AI-Based PDF Chatbot
import logging
from flask import Flask, render_template, session, flash, request, jsonify, send_from_directory, redirect
from flask_session import Session
from gunicorn.app.base import BaseApplication
from werkzeug.utils import secure_filename
import os
from abilities import llm_prompt
from pdf_manager import PDFManager
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__)
# Configuring server-side session
app.config["SESSION_PERMANENT"] = False
app.config["SESSION_TYPE"] = "filesystem"
Session(app)
app.config['UPLOAD_FOLDER'] = 'uploads'
app.config['ALLOWED_EXTENSIONS'] = {'pdf'}
def allowed_file(filename):
return '.' in filename and filename.rsplit('.', 1)[1].lower() in app.config['ALLOWED_EXTENSIONS']
Frequently Asked Questions
How can businesses benefit from using the PDF Chatbot app?
The PDF Chatbot app offers several advantages for businesses: - Efficient document analysis: Quickly extract information from large PDF documents. - Improved customer service: Provide instant answers to customer queries about product manuals or reports. - Time-saving: Reduce the time employees spend searching through lengthy documents. - Knowledge management: Make organizational knowledge more accessible and actionable. - Training support: Use the chatbot to help new employees understand company documentation.
Can the PDF Chatbot be customized for specific industries?
Yes, the PDF Chatbot can be tailored for various industries. For example: - Legal: Analyze contracts and legal documents. - Healthcare: Interpret medical records and research papers. - Finance: Extract data from financial reports and regulatory filings. - Education: Assist students in understanding textbooks and research materials. - Technical: Help engineers quickly find information in technical specifications.
Customization would involve training the AI model on industry-specific terminology and adjusting the prompt engineering in the llm_prompt
function to better handle domain-specific queries.
What are the security considerations when using the PDF Chatbot for sensitive documents?
When using the PDF Chatbot for sensitive information, consider: - Data encryption: Implement encryption for uploaded files and stored data. - Access control: Add user authentication and authorization to restrict access. - Compliance: Ensure the app meets relevant data protection regulations (e.g., GDPR, HIPAA). - Secure hosting: Use a secure, compliant cloud provider or on-premises hosting. - Data retention: Implement policies to delete uploaded files after a set period. - Audit trails: Add logging to track file uploads and user interactions.
How can I modify the PDF Chatbot to support multiple file uploads?
To support multiple file uploads, you'll need to make changes to both the frontend and backend. Here's a basic example of how to modify the code:
In template.html
, update the file input to allow multiple selections:
html
<input type="file" id="pdfFile" name="file" accept=".pdf" multiple class="block w-full text-sm text-gray-500
file:mr-4 file:py-2 file:px-4
file:rounded-full file:border-0
file:text-sm file:font-semibold
file:bg-violet-50 file:text-violet-700
hover:file:bg-violet-100"/>
In main.py
, modify the upload_file
function to handle multiple files:
python
@app.route("/", methods=['GET', 'POST'])
def upload_file():
if request.method == 'POST':
uploaded_files = []
for file in request.files.getlist('file'):
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
file.save(file_path)
uploaded_files.append(file_path)
session['file_paths'] = uploaded_files
flash(f'{len(uploaded_files)} files uploaded successfully!')
return redirect('/')
uploaded_files = session.get('file_paths', [])
return render_template("template.html", uploaded_files=[os.path.basename(f) for f in uploaded_files])
You'll also need to update the send_message
function to handle multiple files when processing queries.
How can I integrate a different language model into the PDF Chatbot?
To integrate a different language model, you'll need to modify the llm_prompt
function in the abilities.py
file. Here's an example of how you might integrate the OpenAI GPT-3.5 model:
```python import openai
def llm_prompt(prompt, model="gpt-3.5-turbo", temperature=0.7): openai.api_key = "your-api-key-here"
response = openai.ChatCompletion.create(
model=model,
messages=[
{"role": "system", "content": "You are a helpful assistant that answers questions about PDF documents."},
{"role": "user", "content": prompt}
],
temperature=temperature
)
return response.choices[0].message['content']
```
Remember to add openai
to your requirements.txt
file and set up proper error handling and API key management. When integrating a new model, ensure it can handle the context length of your PDF documents and adjust the prompt engineering as needed to get optimal results with the PDF Chatbot.
Created: | Last Updated:
Introduction to the PDF Chatbot Template
The PDF Chatbot template is a powerful tool for creating an application that can answer questions about the content of uploaded PDF documents. This application uses a chatbot interface to interact with users and provide responses based on the text extracted from the PDFs. It's an excellent solution for customer support, research, or any scenario where quick access to information within PDF documents is needed.
Getting Started with the Template
To begin building your PDF Chatbot application, click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy or paste any code manually.
Initial Setup
Before testing the application, ensure that you have all the necessary files and configurations in place. This template does not require setting up environment secrets, so you can proceed to the next step.
Test: Deploying the App
To deploy your PDF Chatbot app, press the "Test" button on the Lazy platform. This will initiate the deployment process and launch the Lazy CLI. The application will be executed on the Lazy platform, and you won't need to worry about installing libraries or setting up your environment.
