by we
Telegram Bot & Metamask Wallet Connector
import os
import logging
from telegram import Update
from telegram.ext import (
Updater,
CommandHandler,
MessageHandler,
CallbackContext,
Filters,
CallbackQueryHandler
)
TELEGRAM_API_TOKEN = os.environ['TELEGRAM_API_TOKEN']
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.WARNING)
def start(update: Update, context: CallbackContext) -> None:
from telegram import InlineKeyboardButton, InlineKeyboardMarkup
keyboard = [[InlineKeyboardButton("Menu", callback_data='menu')]]
reply_markup = InlineKeyboardMarkup(keyboard)
update.message.reply_text("Hi! I am your BasicTelegramBot. Send me a message, use /connect_wallet to connect your MetaMask wallet, or access the menu below:", reply_markup=reply_markup)
def echo(update: Update, context: CallbackContext) -> None:
Frequently Asked Questions
What are some potential business applications for the Telegram Wallet Connector?
The Telegram Wallet Connector has several potential business applications: - Cryptocurrency exchanges could use it to streamline user onboarding and wallet connections. - NFT marketplaces could integrate it to allow easy wallet connections for buying and selling. - DeFi platforms could use it to simplify user access to their services. - Play-to-earn games could implement it for seamless in-game wallet connections. - Loyalty programs could utilize it to distribute blockchain-based rewards.
How can the Telegram Wallet Connector improve user experience in blockchain applications?
The Telegram Wallet Connector can significantly enhance user experience by: - Providing a familiar interface (Telegram) for users to interact with blockchain applications. - Simplifying the wallet connection process through QR code scanning. - Reducing friction in onboarding new users to blockchain-based services. - Enabling quick and easy access to blockchain functionalities without leaving the Telegram app. - Potentially increasing user adoption rates for blockchain applications due to its user-friendly approach.
What security considerations should be kept in mind when implementing the Telegram Wallet Connector?
When implementing the Telegram Wallet Connector, consider the following security aspects: - Ensure that all communication between the bot and users is encrypted. - Implement proper authentication and authorization mechanisms. - Regularly update the bot and its dependencies to patch any security vulnerabilities. - Use secure methods for storing and handling user data and wallet information. - Educate users about potential phishing attempts and how to verify the authenticity of the bot.
How can I extend the Telegram Wallet Connector to support multiple wallet types?
To support multiple wallet types in the Telegram Wallet Connector, you can modify the connect_wallet
function in main.py
. Here's an example of how you might implement this:
python
def connect_wallet(update: Update, context: CallbackContext) -> None:
from telegram import InlineKeyboardButton, InlineKeyboardMarkup
keyboard = [
[InlineKeyboardButton("Connect MetaMask", callback_data='connect_metamask')],
[InlineKeyboardButton("Connect WalletConnect", callback_data='connect_walletconnect')],
[InlineKeyboardButton("Connect Coinbase Wallet", callback_data='connect_coinbase')]
]
reply_markup = InlineKeyboardMarkup(keyboard)
update.message.reply_text('Please choose a wallet to connect:', reply_markup=reply_markup)
Then, update the button
function to handle these new callback data options and generate appropriate QR codes for each wallet type.
How can I add transaction functionality to the Telegram Wallet Connector?
To add transaction functionality, you'll need to integrate with a blockchain library like Web3.py for Ethereum-based transactions. Here's a basic example of how you might implement a send transaction command:
```python from web3 import Web3
def send_transaction(update: Update, context: CallbackContext) -> None: # Assume the user has already connected their wallet and we have their address user_address = get_user_address(update.effective_user.id)
# Get transaction details from user input
recipient = context.args[0]
amount = float(context.args[1])
# Connect to an Ethereum node (replace with your own node URL)
w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR-PROJECT-ID'))
# Create and sign the transaction (this is a simplified example)
transaction = {
'to': recipient,
'value': w3.toWei(amount, 'ether'),
'gas': 21000,
'gasPrice': w3.eth.gas_price,
'nonce': w3.eth.get_transaction_count(user_address),
}
# You would need to implement a secure way to manage private keys
signed_txn = w3.eth.account.sign_transaction(transaction, private_key=user_private_key)
# Send the transaction
tx_hash = w3.eth.send_raw_transaction(signed_txn.rawTransaction)
update.message.reply_text(f"Transaction sent! Hash: {tx_hash.hex()}")
```
Remember to add proper error handling and security measures when implementing transaction functionality in the Telegram Wallet Connector.
Created: | Last Updated:
Here's a step-by-step guide for using the Telegram Metamask Wallet Connector template:
Introduction
The Telegram Metamask Wallet Connector template allows you to create a Telegram bot that can connect users' MetaMask wallets via QR code. This bot can respond to messages, provide a menu, and facilitate wallet connections.
Getting Started
- Click "Start with this Template" to begin using the Telegram Wallet Connector template in the Lazy Builder interface.
Initial Setup
- Set up the required environment secret:
- In the Lazy Builder, go to the Environment Secrets tab.
- Add a new secret with the key
TELEGRAM_API_TOKEN
. - To get the value for this token:
- Open Telegram and search for the BotFather.
- Start a chat and use the
/newbot
command to create a new bot. - Follow the prompts to choose a name and username for your bot.
- BotFather will provide you with a token. Copy this token.
- Paste the token as the value for the
TELEGRAM_API_TOKEN
secret.
Test the App
- Click the "Test" button in the Lazy Builder to deploy and run your Telegram bot.
