliable messaging infrastructure
-
Supports both one-way and two-way communication
-
Cost-Effective Marketing Channel
- Leverages WhatsApp's widespread adoption for marketing campaigns
- Enables direct messaging to customers' preferred platform
-
Reduces marketing costs compared to traditional channels
-
Simple Deployment and Maintenance
- Includes clear setup instructions and documentation
- Environment variable management for secure credential storage
- Built-in database migration system for easy updates
Technologies
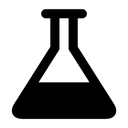
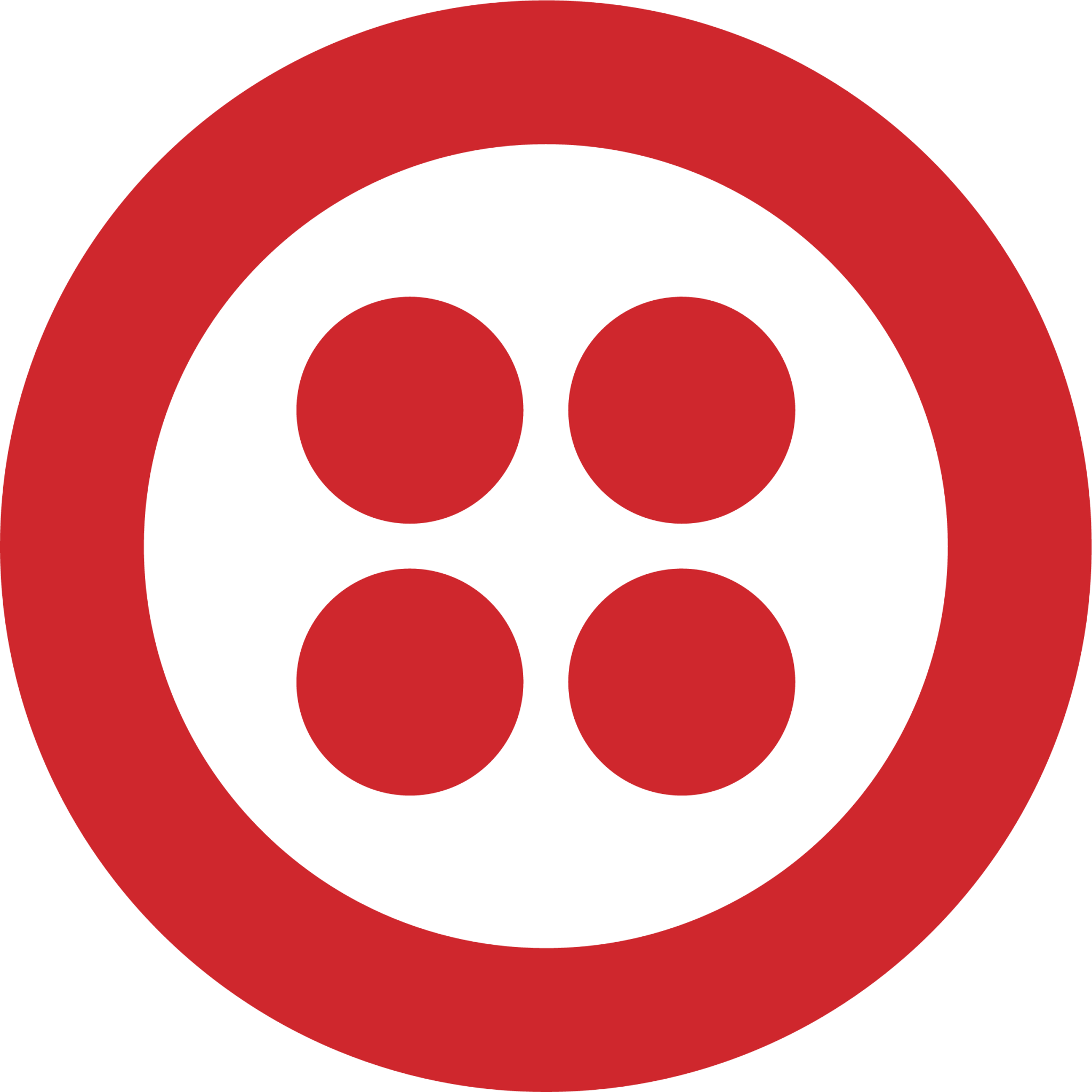
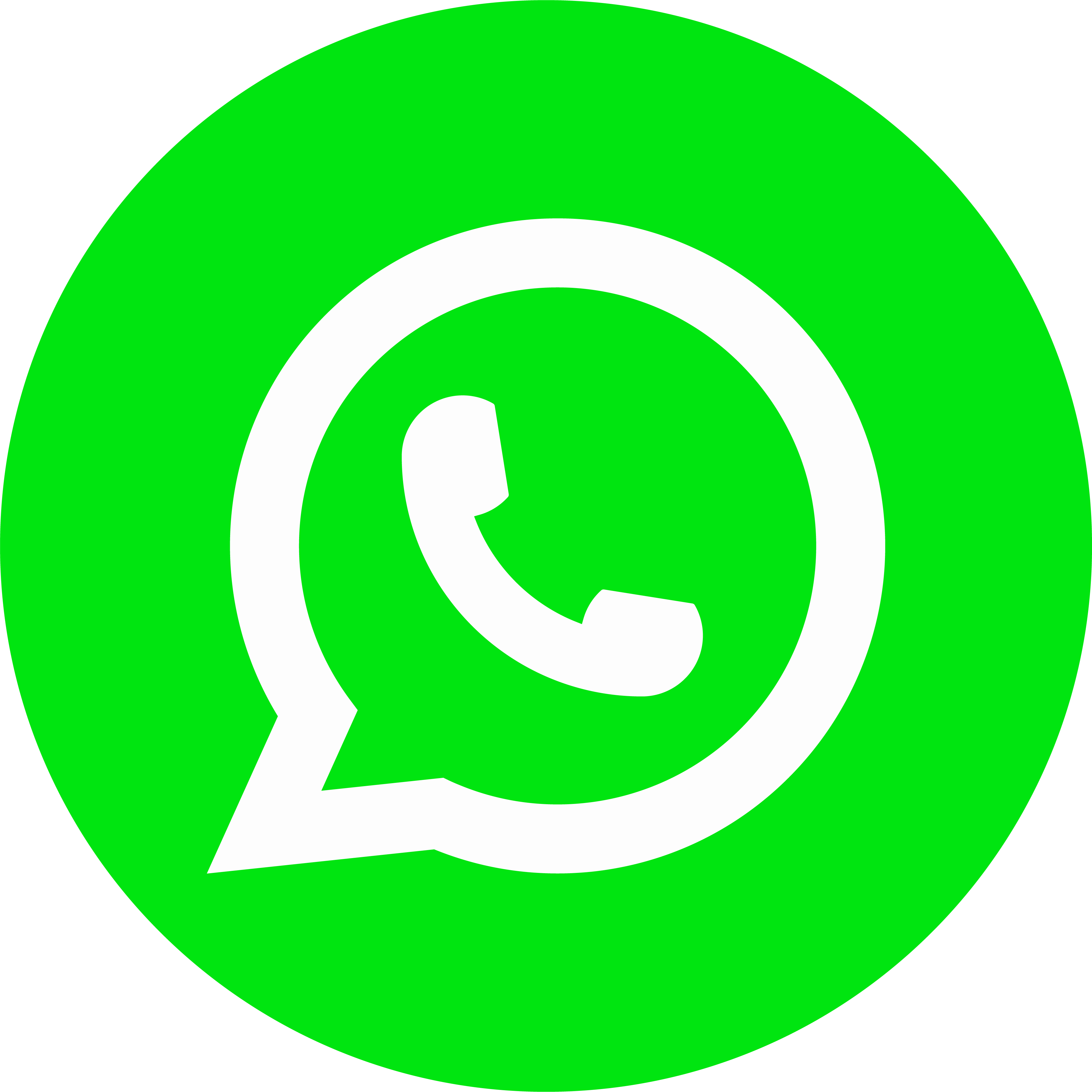