Entering Input
If the application requires user input, the Lazy App's CLI interface will prompt you to provide it after pressing the "Test" button. Follow the prompts in the CLI to input the necessary information for your chatbot to function correctly.
Using the App
Once the app is deployed, Lazy will provide a dedicated server link to use the chatbot interface. If your application includes a frontend, you can interact with the chatbot by typing messages and receiving responses directly through the provided interface.
Integrating the App
If you need to integrate the PDF Chatbot app into an external service or frontend, you can use the server link provided by Lazy after deployment. For example, you might want to embed the chatbot in a website or connect it to an external database. Follow the specific integration steps required by the external tool to complete the setup.
Remember, this template is designed to simplify the process of creating a chatbot that can interact with PDF content. With Lazy handling the deployment and server management, you can focus on customizing the chatbot to meet your specific needs.
For further assistance or to explore more capabilities of the Lazy platform, refer to the official documentation or reach out to the Lazy support team.
Here are 5 key business benefits for the PDF Chatbot template:
Template Benefits
-
Enhanced Document Analysis: Enables quick extraction and analysis of information from PDF documents, saving time and improving efficiency for businesses dealing with large volumes of PDF content.
-
Improved Customer Support: Can be used to create an AI-powered assistant that answers customer queries about product manuals, terms of service, or other PDF-based documentation, reducing the workload on human support staff.
-
Streamlined Research: Researchers and analysts can quickly query multiple PDF sources, synthesizing information and uncovering insights faster than manual reading and searching.
-
Compliance and Legal Aid: Helps legal professionals or compliance officers quickly find relevant information in lengthy legal documents, contracts, or regulatory PDFs, ensuring thorough review and reducing oversight risks.
-
Interactive Training and Education: Can be adapted for educational purposes, allowing students or employees to ask questions about training materials or textbooks in PDF format, creating a more engaging and personalized learning experience.
Technologies
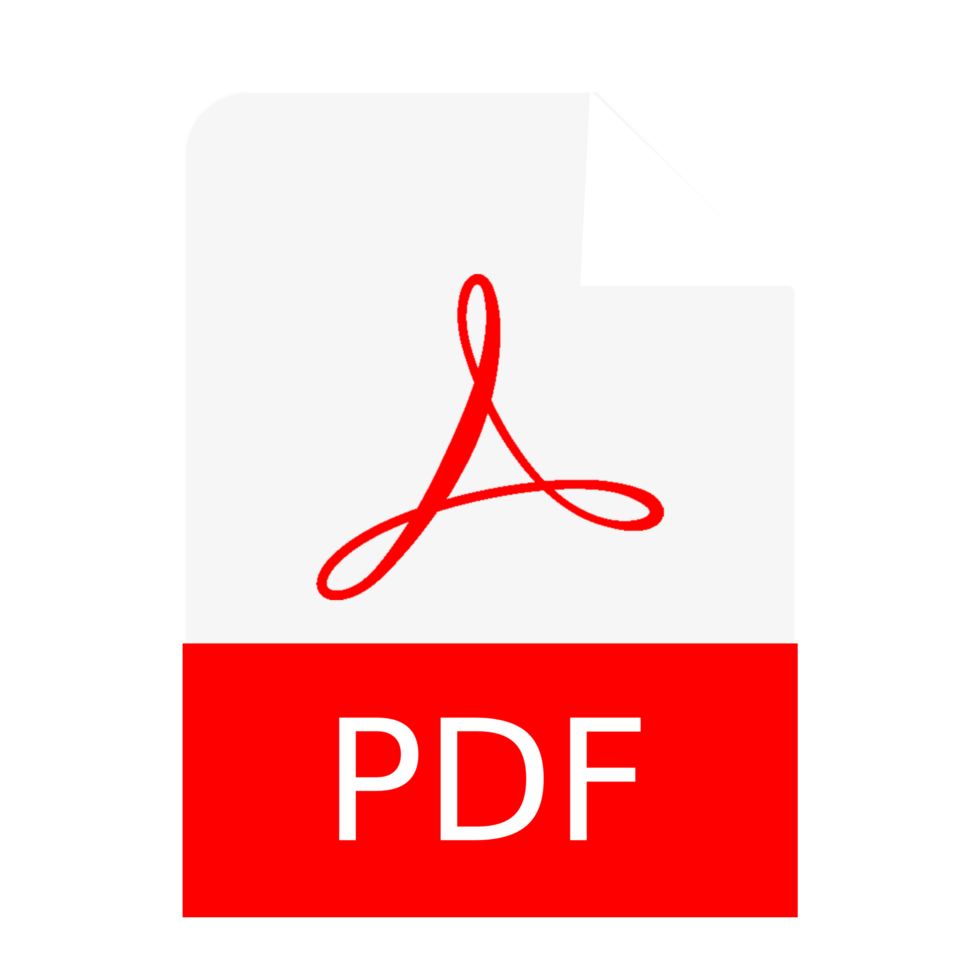