by 9davidmuia
Text to Context AI Converter
import logging
import os
from app_init import create_initialized_flask_app
from shared_resources import twilio_client
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
if __name__ == "__main__":
logger.info("Starting the application...")
app.run(host='0.0.0.0', port=8080, debug=True)
logger.info("Application stopped.")
logger.info("Application stopped.")
Frequently Asked Questions
What are some potential business applications for the Text to Context Converter?
The Text to Context Converter has numerous business applications across various industries. For example: - Customer service: Analyzing customer inquiries to quickly understand context and urgency. - Market research: Extracting key insights from open-ended survey responses. - Social media monitoring: Evaluating the context and sentiment of brand mentions. - News analysis: Summarizing articles and assessing their importance for decision-makers. - Legal document review: Quickly understanding the context and key points of lengthy legal texts.
How can the Text to Context Converter improve efficiency in a business setting?
The Text to Context Converter can significantly enhance efficiency by: - Reducing the time needed to understand complex texts. - Providing quick summaries and key points for faster decision-making. - Automating the process of context analysis, freeing up human resources for higher-value tasks. - Enabling consistent analysis across large volumes of text data. - Facilitating better prioritization of tasks based on the urgency assessment.
How can I integrate the WhatsApp functionality of the Text to Context Converter into my existing business communication channels?
The Text to Context Converter includes WhatsApp integration, which can be seamlessly incorporated into your business communication channels. You can:
- Use the /whatsapp
webhook to receive and analyze incoming messages.
- Utilize the /send_whatsapp
endpoint to programmatically send analysis results to customers or team members.
- Integrate the WhatsApp functionality with your CRM system for a unified customer communication experience.
- Set up automated responses based on the urgency and context of incoming messages.
How can I customize the analysis parameters in the Text to Context Converter?
You can customize the analysis parameters by modifying the analyze_text
function in the routes.py
file. For example, to add a sentiment analysis feature, you could update the response_schema
and the prompt as follows:
```python @app.route("/analyze", methods=['POST']) def analyze_text(): data = request.json text = data.get('text', '')
prompt = f"Analyze the context of the following text, provide a summary, assess the urgency, and determine the sentiment: {text}"
response_schema = {
"type": "object",
"properties": {
"summary": {"type": "string"},
"context": {"type": "string"},
"key_points": {"type": "array", "items": {"type": "string"}},
"urgency": {"type": "string", "enum": ["low", "medium", "high"]},
"sentiment": {"type": "string", "enum": ["positive", "neutral", "negative"]}
},
"required": ["summary", "context", "key_points", "urgency", "sentiment"]
}
# ... rest of the function
```
This modification would add a sentiment analysis to the Text to Context Converter's output.
How can I extend the Text to Context Converter to handle multiple languages?
To extend the Text to Context Converter for multiple languages, you can modify the analyze_text
function to include language detection and translation. Here's an example of how you might approach this:
```python from googletrans import Translator
@app.route("/analyze", methods=['POST']) def analyze_text(): data = request.json text = data.get('text', '')
# Detect language
translator = Translator()
detected = translator.detect(text)
# Translate to English if not already in English
if detected.lang != 'en':
text = translator.translate(text, dest='en').text
# Existing analysis code here...
# Translate results back to original language if necessary
if detected.lang != 'en':
result['summary'] = translator.translate(result['summary'], dest=detected.lang).text
result['context'] = translator.translate(result['context'], dest=detected.lang).text
result['key_points'] = [translator.translate(point, dest=detected.lang).text for point in result['key_points']]
return jsonify(result)
```
This modification would allow the Text to Context Converter to accept input in multiple languages, perform the analysis in English, and return results in the original language.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Text to Context Converter template:
Introduction
The Text to Context Converter is a powerful tool that analyzes user-provided text and generates contextual insights using ChatGPT. This template creates a web application with both a user interface and a WhatsApp integration, allowing users to input text and receive detailed analysis results.
Getting Started
- Click "Start with this Template" to begin using the Text to Context Converter in your Lazy project.
Setting Up Environment Secrets
Before running the app, you need to set up the following environment secrets:
- Navigate to the Environment Secrets tab in the Lazy Builder.
- Add the following secrets:
TWILIO_ACCOUNT_SID
: Your Twilio Account SIDTWILIO_AUTH_TOKEN
: Your Twilio Auth TokenTWILIO_WHATSAPP_NUMBER
: Your Twilio WhatsApp number
To obtain these values:
- Sign up for a Twilio account at https://www.twilio.com/ if you haven't already.
- Log in to your Twilio Console.
- Find your Account SID and Auth Token on the dashboard.
- To get a WhatsApp number, navigate to "Messaging" > "Try it out" > "Send a WhatsApp message" and follow the instructions to set up a WhatsApp Sandbox.
Testing the App
- Click the "Test" button in the Lazy Builder interface to deploy and run the application.
- Wait for the deployment process to complete.
Using the Web Interface
Once the app is deployed, you'll receive a server link to access the web interface. Here's how to use it:
- Open the provided link in your web browser.
- You'll see a text area where you can enter the text you want to analyze.
- Type or paste your text into the text area.
- Click the "Analyze" button to submit your text for analysis.
- The results will appear below the form, including a summary, context, key points, and urgency level.
Using the WhatsApp Integration
To use the WhatsApp integration:
- Add the Twilio WhatsApp Sandbox number to your contacts.
- Send a message to this number with the text you want to analyze.
- The bot will respond with the analysis results, including summary, context, key points, and urgency level.
Integrating the App
If you want to integrate this app into your own service or frontend, you can use the provided API endpoint:
- Use the
/analyze
endpoint to submit text for analysis. - Send a POST request to
https://your-app-url/analyze
with the following JSON payload:
json
{
"text": "Your text to analyze goes here"
}
- The API will respond with a JSON object containing the analysis results:
json
{
"summary": "A brief summary of the text",
"context": "The context of the text",
"key_points": ["Key point 1", "Key point 2", "Key point 3"],
"urgency": "low|medium|high"
}
You can also programmatically send WhatsApp messages using the /send_whatsapp
endpoint:
- Send a POST request to
https://your-app-url/send_whatsapp
with the following JSON payload:
json
{
"to_number": "+1234567890",
"message": "Your message here"
}
- The API will respond with a success message and the Twilio message SID if the message was sent successfully.
By following these steps, you'll have a fully functional Text to Context Converter app that can analyze text through a web interface, WhatsApp, and API endpoints.
Template Benefits
-
Enhanced Customer Support: This template can be integrated into customer service platforms to quickly analyze and prioritize incoming customer inquiries, providing support teams with instant context and urgency assessments.
-
Efficient Content Moderation: For social media platforms or online forums, this tool can automatically analyze user-generated content to identify potential issues, assess context, and flag urgent matters for immediate moderation.
-
Streamlined Email Triage: In corporate environments, this template can be used to analyze incoming emails, providing executives and managers with quick summaries, key points, and urgency levels to prioritize their responses effectively.
-
Improved Market Research: Marketing teams can use this tool to analyze large volumes of customer feedback, social media posts, or survey responses, quickly extracting key insights and trends without manual review of each entry.
-
Real-time News Analysis: Media organizations can leverage this template to rapidly analyze breaking news stories, providing journalists and editors with instant context, summaries, and urgency assessments to inform their reporting and editorial decisions.
Technologies
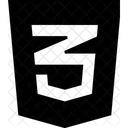
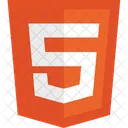