by 4nimal.yt
stress3 bot design
from models import TournamentStage
from models import Participant
from abilities import llm
import os
import discord
from discord import app_commands
from abilities import apply_sqlite_migrations
import asyncio
from flask import Flask, render_template, request, flash, redirect, url_for
from models import Base, engine
from sqlalchemy.orm import Session
from sqlalchemy import select
app = Flask(__name__)
app.secret_key = os.urandom(24) # For flash messages
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
intents = discord.Intents.default()
Frequently Asked Questions
How can the Culling Games Discord bot enhance engagement for online gaming communities?
The Culling Games Discord bot can significantly boost engagement in online gaming communities by providing an immersive, interactive experience. It simulates a tournament-style game with different stages, participant rankings, and dynamic responses. This creates a unique, ongoing narrative that keeps players invested and encourages regular interaction. The bot's ability to recognize participants and provide context-aware responses adds depth to the gaming experience, making it more compelling for community members.
What are some potential business applications for a bot like the Culling Games system outside of gaming?
While the Culling Games bot is designed for a gaming context, its core functionality can be adapted for various business applications:
- Employee Training: Companies could use a similar system for gamified training programs, where employees progress through "stages" of learning.
- Customer Loyalty Programs: Retail businesses could implement a tiered reward system with challenges and advancements similar to the Culling Games stages.
- Project Management: Teams could use a modified version to track project milestones and team member contributions in a more engaging way.
The bot's ability to track progress, provide personalized responses, and manage different stages makes it versatile for many business scenarios that benefit from gamification.
How could the Culling Games bot be monetized in a gaming community?
The Culling Games bot offers several monetization opportunities:
- Premium Features: Offer advanced stats, exclusive tournament entries, or special "cursed tools" for a subscription fee.
- Sponsored Tournaments: Partner with gaming companies to host branded tournaments using the bot.
- In-Game Purchases: Allow participants to buy virtual items or power-ups that give them advantages in the game.
- Data Insights: Anonymized data from the game could be valuable for gaming companies looking to understand player behavior and preferences.
By leveraging the engagement the bot creates, there are multiple avenues to generate revenue while enhancing the player experience.
How can I modify the Culling Games bot to add a new command for players to check their current status?
To add a new command for players to check their status, you can modify the main.py
file. Here's an example of how to implement this:
```python @tree.command(name="status", description="Check your current status in the Culling Games") async def status(interaction: discord.Interaction): with Session(engine) as session: participant = session.execute( select(Participant).where(Participant.name == interaction.user.name) ).scalar_one_or_none()
if participant:
status_message = f"Name: {participant.name}\nGrade: {participant.grade}\nTool Grade: {participant.tool_grade}\nStatus: {'Active' if participant.active else 'Eliminated'}"
else:
status_message = "You are not currently registered in the Culling Games."
await interaction.response.send_message(status_message, ephemeral=True)
```
This code adds a new /status
command that players can use to check their current status in the game. It retrieves the player's information from the database and sends it as a private message.
How can I extend the Culling Games bot to support multiple servers with different configurations?
To support multiple servers with different configurations, you'll need to modify the database schema and the bot's logic. Here's a high-level approach:
Created: | Last Updated:
Here's a step-by-step guide for using the Culling Games Discord Bot template:
Introduction
This template provides a Discord bot for hosting and managing the Culling Games, a tournament-style event inspired by the Jujutsu Kaisen universe. The bot allows administrators to send messages as the bot, manages participant information, and responds to user mentions with context-aware responses.
Getting Started
-
Click "Start with this Template" to begin using this template in the Lazy Builder interface.
-
Set up the required environment secrets:
- In the Lazy Builder, navigate to the Environment Secrets tab.
- Add the following secrets:
CLIENT_ID
: Your Discord application's client IDBOT_TOKEN
: Your Discord bot token
To obtain these values:
- Go to the Discord Developer Portal
- Create a new application or select an existing one
- Navigate to the "Bot" section and click "Add Bot"
- Under the "TOKEN" section, click "Copy" to get your BOT_TOKEN
- Go to the "OAuth2" section and copy the "CLIENT ID"
- In the Discord Developer Portal, under the "Bot" section, enable the "Message Content Intent" slider.
Testing the Bot
-
Click the "Test" button in the Lazy Builder interface to start the deployment process.
-
Once deployment is complete, you'll see a Discord bot invitation link in the Lazy CLI. Use this link to invite the bot to your Discord server.
Using the Bot
-
The bot will now be active in your Discord server. Here are the main features:
-
Administrators can use the
/ttm
command to send messages as the bot. -
When users mention the bot, it will respond with context-aware messages based on the current tournament stage and the user's participant information.
-
To add participants to the Culling Games:
- Access the web interface provided by the Lazy CLI after deployment.
