by omaralt1012
Responsive Login Page
import logging
from flask import Flask
from gunicorn.app.base import BaseApplication
from routes import register_routes
from models import db
from migrations.run_migrations import run_migrations
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
run_migrations(app)
register_routes(app)
return app
app = create_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
Frequently Asked Questions
How can this Responsive Login Page template benefit my business?
The Responsive Login Page template offers several benefits for your business: - It provides a professional and user-friendly interface for customer authentication. - The responsive design ensures a seamless experience across various devices, potentially increasing user engagement and retention. - The template's clean layout and intuitive design can help reduce user frustration and improve conversion rates for sign-ups or logins.
Can this template be customized to match my brand's identity?
Absolutely! The Responsive Login Page template is highly customizable. You can easily modify the colors, fonts, and layout to align with your brand's identity. For example, you can update the CSS variables in the styles.css
file to change the primary colors:
css
:root {
--primary-color: #YourBrandColor;
--secondary-color: #YourSecondaryColor;
}
This flexibility allows you to create a cohesive look and feel across your entire web application.
What industries or types of applications would benefit most from this login page template?
The Responsive Login Page template is versatile and can be beneficial for various industries and applications, including: - E-commerce platforms requiring user accounts for purchases and order tracking - SaaS (Software as a Service) applications with subscription-based access - Educational platforms or learning management systems - Healthcare portals for patient access to medical records - Financial services applications requiring secure user authentication
How does the template handle form validation?
The Responsive Login Page template includes basic client-side validation to ensure that both username and email fields are filled before submission. This is implemented in the routes.py
file:
```python @app.route("/login", methods=["GET", "POST"]) def login(): if request.method == "POST": username = request.form.get("username") email = request.form.get("email")
if not username or not email:
flash("Please fill in both username and email fields.", "danger")
else:
# Mock authentication process
flash("Login successful!", "success")
return redirect(url_for("home_route"))
```
You can easily extend this validation to include more complex checks, such as email format validation or password strength requirements.
Is the Responsive Login Page template secure for handling user authentication?
While the template provides a solid foundation for user authentication, it's important to note that the current implementation uses a mock authentication process. For a production environment, you should implement proper security measures, including:
- Secure password hashing (the template includes a function for this in database_operations.py
)
- HTTPS encryption for all communications
- Protection against common vulnerabilities like SQL injection and cross-site scripting (XSS)
- Implementation of features like rate limiting and multi-factor authentication
Always consult with security experts and follow best practices when implementing authentication systems for production use.
Created: | Last Updated:
Here's a step-by-step guide for using the Responsive Login Page template:
Introduction
This template provides a responsive login page with a user-friendly design, input fields for username and email, basic validation, and mock authentication. It's built using Flask, SQLAlchemy, and Tailwind CSS, offering a solid foundation for a login system that you can easily customize and expand upon.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- The application will be deployed, and you'll see the Lazy CLI appear.
Using the Application
After the deployment is complete:
- Lazy will provide you with a dedicated server link to access your login page.
- Open this link in your web browser to view and interact with the login page.
The login page offers the following features:
- A responsive design that adapts to different screen sizes
- Input fields for username and email
- Basic client-side validation
- A mock authentication process
To test the login functionality:
- Enter any username and email address in the respective fields.
- Click the "Sign in" button.
- You'll see a "Login successful!" message, demonstrating the mock authentication process.
Customizing the Application
To customize this template for your specific needs:
- Modify the HTML templates in the
home.html
,login.html
, andregister.html
files to adjust the layout and content. - Update the CSS in
styles.css
to change the appearance of the login page. - Enhance the authentication logic in
routes.py
to implement real user authentication instead of the mock process. - Extend the database schema in
models.py
anddatabase_operations.py
to store and manage user data as needed.
Next Steps
To turn this into a fully functional login system:
- Implement proper user authentication and password hashing.
- Add user registration functionality.
- Create protected routes that require user authentication.
- Implement password reset functionality.
- Consider adding additional security measures like rate limiting and CSRF protection.
Remember, all development and customization can be done directly within the Lazy Builder interface. There's no need for local setup or environment configuration.
Here are 5 key business benefits for this responsive login page template:
Template Benefits
-
Enhanced User Experience: The responsive design ensures a seamless login experience across desktop and mobile devices, improving user satisfaction and engagement.
-
Increased Security: By implementing user authentication and password hashing, the template provides a basic level of security to protect user accounts and sensitive information.
-
Scalability: The modular structure and use of Flask framework allow for easy expansion of features and integration with other systems as the application grows.
-
Rapid Development: Pre-built components and styling with Tailwind CSS enable quick customization and deployment, reducing development time and costs.
-
Maintainability: The organized file structure, separation of concerns, and use of templates make the codebase easier to maintain and update over time, lowering long-term development costs.
Technologies
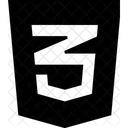
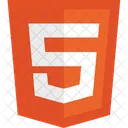
