by Lazy Sloth
Set Default Payment Method in Stripe API
import os
from flask import Flask, jsonify, request, render_template_string
# Set the Stripe API key from environment variables
stripe_api_key = os.environ.get('STRIPE_API_KEY')
if not stripe_api_key:
raise ValueError("The STRIPE_API_KEY environment variable is not set.")
import stripe
stripe.api_key = stripe_api_key
app = Flask(__name__)
# HTML form for setting the default payment method
HTML_FORM = '''
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Stripe Default Payment Method</title>
</head>
<body>
Frequently Asked Questions
What business problem does this Set Default Payment Method in Stripe API template solve?
This template addresses the common business need of allowing customers to set a default payment method for future transactions. By implementing this functionality, businesses can streamline the checkout process for returning customers, reduce cart abandonment rates, and improve overall customer experience. The Set Default Payment Method in Stripe API template provides a simple interface for customers to update their preferred payment method, which can lead to increased customer satisfaction and loyalty.
How can this template be customized for different business needs?
The Set Default Payment Method in Stripe API template can be easily customized to fit various business requirements. For example, you could: - Integrate it into an existing customer portal or account management system - Add additional fields for collecting billing information - Implement a feature to display and manage multiple saved payment methods - Customize the UI to match your brand's look and feel - Add error handling and validation specific to your business rules
What are the potential benefits of implementing this template for an e-commerce business?
Implementing the Set Default Payment Method in Stripe API template in an e-commerce business can lead to several benefits: - Faster checkout process for returning customers - Reduced cart abandonment rates due to payment friction - Improved customer data management - Enhanced ability to process recurring payments or subscriptions - Increased customer trust by using a secure, well-known payment processor (Stripe)
How can I add error handling for specific Stripe API errors in this template?
To add more specific error handling for Stripe API errors, you can modify the set_default_payment_method
function in the Flask app. Here's an example of how you could implement this:
```python from stripe.error import StripeError, CardError, InvalidRequestError
@app.route('/set_default_payment_method', methods=['POST']) def set_default_payment_method(): try: data = request.json if 'customer' not in data or 'payment_method' not in data: raise ValueError("Missing required fields in request.")
stripe.Customer.modify(
data['customer'],
invoice_settings={
'default_payment_method': data['payment_method']
}
)
return jsonify({"message": "Default payment method set successfully."}), 200
except CardError as e:
return jsonify(error="Invalid card details: " + str(e)), 400
except InvalidRequestError as e:
return jsonify(error="Invalid request: " + str(e)), 400
except StripeError as e:
return jsonify(error="Stripe error: " + str(e)), 500
except Exception as e:
return jsonify(error="Unexpected error: " + str(e)), 500
```
This modification adds specific handling for common Stripe errors, providing more detailed feedback to the user.
How can I extend this template to display a list of available payment methods for a customer?
To extend the Set Default Payment Method in Stripe API template to display available payment methods, you can add a new route and modify the HTML. Here's an example:
```python
@app.route('/get_payment_methods/
# Add this to your HTML_FORM string
'''
Available Payment Methods
''' ```
This modification adds a new endpoint to fetch payment methods and updates the HTML to display them when a customer ID is entered.
Created: | Last Updated:
Introduction to the Stripe Default Payment Method Template
Welcome to the Stripe Default Payment Method Template! This template is designed to help you integrate a simple web application that uses the Stripe API to set a default payment method for customers. It's built with Flask, a lightweight web framework for Python, and includes a user-friendly interface for inputting customer and payment method IDs. This guide will walk you through the steps to get this template up and running on the Lazy platform.
Getting Started
To begin using this template, click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before you can test and use the application, you need to set up an environment secret for the Stripe API key. Here's how to do it:
- Log in to your Stripe account and navigate to the API keys section.
- Copy your Stripe API key. If you don't have one, you'll need to create it.
- Go to the Environment Secrets tab within the Lazy Builder.
- Create a new secret with the key
STRIPE_API_KEY
and paste your Stripe API key as the value.
Test: Pressing the Test Button
Once you have set up your environment secret, press the "Test" button on the Lazy platform. This will deploy your application and launch the Lazy CLI.
Using the App
After pressing the "Test" button, Lazy will provide you with a dedicated server link to use the web interface. Navigate to this link in your web browser to access the application's frontend.
Here's how to use the interface:
- Enter the Customer ID for the Stripe customer whose default payment method you want to set.
- Enter the Payment Method ID that you want to set as the default for the customer.
- Click the "Set Default Payment Method" button to submit the form.
- The application will process your request and display a message indicating whether the default payment method was set successfully or if there was an error.
Integrating the App
If you want to integrate this functionality into another service or frontend, you can use the provided server link to make API calls to the /set_default_payment_method
endpoint. Here's a sample request you might make to the endpoint:
`POST /set_default_payment_method
Content-Type: application/json
{
"customer": "cus_xxxxxxxxxxxxx",
"payment_method": "pm_xxxxxxxxxxxxx"
}`
And here's a sample response you might receive:
{
"message": "Default payment method set successfully."
}
If you need to integrate this app into an external tool, you may need to add the app's server link provided by Lazy to that tool. Ensure you follow the specific integration steps required by the external tool, such as setting the correct scopes or adding the API endpoint in the tool's configuration.
By following these steps, you should now have a fully functional application that can set a default payment method for Stripe customers using the Lazy platform.
Here are 5 key business benefits for this template:
Template Benefits
-
Streamlined Payment Processing: By allowing customers to set a default payment method, businesses can automate recurring payments and reduce friction in the checkout process, leading to higher conversion rates and customer satisfaction.
-
Improved Cash Flow Management: With default payment methods in place, businesses can more reliably collect payments on time, reducing late payments and improving overall cash flow predictability.
-
Enhanced Customer Experience: Customers benefit from a smoother, more convenient payment process, as they don't need to re-enter payment information for each transaction, potentially increasing customer loyalty and retention.
-
Reduced Administrative Overhead: Automating the default payment method setup reduces the need for manual intervention in payment collection, saving time and resources for the business.
-
Flexibility and Scalability: This template can be easily integrated into existing systems and scaled to handle a growing customer base, making it suitable for businesses of various sizes and industries that rely on recurring payments or subscriptions.
Technologies
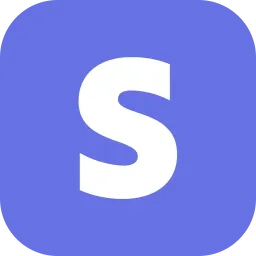