by moonboy
PDF to ePub Converter
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
import sys
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO, stream=sys.stdout,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
Frequently Asked Questions
What are some potential business applications for the PDF to ePub Converter?
The PDF to ePub Converter has several business applications: - Publishing houses can use it to convert their PDF catalogs to ePub format for e-readers. - Educational institutions can convert PDF textbooks to ePub for better accessibility on various devices. - Corporate training departments can transform PDF manuals into ePub format for easier distribution and reading on mobile devices. - Authors and self-publishers can convert their PDF manuscripts to ePub for submission to online bookstores.
How can the PDF to ePub Converter improve user experience for readers?
The PDF to ePub Converter enhances reader experience in several ways: - ePub files are reflowable, allowing text to adjust to different screen sizes and orientations. - Readers can customize font size, style, and background color in ePub files, which is not possible with PDFs. - ePub format supports better navigation features like table of contents and bookmarking. - The converter makes content more accessible on e-readers and mobile devices, which are optimized for ePub format.
What are the potential monetization strategies for the PDF to ePub Converter?
There are several ways to monetize the PDF to ePub Converter: - Offer a freemium model with basic conversions free and advanced features (like bulk conversion or OCR) as paid options. - Implement a subscription-based service for businesses that need regular conversions. - Charge per conversion, with pricing tiers based on file size or complexity. - Partner with e-publishing platforms or e-reader manufacturers for integrated conversion services.
How does the PDF to ePub Converter handle file uploads, especially for large files?
The PDF to ePub Converter uses a chunk-based upload system to handle large files efficiently. Here's a key part of the implementation from the home.js
file:
```javascript function uploadChunk(chunkIndex) { if (chunkIndex >= chunks) { return; }
const start = chunkIndex * chunkSize;
const end = Math.min(start + chunkSize, file.size);
const chunk = file.slice(start, end);
const formData = new FormData();
formData.append('file', chunk, file.name);
formData.append('chunk', chunkIndex);
formData.append('chunks', chunks);
// ... XHR request to upload chunk ...
} ```
This code splits the file into chunks and uploads them separately, allowing for better handling of large files and providing a progress indicator for users.
Can you explain how the PDF to ePub Converter performs the actual conversion process?
The PDF to ePub Converter uses the ebooklib
and PyPDF2
libraries to perform the conversion. Here's a simplified version of the conversion process from the pdf2epub.py
file:
```python def convert_pdf_to_epub(pdf_path, epub_path): book = epub.EpubBook() reader = PdfReader(pdf_path)
for i, page in enumerate(reader.pages):
content = page.extract_text()
c = epub.EpubHtml(title=f'Page {i+1}', file_name=f'page_{i+1}.xhtml', lang='en')
c.content = f'<h1>Page {i+1}</h1><p>{escape(content)}</p>'
book.add_item(c)
# ... Add TOC, NCX, Nav, and CSS ...
epub.write_epub(epub_path, book, {})
```
This function reads each page of the PDF, extracts the text, creates an EPUB chapter for each page, and then compiles these chapters into a complete EPUB file. The PDF to ePub Converter wraps this functionality in a web interface for easy use.
Created: | Last Updated:
Here's a step-by-step guide for using the PDF to ePub Converter template:
Introduction
This template provides a web application for converting PDF files to ePub format. It features a user-friendly upload interface and handles the conversion process server-side.
Getting Started
- Click "Start with this Template" to begin using the PDF to ePub Converter template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the PDF to ePub Converter
-
Once the application is deployed, you'll receive a dedicated server link to access the web interface.
-
Open the provided link in your web browser to access the PDF to ePub Converter interface.
-
Use the file upload form to select and upload a PDF file you want to convert.
-
After uploading, click the "Convert to ePub" button to start the conversion process.
-
Once the conversion is complete, the application will automatically download the converted ePub file to your device.
Integration (Optional)
This application is designed to be used as a standalone web service. There are no additional integration steps required for its basic functionality. However, if you want to incorporate this conversion capability into another application or service, you can do so by making HTTP requests to the server.
Here's a sample code snippet for integrating the PDF to ePub conversion into another application using Python and the requests
library:
```python import requests
def convert_pdf_to_epub(pdf_file_path, server_url): # Upload the PDF file with open(pdf_file_path, 'rb') as pdf_file: files = {'pdf_file': pdf_file} response = requests.post(f"{server_url}/upload", files=files)
if response.status_code == 200:
# Trigger the conversion
response = requests.post(f"{server_url}/convert")
if response.status_code == 200:
# Save the converted ePub file
with open('converted.epub', 'wb') as epub_file:
epub_file.write(response.content)
print("Conversion successful. ePub file saved as 'converted.epub'")
else:
print("Conversion failed")
else:
print("File upload failed")
Usage example: server_url = "YOUR_SERVER_URL_HERE" pdf_file_path = "path/to/your/pdf/file.pdf" convert_pdf_to_epub(pdf_file_path, server_url) ```
Replace "YOUR_SERVER_URL_HERE"
with the actual server URL provided by the Lazy platform when you deploy the application.
This integration allows you to programmatically convert PDF files to ePub format using the deployed PDF to ePub Converter service.
Here are 5 key business benefits for this PDF to ePub Converter template:
Template Benefits
-
Enhanced Content Accessibility: Converts PDF documents to the more flexible ePub format, making content easily readable on various devices and e-readers. This improves accessibility and reach of digital publications.
-
Improved User Experience: Provides a simple, intuitive web interface for users to upload PDFs and receive converted ePub files. The progress tracking and responsive design ensure a smooth experience across devices.
-
Scalable Architecture: Utilizes a modular Flask structure with Gunicorn, allowing for easy scaling to handle increased conversion demands. The chunked file upload system supports large PDF files efficiently.
-
Cost-Effective Document Management: Offers businesses a tool to convert existing PDF libraries to ePub format without the need for expensive proprietary software, potentially reducing licensing costs.
-
Integration Potential: The template's structure allows for easy integration into existing document management systems or e-commerce platforms, enabling businesses to offer PDF-to-ePub conversion as a value-added service.
Technologies
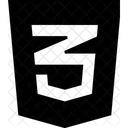
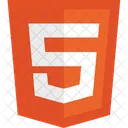
