Modern Customer Portal
import logging
from flask import Flask, url_for
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from models import db, User
from abilities import apply_sqlite_migrations, flask_app_authenticator
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
# Set up authentication
app.before_request(flask_app_authenticator(
Frequently Asked Questions
How can the Modern Customer Portal benefit my business?
The Modern Customer Portal can significantly enhance your customer experience and streamline operations. It provides a secure, user-friendly interface for customers to access their accounts, view information, and interact with your services. This self-service approach can reduce support costs, increase customer satisfaction, and provide valuable insights into customer behavior and preferences.
Is the Modern Customer Portal suitable for small businesses?
Absolutely! The Modern Customer Portal is designed to be scalable and adaptable to businesses of all sizes. For small businesses, it offers an affordable way to provide a professional, secure online presence for customers. The portal's clean design and responsive layout ensure a great user experience across devices, which is crucial for small businesses looking to compete with larger enterprises.
Can the Modern Customer Portal be customized to match my brand identity?
Yes, the Modern Customer Portal is highly customizable. You can easily adjust the color scheme, add your logo, and modify the content to align with your brand. For example, in the styles.css
file, you can update the color variables to match your brand colors:
css
:root {
--primary-color: #YOUR_PRIMARY_COLOR;
--secondary-color: #YOUR_SECONDARY_COLOR;
--background-color: #YOUR_BACKGROUND_COLOR;
--text-color: #YOUR_TEXT_COLOR;
--border-color: #YOUR_BORDER_COLOR;
}
Additionally, you can replace the placeholder logo in the _desktop_header.html
file with your own logo.
How does the Modern Customer Portal handle user authentication?
The Modern Customer Portal uses a secure authentication system. It leverages Flask's session management for maintaining user sessions and includes routes for login, registration, and logout. The authentication process is set up in the main.py
file using the flask_app_authenticator
function. Here's a snippet showing how it's implemented:
python
app.before_request(flask_app_authenticator(
allowed_domains=None,
allowed_users=None,
logo_path=None,
app_title="My App",
custom_styles=None,
session_expiry=None
))
This setup ensures that only authenticated users can access protected routes, enhancing the security of your customer portal.
What industries could benefit most from implementing the Modern Customer Portal?
The Modern Customer Portal is versatile and can benefit a wide range of industries. It's particularly valuable for:
- Financial services: Providing secure access to account information and transactions
- Healthcare: Offering patient portals for appointment scheduling and medical record access
- E-commerce: Managing order history, returns, and customer preferences
- SaaS companies: Providing product usage information and support resources
- Utilities: Allowing customers to view bills, usage data, and make payments
The portal's flexibility allows it to be tailored to meet the specific needs of these and many other industries, improving customer engagement and operational efficiency.
Created: | Last Updated:
Here's a step-by-step guide for using the Modern Customer Portal template:
Introduction
This template provides a modern customer portal with secure login and registration functionality, featuring a clean, responsive design. It's built using Flask, SQLAlchemy, and Tailwind CSS, offering a solid foundation for a customer-facing web application.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
After starting with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment process and launch the Lazy CLI.
Using the Application
Once the application is deployed:
- Lazy will provide you with a dedicated server link to access your customer portal.
- Open this link in your web browser to view the home page of your customer portal.
Home Page
The home page of your customer portal will display:
- A welcome message
- A "Login" button
- A "Register" button
Registration
To set up new user accounts:
- Click the "Register" button on the home page.
- Fill in the registration form with an email address and password.
- Submit the form to create a new user account.
Login
For existing users to access the portal:
- Click the "Login" button on the home page.
- Enter the registered email address and password.
- Submit the form to log in.
User Dashboard
After successful login:
- Users will see their display name or email address in the header.
- A profile picture will be displayed if available.
- A "Logout" button will be present for ending the session.
Customization
To customize the application for your specific needs:
- Modify the
home.html
file to change the content of the home page. - Update the
styles.css
file to adjust the visual design. - Edit the
routes.py
file to add new pages or functionality to your portal.
Database Operations
The template includes basic database operations for user management:
- Creating new users
- Retrieving user information
- Updating user profile pictures
These operations are defined in the database_operations.py
file and can be extended as needed.
By following these steps, you'll have a functional customer portal that you can further customize and expand to meet your specific requirements.
Here are 5 key business benefits for this Modern Customer Portal template:
Template Benefits
-
Enhanced User Experience: The clean, responsive design ensures a seamless experience across devices, improving customer satisfaction and engagement.
-
Improved Security: Built-in authentication and secure session management protect user data and limit access to authorized individuals.
-
Scalable Architecture: The use of Flask, SQLAlchemy, and Gunicorn provides a robust foundation that can easily scale as the user base grows.
-
Easy Customization: The modular structure and use of Tailwind CSS allow for quick customization to match brand identity and specific business needs.
-
Efficient User Management: Automated user creation and profile picture updates streamline account management, reducing administrative overhead.
Technologies
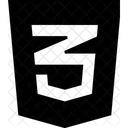
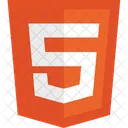