by Lazy Sloth
Create Account with Stripe API
import os
import stripe
import uvicorn
import logging
from fastapi import FastAPI, HTTPException, Request
from starlette.responses import HTMLResponse, RedirectResponse
from fastapi.middleware.cors import CORSMiddleware
from pydantic import BaseModel
app = FastAPI()
# CORS configuration
origins = ["*"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
# Set your secret key: remember to change this to your live secret key in production
# See your keys here: https://dashboard.stripe.com/account/apikeys
Frequently Asked Questions
What business problem does the Create Account with Stripe API template solve?
The Create Account with Stripe API template solves the challenge of seamlessly onboarding new sellers or service providers to your platform. It automates the process of creating Stripe accounts for your users, allowing them to start accepting payments quickly and easily. This is particularly useful for marketplaces, freelance platforms, or any business model where you need to facilitate payments to multiple parties.
How can this template benefit my e-commerce platform?
For e-commerce platforms, especially those with a multi-vendor model, the Create Account with Stripe API template can significantly streamline the seller onboarding process. It allows you to quickly set up new vendors with their own Stripe accounts, enabling them to receive payments directly. This can lead to faster marketplace growth, improved seller satisfaction, and reduced administrative overhead in managing payments.
Are there any industries or business models where this template is particularly useful?
The Create Account with Stripe API template is particularly valuable for: - Freelance marketplaces (e.g., for designers, writers, developers) - Sharing economy platforms (e.g., ride-sharing, home-sharing) - Multi-vendor e-commerce sites - Crowdfunding platforms - Online learning platforms where instructors receive payments These business models benefit from the ability to quickly onboard users who need to receive payments, making the platform more attractive and easier to use.
How do I customize the return URL in the Create Account with Stripe API template?
To customize the return URL in the Create Account with Stripe API template, you need to modify the frontend JavaScript code. Specifically, replace the RETURN_URL
placeholder with your desired URL. Here's an example:
javascript
const returnUrl = "https://yourdomain.com/onboarding-complete";
This URL is where users will be redirected after they complete the Stripe account creation process. Make sure this page is set up to handle the return and provide appropriate next steps or confirmation to the user.
Can I add additional parameters to the Stripe account creation process using this template?
Yes, you can add additional parameters to the Stripe account creation process by modifying the stripe.Account.create()
call in the backend code. For example, if you want to prefill some information or set specific account details, you can add parameters like this:
python
account_id = stripe.Account.create(
type="express",
country="US",
email="user@example.com",
business_type="individual",
capabilities={
"card_payments": {"requested": True},
"transfers": {"requested": True},
},
).id
This example creates an account for a US-based individual with card payment and transfer capabilities. Remember to collect and validate any additional information from the user before passing it to the API.
Created: | Last Updated:
Introduction to the Create Account with Stripe API Template
Welcome to the Create Account with Stripe API Template! This template is designed to help you seamlessly integrate Stripe account creation into your application. With this template, you can automatically redirect users to Stripe's hosted account creation page, where they can provide their information. Once completed, users will be redirected back to your specified return URL. This process is made possible through the Stripe Connect platform, and it requires that you have your Stripe secret key ready.
Clicking Start with this Template
To begin using this template, simply click on the "Start with this Template" button. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before you can test and use the app, you'll need to set up an environment secret within the Lazy Builder. Specifically, you need to provide your Stripe secret key. Here's how to do it:
- Log in to your Stripe dashboard and navigate to the API keys section.
- Copy your secret key. Remember to use a test key for development and switch to a live key in production.
- In the Lazy Builder, go to the Environment Secrets tab.
- Create a new secret with the key
STRIPE_SECRET_KEY
and paste your Stripe secret key as the value.
With the secret key in place, your app will be able to communicate securely with Stripe's API.
Test: Pressing the Test Button
Once you've set up your environment secret, press the "Test" button. This will deploy your app using the Lazy CLI. If the app requires any user input, you will be prompted to provide it through the Lazy CLI interface.
Entering Input: Filling in User Input
If the template code requires user input, you will be prompted for it after pressing the "Test" button. Follow the instructions in the CLI to enter the necessary information.
Using the App
After deployment, Lazy will provide you with a dedicated server link to use the API. If you're using FastAPI, you will also receive a link to the API documentation. Use these links to interact with your app and test the Stripe account creation process.
Integrating the App
To integrate this app into your service or frontend, you will need to make a POST request to the backend endpoint provided by Lazy. Here's a sample JavaScript code snippet that you can use in your frontend application:
`Remember to replace
YOUR_LAZY_BACKEND_URLwith the URL provided by Lazy after deployment, and
YOUR_RETURN_URL` with the URL where you want users to be redirected after completing the Stripe account setup.
By following these steps, you should now have a fully functional integration with Stripe for account creation in your application. If you encounter any issues or have further questions, refer to the Stripe API documentation provided in the code or reach out for support.
Here are 5 key business benefits for this template:
Template Benefits
-
Streamlined Onboarding: Enables businesses to quickly and easily onboard new merchants or service providers by automating the Stripe account creation process.
-
Improved User Experience: Provides a seamless flow for users to create their Stripe accounts without leaving the main application, reducing friction and potential drop-offs.
-
Flexibility and Customization: Allows businesses to specify custom return URLs, making it adaptable to various business workflows and user journeys.
-
Scalability: Facilitates rapid expansion of a platform's user base by simplifying the account creation process for multiple vendors or partners.
-
Compliance and Security: Leverages Stripe's secure, compliant infrastructure for handling sensitive financial information, reducing the burden on businesses to manage this data directly.
Technologies
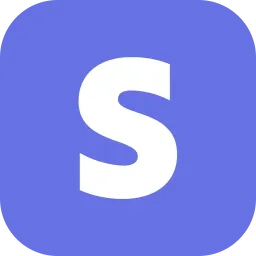