Using the App
-
Once deployed, your Telegram bot is ready to use. Here's how to interact with it:
-
Open Telegram and search for your bot using the username you created.
- Start a chat with the bot.
- Send the
/start
command to receive a welcome message and see the initial menu. - Use the
/connect_wallet
command to initiate the wallet connection process. - The bot will respond to any text messages by echoing them back.
Integrating the App
-
To fully integrate the wallet connection feature:
-
Replace the placeholder in the
generate_qr_code
function call with actual MetaMask wallet connection data. For example:
python
qr_code_stream = generate_qr_code("ethereum:0x123...789?value=0")
-
Implement the menu actions in the
button
function where the TODO comment is located. -
To customize the bot further:
-
Modify the
start
function to change the welcome message. - Add new command handlers in the
main
function to introduce more features.
By following these steps, you'll have a functional Telegram bot that can connect MetaMask wallets and respond to user interactions. Remember to test thoroughly and ensure all wallet connection data is handled securely.
Here are 5 key business benefits for the Telegram Wallet Connector template:
Template Benefits
-
Enhanced Customer Engagement: This bot provides an interactive platform for businesses to engage with customers directly through Telegram, a widely used messaging app. It offers a seamless way to communicate and provide services, potentially increasing customer satisfaction and loyalty.
-
Simplified Crypto Integration: By allowing users to connect their MetaMask wallets via QR code, the template facilitates easy integration of cryptocurrency functionality into business operations. This can open up new payment options and attract crypto-savvy customers.
-
Automated Customer Service: The bot can handle basic inquiries and commands automatically, reducing the workload on human customer service representatives and providing 24/7 availability for users.
-
Scalable Infrastructure: Built on the Telegram API, this template provides a scalable foundation that can handle a large number of users simultaneously, making it suitable for businesses of various sizes.
-
Customizable User Experience: The template includes a menu system that can be easily expanded to include additional features and services, allowing businesses to tailor the bot's functionality to their specific needs and offer a personalized experience to their users.
<@1082908341216485446> For more information about implementing and customizing this template for your business needs, please reach out.
Technologies
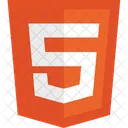
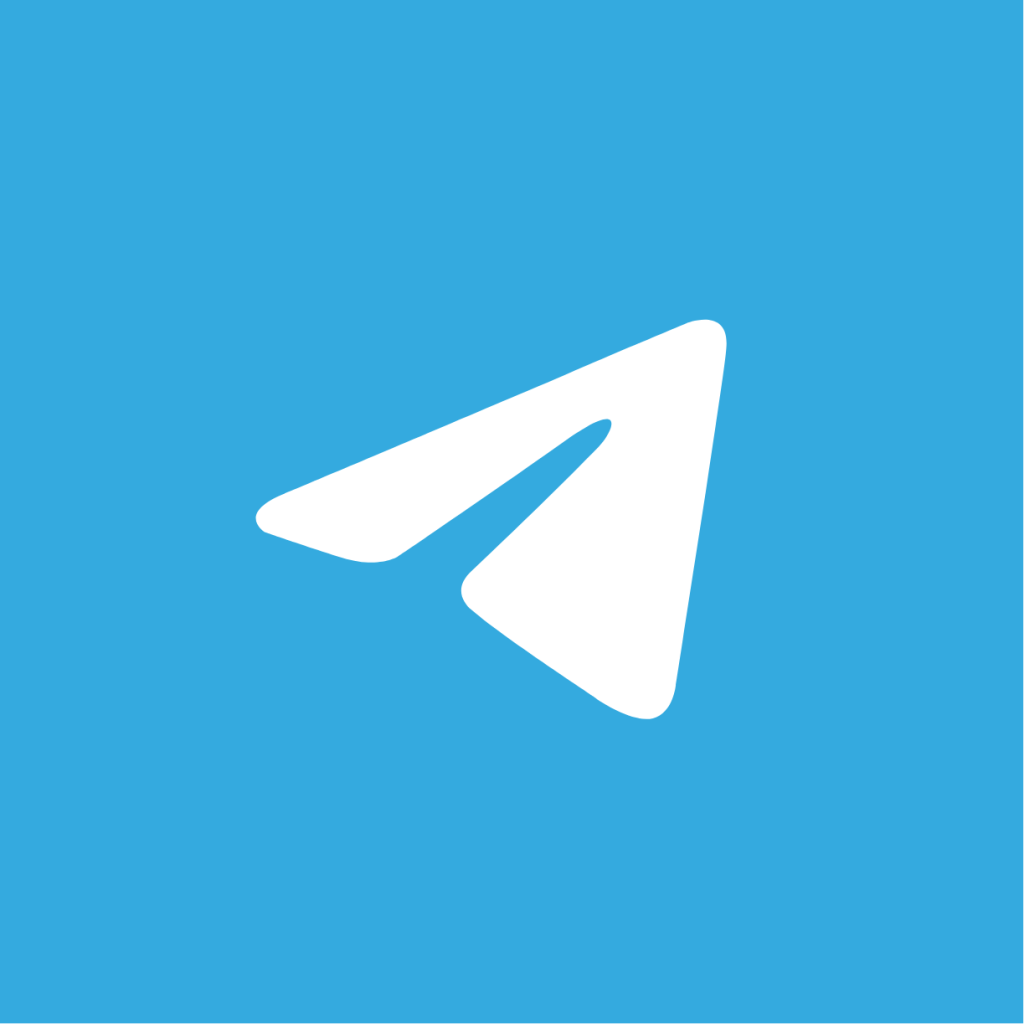
