RealTime Code IDE
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the RealTime Code IDE benefit businesses in the software development industry?
The RealTime Code IDE offers significant advantages for businesses in software development. It provides a collaborative platform where developers can write, edit, and preview code in real-time across multiple languages. This can greatly enhance team productivity, especially for remote teams. The ability to quickly switch between languages like HTML, CSS, JavaScript, and TypeScript makes it ideal for full-stack development projects. Additionally, the instant preview feature allows for rapid prototyping and iterative development, potentially reducing development cycles and time-to-market for web applications.
Can the RealTime Code IDE be integrated into existing development workflows or training programs?
Absolutely! The RealTime Code IDE is versatile enough to be integrated into various development workflows and training programs. For businesses, it can serve as a powerful tool for code reviews, pair programming sessions, or even technical interviews. In training scenarios, it's an excellent platform for interactive coding exercises and demonstrations. The multi-language support in RealTime Code IDE makes it particularly useful for full-stack development courses or for companies that work across different technology stacks.
What are the potential cost savings associated with implementing the RealTime Code IDE in a business environment?
Implementing the RealTime Code IDE can lead to significant cost savings for businesses. By providing a comprehensive development environment in the browser, it reduces the need for setting up and maintaining individual development environments on each developer's machine. This can cut down on IT infrastructure costs and setup time. Moreover, its real-time collaboration features can potentially reduce the need for additional collaboration tools. The quick preview functionality can also lead to faster debugging and reduced QA cycles, ultimately saving time and resources in the development process.
How does the RealTime Code IDE handle TypeScript compilation for preview?
The RealTime Code IDE uses Monaco Editor's built-in TypeScript worker to compile TypeScript code on the fly. Here's a simplified example of how it works:
```javascript async function compileTypeScript() { const worker = await monaco.languages.typescript.getTypeScriptWorker(); const client = await worker(editors['typescript'].getModel().uri); const emitOutput = await client.getEmitOutput(editors['typescript'].getModel().uri.toString());
if (emitOutput.outputFiles.length > 0) {
const compiledCode = emitOutput.outputFiles[0].text;
// Use compiledCode to update the preview
}
} ```
This process allows for real-time compilation and preview of TypeScript code, enhancing the development experience in the RealTime Code IDE.
Can you explain how the RealTime Code IDE manages different language editors and previews?
The RealTime Code IDE uses a tab-based system to manage different language editors. Each language has its own Monaco Editor instance, and the active language is controlled by a switchLanguage
function. Here's a simplified version of how this is implemented:
```javascript let editors = {}; let currentLanguage = 'html';
function switchLanguage(language) { // Update tabs document.querySelectorAll('.editor-tab').forEach(tab => { tab.classList.toggle('active', tab.textContent === language.toUpperCase()); });
// Update editors
Object.keys(editors).forEach(lang => {
document.getElementById(`editor-${lang}`).classList.toggle('hidden', lang !== language);
});
currentLanguage = language;
updatePreview();
} ```
This setup allows users to seamlessly switch between different language editors while maintaining a consistent preview based on the active language, making the RealTime Code IDE a versatile tool for multi-language development.
Created: | Last Updated:
Here's a step-by-step guide for using the RealTime Code IDE template:
Introduction
The RealTime Code IDE template provides a web-based Integrated Development Environment (IDE) for real-time code editing and previewing in multiple languages. It supports HTML, CSS, JavaScript, TypeScript, Python, and PHP, with a split-screen layout for easy visualization of your code and its output.
Getting Started
- Click "Start with this Template" to begin using the RealTime Code IDE template in your Lazy Builder interface.
Test the Application
- Press the "Test" button to deploy the application and launch the Lazy CLI.
Using the RealTime Code IDE
-
Once the application is deployed, you'll receive a dedicated server link to access the web-based IDE.
-
Open the provided link in your web browser to start using the RealTime Code IDE.
-
The IDE interface consists of two main sections:
- Left panel: Code editor
-
Right panel: Preview window
-
Use the tabs at the top of the editor panel to switch between different programming languages:
- HTML
- CSS
- JavaScript
- TypeScript
- Python
-
PHP
-
Start coding in your chosen language. The editor provides features like syntax highlighting, auto-completion, and error checking.
-
For HTML, CSS, and JavaScript:
-
As you type, the preview window on the right will automatically update to show the result of your code.
-
For TypeScript:
-
The preview window will display the compiled JavaScript output and any React components you've created.
-
For Python and PHP:
- These server-side languages cannot be previewed directly in the browser. The preview window will display a message indicating that preview is not available for these languages.
-
Use the mobile toolbar (available on mobile devices) for additional functionality:
- "Select All" button: Quickly select all the code in the current editor.
- "Paste" button: Paste content from your device's clipboard into the editor.
Additional Features
- The editor uses Monaco Editor, providing a rich coding experience similar to Visual Studio Code.
- TypeScript code is automatically compiled to JavaScript for preview.
- The preview for TypeScript includes React support, allowing you to create and test React components in real-time.
- The IDE includes support for various UI libraries and frameworks, including Tailwind CSS, DaisyUI, and Chakra UI.
By following these steps, you can effectively use the RealTime Code IDE template to write, edit, and preview code in multiple languages within a single, convenient web-based interface.
Here are 5 key business benefits for this RealTime Code IDE template:
Template Benefits
-
Rapid Prototyping and Development: Enables developers to quickly prototype and test code ideas across multiple languages in a single environment, accelerating the development process.
-
Enhanced Collaboration: Facilitates real-time code sharing and collaboration among team members, allowing for instant feedback and pair programming scenarios.
-
Cross-Platform Compatibility: As a web-based solution, it provides a consistent development environment across different operating systems and devices, reducing setup and compatibility issues.
-
Integrated Learning Tool: Serves as an excellent platform for coding education and training, allowing instructors to demonstrate concepts in real-time with immediate visual feedback.
-
Streamlined Code Review Process: Offers a convenient way to review and discuss code changes, with the ability to instantly see the impact of modifications, leading to more efficient code reviews and quality assurance.
Technologies
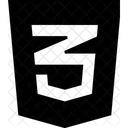
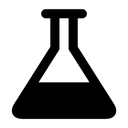
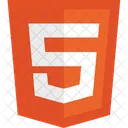
