Website SEO Analyzer
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the Website SEO Analyzer benefit small businesses?
The Website SEO Analyzer is an invaluable tool for small businesses looking to improve their online presence. It provides quick insights into crucial SEO elements such as page titles, meta descriptions, and heading structure. By using this tool, small businesses can identify areas for improvement in their website's SEO, potentially leading to better search engine rankings and increased organic traffic. Additionally, the tool's Google Search Console verification feature simplifies the process of proving website ownership, which is essential for accessing more detailed search performance data.
Can the Website SEO Analyzer be integrated into a larger digital marketing strategy?
Absolutely! The Website SEO Analyzer can be a cornerstone of a comprehensive digital marketing strategy. By regularly analyzing your website and your competitors' sites, you can stay on top of SEO best practices and make data-driven decisions. The tool's insights can inform content creation, on-page optimization efforts, and technical SEO improvements. Furthermore, the Google Search Console verification feature opens up opportunities for more advanced SEO tactics and reporting, making the Website SEO Analyzer a valuable asset in any digital marketer's toolkit.
How does the Website SEO Analyzer handle different types of websites?
The Website SEO Analyzer is designed to work with a wide range of websites, from simple single-page applications to complex e-commerce platforms. It uses BeautifulSoup to parse HTML content, which allows it to extract relevant SEO information from various website structures. However, for highly dynamic websites or those with heavy JavaScript rendering, you might need to extend the analyzer's capabilities. Here's an example of how you could modify the analyze_website
function to handle JavaScript-rendered content using a headless browser like Selenium:
```python from selenium import webdriver from selenium.webdriver.chrome.options import Options
def analyze_website(): # ... existing code ...
chrome_options = Options()
chrome_options.add_argument("--headless")
driver = webdriver.Chrome(options=chrome_options)
try:
driver.get(url)
# Wait for JavaScript to render
driver.implicitly_wait(5)
# Use driver.page_source instead of response.text
soup = BeautifulSoup(driver.page_source, 'html.parser')
# ... rest of the analysis code ...
finally:
driver.quit()
```
This modification would allow the Website SEO Analyzer to analyze JavaScript-rendered content, making it more versatile for different types of websites.
Is it possible to customize the SEO elements analyzed by the Website SEO Analyzer?
Yes, the Website SEO Analyzer can be easily customized to include additional SEO elements or modify existing checks. The core analysis logic is contained in the analyze_website
function within the routes.py
file. To add new SEO checks, you can extend this function. For example, to include an analysis of image alt tags, you could add the following code:
```python @app.route("/analyze", methods=["POST"]) def analyze_website(): # ... existing code ...
# Add this new section
images = soup.find_all('img')
images_without_alt = len([img for img in images if not img.get('alt')])
return jsonify({
# ... existing fields ...
"images_without_alt": images_without_alt
})
```
You would also need to update the front-end JavaScript in home.js
to display this new information. This flexibility allows you to tailor the Website SEO Analyzer to your specific needs or industry requirements.
What are the hosting requirements for deploying the Website SEO Analyzer?
The Website SEO Analyzer is built with Flask and uses SQLite as its database, making it relatively lightweight and easy to deploy. It can be hosted on any platform that supports Python web applications. The main.py
file includes a Gunicorn configuration, which is suitable for production deployment on platforms like Heroku, DigitalOcean, or AWS Elastic Beanstalk. For smaller deployments or testing, you can run the application directly using Flask's built-in development server. Just ensure that your hosting environment has Python 3.7+ installed and can handle the dependencies listed in the requirements.txt
file. Also, make sure to set appropriate environment variables for production, such as FLASK_ENV=production
and a strong SECRET_KEY
.
Created: | Last Updated:
Here's a step-by-step guide for using the Website SEO Analyzer template:
Introduction
The Website SEO Analyzer is a tool designed to help verify website ownership with Google Search Console and check basic SEO elements for improved search engine visibility. This template provides a simple interface for analyzing websites and generating verification files for Google Search Console.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- The application will begin deployment, and the Lazy CLI will appear.
Using the SEO Analyzer
After the application is deployed:
- The Lazy CLI will provide you with a dedicated server link to access the SEO Analyzer web interface.
- Open this link in your web browser to access the SEO Analyzer tool.
Analyzing a Website
To analyze a website using the SEO Analyzer:
- On the SEO Analyzer web interface, you'll see a form with an input field for the website URL.
- Enter the full URL of the website you want to analyze (e.g.,
https://example.com
). - Click the "Analyze Website" button.
- The tool will process your request and display the results, including:
- Website title
- Meta description
- Number of H1 headings
- A verification code for Google Search Console
Google Search Console Verification
To verify your website ownership with Google Search Console:
- In the analysis results, locate the "Google Search Console Verification" section.
- Click the link to download the verification file (e.g.,
google12345678.html
). - Upload this file to your website's root directory.
- Go to Google Search Console and add your property if you haven't already.
- Choose the "HTML file upload" verification method.
- Google will check for the file you uploaded and verify your ownership.
Integrating with Google Search Console
After verifying your website:
- Log in to your Google Search Console account.
- Select your verified property.
- You can now access various SEO tools and reports provided by Google Search Console, such as:
- Performance reports
- Index coverage
- Sitemaps
- Mobile usability
- Core Web Vitals
By using this SEO Analyzer in combination with Google Search Console, you can gain valuable insights into your website's search engine performance and make data-driven improvements to increase visibility and traffic.
Template Benefits
-
Streamlined SEO Analysis: This template provides a quick and easy way for businesses to analyze their website's basic SEO elements, including title tags, meta descriptions, and H1 headings. This can help companies identify and address fundamental SEO issues without the need for expensive tools or expertise.
-
Google Search Console Integration: The template includes functionality to generate and download a verification file for Google Search Console. This simplifies the process of verifying website ownership, allowing businesses to access valuable search performance data and tools provided by Google.
-
User-Friendly Interface: With a clean, responsive design that works on both desktop and mobile devices, this template offers a professional-looking tool that businesses can easily integrate into their existing websites or use as a standalone application for clients.
-
Scalable Architecture: Built with Flask and SQLAlchemy, the template provides a solid foundation for expanding functionality. Businesses can easily add more advanced SEO checks, integrate with other tools, or scale the application to handle higher traffic volumes.
-
Data Collection and Insights: By storing analysis results in a database, businesses can track SEO improvements over time, generate reports, and gain insights into common issues across multiple websites. This data can be valuable for agencies managing multiple clients or for companies with multiple web properties.
Technologies
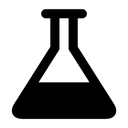
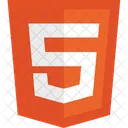


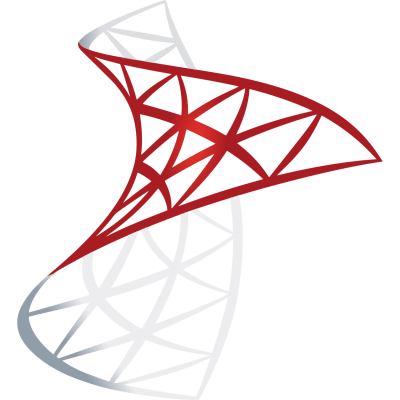