- Use the form to add new participants, specifying their name, grade, and tool grade.
Integrating the Bot
- To fully integrate the bot into your Discord server:
- Ensure the bot has the necessary permissions to read and send messages in the desired channels.
-
Consider creating a dedicated channel for Culling Games announcements and interactions.
-
Customize the tournament stages and rules:
- The initial tournament stages are pre-populated in the database.
- To modify these, you'll need to update the SQL migration files or use a database management tool to alter the
tournament_stages
table.
By following these steps, you'll have a functional Culling Games Discord bot that can manage participants, respond to queries, and facilitate the tournament process.
Here are 5 key business benefits for this template:
Template Benefits
-
Automated Tournament Management - The system automates many aspects of running a complex tournament, including participant registration, stage tracking, and rule enforcement. This reduces manual work and potential errors.
-
Engaging User Experience - The Discord bot provides an interactive and immersive experience for participants, allowing them to engage with the tournament system directly through chat. This increases user engagement and retention.
-
Flexible Tournament Structure - The template supports a multi-stage tournament with different rules and advancement criteria for each stage. This flexibility allows for creating diverse and exciting competition formats.
-
Data-Driven Decision Making - By storing participant and tournament data in a database, organizers can easily track progress, analyze performance, and make informed decisions about tournament management.
-
Scalable Architecture - The combination of a Flask web application and Discord bot allows for easy scaling of the system. Additional features or integrations can be added to either component as the tournament grows in complexity or size.
Technologies
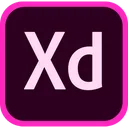
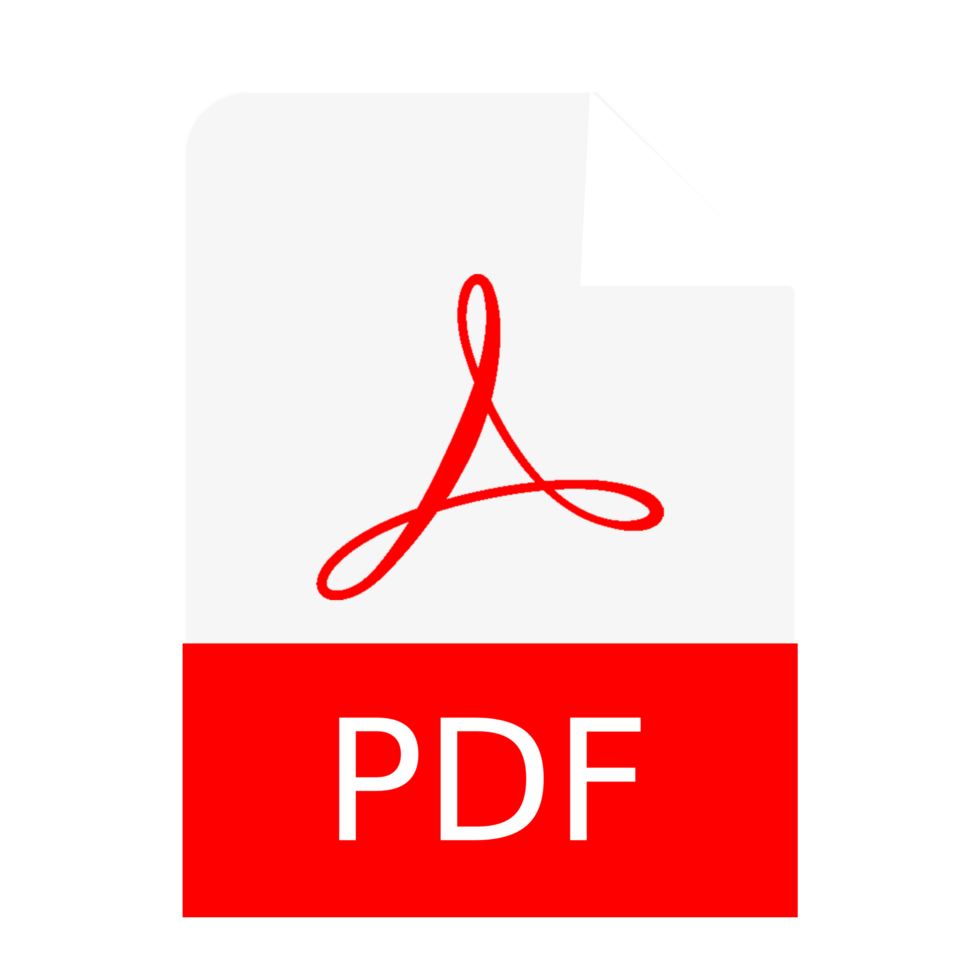
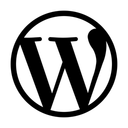
