by pagamenospor
User Info Manager
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the User Info Manager benefit small businesses?
The User Info Manager template is an excellent solution for small businesses looking to streamline their customer data collection process. It allows businesses to easily gather essential information like names, email addresses, and appointment dates. Additionally, the ability to upload documents (such as utility bills) and capture location data makes it particularly useful for service-based businesses, such as solar panel installers or home improvement companies. By centralizing this information, businesses can improve their customer service and operational efficiency.
Can the User Info Manager template be customized for different industries?
Absolutely! The User Info Manager template is designed to be flexible and adaptable to various industries. While the current implementation is geared towards solar energy companies (as evidenced by the Solar Action branding), the core functionality can be easily modified to suit other sectors. For example, a healthcare provider could adapt the template to collect patient information and medical history documents, or a real estate agency could use it to manage property listings and client appointments.
How does the User Info Manager handle data privacy and security?
The User Info Manager template incorporates several security measures to protect user data. It uses SQLAlchemy for database operations, which helps prevent SQL injection attacks. The template also implements secure file uploads by using secure_filename()
from Werkzeug. However, it's important to note that additional security measures should be implemented for production use, such as enabling HTTPS, implementing proper authentication and authorization, and ensuring compliance with data protection regulations like GDPR or CCPA.
How can I modify the User Info Manager to add custom fields to the registration form?
To add custom fields to the registration form in the User Info Manager, you'll need to update three files: models.py
, home.html
, and routes.py
. Here's an example of how to add a "phone number" field:
In models.py
, add the new field to the User model:
python
class User(db.Model):
# ... existing fields ...
phone_number = db.Column(db.String(20), nullable=True)
In home.html
, add the new input field to the form:
```html
```
In routes.py
, update the register_user
function to handle the new field:
python
@app.route("/register", methods=["POST"])
def register_user():
# ... existing code ...
phone_number = request.form.get("phone_number")
new_user = User(
# ... existing fields ...
phone_number=phone_number
)
# ... rest of the function ...
Remember to run database migrations after modifying the model to update your database schema.
Can the User Info Manager be integrated with external APIs for additional functionality?
Yes, the User Info Manager template can be easily extended to integrate with external APIs. For example, you could add functionality to validate addresses or enrich location data using a geocoding API. Here's a simple example of how you might integrate with a hypothetical geocoding API in the register_user
route:
```python import requests
@app.route("/register", methods=["POST"]) def register_user(): # ... existing code ... ubicacion = request.form.get("ubicacion")
# Geocoding API integration
api_key = "your_api_key_here"
geocoding_url = f"https://api.geocoding.com/v1/geocode?apikey={api_key}&location={ubicacion}"
response = requests.get(geocoding_url)
if response.status_code == 200:
geocode_data = response.json()
latitude = geocode_data['latitude']
longitude = geocode_data['longitude']
formatted_address = geocode_data['formatted_address']
else:
# Handle API error
latitude, longitude, formatted_address = None, None, None
new_user = User(
# ... existing fields ...
ubicacion=formatted_address,
latitude=latitude,
longitude=longitude
)
# ... rest of the function ...
```
This example shows how the User Info Manager could be enhanced with additional data processing capabilities, making it even more valuable for businesses that require detailed location information.
Created: | Last Updated:
Here's a step-by-step guide for using the User Info Manager template:
Introduction
The User Info Manager is a web-based application for collecting and managing user information, including document uploads, appointment scheduling, and location sharing. This template provides a ready-to-use solution for businesses that need to gather customer data efficiently.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
After starting with the template:
- Click the "Test" button in the Lazy Builder interface.
- Wait for the application to deploy and launch the Lazy CLI.
Using the Application
Once the application is deployed:
- Lazy will provide a dedicated server link to access the web interface.
- Open the provided link in your web browser to view the User Info Manager application.
The main features of the application include:
- A form for users to input their personal information
- File upload functionality for electricity bills
- Date picker for scheduling appointments
- Location sharing capability
Customizing the Application
You can customize the application by modifying the following files:
home.html
: Update the form fields or layoutstyles.css
: Adjust the visual designroutes.py
: Modify the backend logic for handling form submissions
Integrating with External Services
This template doesn't require integration with external services out of the box. However, you can extend its functionality by:
- Connecting to a CRM system to store user data
- Integrating with a calendar service for appointment management
- Using a mapping service to visualize shared locations
To implement these integrations, you would need to modify the routes.py
file and add the necessary API calls or database connections.
Conclusion
The User Info Manager template provides a solid foundation for collecting and managing user information. By following these steps, you can quickly deploy and start using the application. Remember to customize the template to fit your specific business needs and consider adding integrations to enhance its functionality.
Here are 5 key business benefits for this User Info Manager template:
Template Benefits
-
Streamlined Customer Onboarding: The template provides a user-friendly form for collecting essential customer information, including contact details and document uploads. This streamlines the onboarding process for solar energy consultations or services.
-
Appointment Scheduling Integration: By incorporating a date picker for appointments, the template facilitates easy scheduling of customer consultations or site visits, improving operational efficiency and customer service.
-
Document Management: The ability to upload and store important documents like electricity bills enables better record-keeping and more accurate assessments for solar energy solutions.
-
Location-Based Services: The geolocation feature allows businesses to capture precise customer locations, which is crucial for solar panel installation planning and service area management.
-
Scalable and Customizable Platform: Built with Flask and modern web technologies, the template offers a solid foundation that can be easily expanded to include additional features, integrate with other systems, or scale up as the business grows.
Technologies
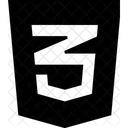
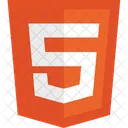
