Job Search Website
import os
from flask import request
import logging
from flask import Flask, render_template
from gunicorn.app.base import BaseApplication
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__, static_folder='static')
from flask import render_template
@app.route("/")
def translate_form():
return render_template("home.html")
@app.route("/search", methods=['POST'])
def search_jobs():
search_query = request.json.get('query', '')
import requests
Frequently Asked Questions
How can this Job Search Website template benefit recruitment agencies?
The Job Search Website template offers significant advantages for recruitment agencies. It provides a clean, user-friendly interface that allows job seekers to easily search for positions. This can increase engagement and the number of applications received. The template's customizable nature means agencies can brand it to match their corporate identity, enhancing their professional image. Moreover, the real-time job search functionality powered by the RapidAPI integration ensures that the most up-to-date job listings are always available, improving the overall user experience and potentially increasing the agency's placement rates.
Can the Job Search Website template be integrated with other HR systems?
Yes, the Job Search Website template is designed with flexibility in mind. While it currently uses the JSearch API from RapidAPI, the backend structure allows for easy integration with other HR systems or job databases. To integrate with a different system, you would primarily need to modify the /search
route in the main.py
file. This adaptability makes the template suitable for companies that want to showcase their own job listings or for job boards that want to aggregate listings from multiple sources.
What are the potential applications of this template beyond job searching?
While the Job Search Website template is primarily designed for job listings, its structure can be adapted for various other search-based applications. For example: - Product catalogs: Businesses could use it to create a searchable product database. - Real estate listings: Real estate agencies could adapt it to showcase property listings. - Event directories: It could be modified to create a searchable event calendar. - Restaurant finders: With some adjustments, it could become a platform for searching local eateries. The template's core search functionality and card-based result display make it versatile for many search-oriented web applications.
How can I customize the appearance of the job cards in the search results?
To customize the appearance of the job cards, you'll need to modify the HTML structure and CSS classes in the search.js
file. Here's an example of how you could add more details to each job card:
javascript
jobCard.innerHTML = `
<h2 class="text-xl font-bold text-blue-600 mb-2">${job.employer_name}</h2>
<h3 class="text-lg font-semibold mb-1">${job.job_title}</h3>
<p class="text-sm text-gray-600 mb-2">${job.job_city}, ${job.job_country}</p>
<p class="text-sm text-gray-700">${job.job_description.substring(0, 100)}...</p>
<a href="${job.job_apply_link}" class="mt-2 inline-block bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600">Apply Now</a>
`;
This example assumes that the API response includes additional fields like job_city
, job_country
, job_description
, and job_apply_link
. You'll need to ensure these fields are available in the API response and adjust the search_jobs()
function in main.py
accordingly.
How can I implement pagination for the search results in the Job Search Website template?
Implementing pagination involves modifying both the frontend and backend code. Here's a basic example of how you could add pagination:
In main.py
, modify the search_jobs()
function:
python
@app.route("/search", methods=['POST'])
def search_jobs():
search_query = request.json.get('query', '')
page = request.json.get('page', 1)
url = "https://jsearch.p.rapidapi.com/search"
querystring = {"query":search_query,"page":str(page),"num_pages":"1"}
# ... rest of the function remains the same
return {"jobs": jobs, "total_pages": response.json().get('total_pages', 1)}
In search.js
, add pagination controls:
```javascript let currentPage = 1; const totalPages = data.total_pages;
// Add pagination controls to the page
const paginationControls = document.createElement('div');
paginationControls.innerHTML = <button id="prevPage" ${currentPage === 1 ? 'disabled' : ''}>Previous</button>
<span>Page ${currentPage} of ${totalPages}</span>
<button id="nextPage" ${currentPage === totalPages ? 'disabled' : ''}>Next</button>
;
jobsContainer.after(paginationControls);
// Add event listeners for pagination buttons document.getElementById('prevPage').addEventListener('click', () => { if (currentPage > 1) { currentPage--; performSearch(); } });
document.getElementById('nextPage').addEventListener('click', () => { if (currentPage < totalPages) { currentPage++; performSearch(); } });
function performSearch() { // Existing search code, but include the current page in the request fetch('/search', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ query: searchInput.value, page: currentPage }) }) // ... rest of the search function } ```
This implementation adds "Previous" and "Next" buttons to navigate through the search results pages. Remember to style these controls to match your template's design.
Created: | Last Updated:
Introduction to the Job Search Website Template
Welcome to the Job Search Website template guide. This template allows you to create a customizable one-page job search website with a search bar and a search button that displays UI cards of matching jobs. It's designed to be simple to use, even for non-technical builders, and Lazy handles all the deployment for you.
Getting Started
To begin building your job search website, click Start with this Template on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code.
Initial Setup: Adding Environment Secrets
Before you can use the job search functionality, you'll need to set up an environment secret for the API key:
- Sign up for a free account on RapidAPI.
- Once you have an account, navigate to the API you wish to use and subscribe to it to obtain your API key.
- In the Lazy Builder, go to the Environment Secrets tab.
- Add a new secret with the key
RAPIDAPI_KEY
and paste the API key you obtained from RapidAPI as the value.
Test: Deploying the App
With your environment secret in place, you're ready to deploy your app. Press the Test button in the Lazy Builder. This will launch the Lazy CLI and begin the deployment process.
Using the App
Once the app is deployed, Lazy will provide you with a dedicated server link. Use this link to access your job search website. You'll see a search bar where you can enter job titles or keywords, and a search button to initiate the search. The results will be displayed as UI cards with job titles and employer names.
Integrating the App
If you wish to integrate this job search functionality into an existing service or frontend, you can use the server link provided by Lazy. Add the link to your service where you want the job search feature to appear. If your service requires API endpoints, you can use the provided link to make requests to your job search app.
Here's a sample request you might make to the job search API:
fetch('YOUR_SERVER_LINK/search', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ query: 'Software Engineer' })
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error('Error:', error));
And a sample response from the API might look like this:
{
"jobs": [
{
"employer_name": "Tech Corp",
"job_title": "Senior Software Engineer"
},
{
"employer_name": "Innovatech",
"job_title": "Junior Software Developer"
}
]
}
Remember, all the steps above are mandatory to run and integrate the template. Follow them carefully to ensure your job search website works correctly. Happy building!
Template Benefits
-
Rapid Job Market Insights: This template provides businesses with a quick and easy way to gather real-time job market data, enabling them to stay informed about industry trends, skill demands, and competitive salary ranges.
-
Enhanced Recruitment Efficiency: HR departments and recruitment agencies can utilize this tool to streamline their job search process, quickly identifying potential candidates and job openings that match specific criteria.
-
Career Development Resource: Companies can offer this as a valuable resource for employees looking to advance their careers within the organization, fostering internal mobility and talent retention.
-
Market Research Tool: Business analysts and strategists can use this template to conduct market research, understanding the job landscape in various industries or regions to inform business decisions and expansion plans.
-
Custom Job Board Creation: Organizations can easily customize this template to create their own branded job boards, attracting talent directly to their website and potentially reducing reliance on external job posting platforms.
Technologies
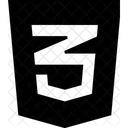
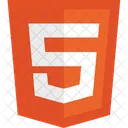
