by Lazy Sloth
Stripe Payment Gateway into Wordpress
import os
import logging
from fastapi import FastAPI, HTTPException
from fastapi.middleware.cors import CORSMiddleware
from pydantic import BaseModel, Field
import stripe
import uvicorn
# Constants
STRIPE_SECRET_KEY = os.environ['STRIPE_SECRET_KEY']
YOUR_DOMAIN = os.environ['YOUR_DOMAIN']
# Configure Stripe API key
stripe.api_key = STRIPE_SECRET_KEY
# FastAPI app initialization
app = FastAPI()
# CORS configuration
origins = ["*"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
Frequently Asked Questions
How can this Stripe payment gateway integration benefit my WordPress business?
This custom Stripe payment gateway integration for WordPress offers several benefits for your business: - Flexibility: You can easily set up various types of payment pages, including one-time payments and subscriptions. - UTM tracking: The template includes UTM parameter tracking, allowing you to measure the effectiveness of your marketing campaigns. - Customization: You have full control over the payment process and can tailor it to match your brand and user experience. - Scalability: As your business grows, this solution can easily accommodate different price points and products without major changes.
Can I use this template for both physical and digital products in my WordPress store?
Yes, this Stripe payment gateway integration is versatile and can be used for both physical and digital products in your WordPress store. The template creates a Stripe checkout session that can be customized based on your product type. For digital products, you might want to add instant delivery mechanisms, while for physical products, you could integrate shipping information collection. The flexibility of this template allows you to adapt it to various business models and product types.
How does this template help with marketing and conversion tracking?
This Stripe payment gateway integration includes built-in support for UTM parameters, which are crucial for marketing and conversion tracking. The template captures UTM data (source, medium, campaign, term, and content) from the URL and passes it to the Stripe checkout session as metadata. This allows you to: - Track which marketing channels are driving the most sales - Measure the ROI of your advertising campaigns - Understand customer behavior and optimize your marketing strategy By linking this data with your analytics tools, you can gain valuable insights into your sales funnel and improve your overall marketing effectiveness.
How can I modify the template to include custom fields in the checkout process?
To include custom fields in the checkout process, you'll need to modify both the backend and frontend code. Here's an example of how you might add a "customer_name" field:
Backend (Python): ```python class Data(BaseModel): # ... existing fields ... customer_name: str = Field(..., min_length=1)
@app.post('/create-checkout-session') def create_checkout_session(data: Data): # ... existing code ... session = stripe.checkout.Session.create( # ... existing parameters ... metadata={utm_params, 'customer_name': data.customer_name}, ) # ... rest of the function ... ```
Frontend (JavaScript):
javascript
async function initialize() {
// ... existing code ...
const customerName = document.getElementById('customer-name').value;
const response = await fetch("LAZY SERVER LINK/create-checkout-session", {
method: "POST",
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
months,
price: 'PRICE_ID',
customer_name: customerName,
...utmParams
})
});
// ... rest of the function ...
}
Remember to add the corresponding input field in your HTML for collecting the customer name.
How can I handle different subscription durations with this Stripe payment gateway template?
To handle different subscription durations, you'll need to create multiple price IDs in your Stripe dashboard for each duration (e.g., monthly, quarterly, annually) and modify the frontend code to allow selection of the appropriate price ID. Here's an example of how you might modify the frontend code:
```javascript function getPriceId(duration) { const priceIds = { 'monthly': 'price_monthly_id', 'quarterly': 'price_quarterly_id', 'annually': 'price_annually_id' }; return priceIds[duration] || 'price_monthly_id'; }
async function initialize() { const duration = document.getElementById('subscription-duration').value; const priceId = getPriceId(duration); const utmParams = getUTMParameters();
const response = await fetch("LAZY SERVER LINK/create-checkout-session", {
method: "POST",
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
months: 1, // This can be adjusted based on duration if needed
price: priceId,
...utmParams
})
});
// ... rest of the function ...
} ```
In this example, you would need to add a dropdown or radio buttons in your HTML to allow customers to select their preferred subscription duration. The getPriceId
function then maps the selected duration to the corresponding Stripe price ID. This approach allows you to offer multiple subscription options while still using the same Stripe payment gateway integration template.
Created: | Last Updated:
Introduction to the Stripe Payment Gateway Integration Template for WordPress
This template is designed to help you integrate a custom Stripe payment gateway into your WordPress site. It includes a backend service built with FastAPI, which handles the creation of Stripe checkout sessions and the retrieval of their statuses. The frontend code can be embedded into your WordPress payment page to allow users to make payments directly on your site. This solution is suitable for various payment setups, including one-time payments and subscriptions.
Getting Started with the Template
To begin using this template, click "Start with this Template" on the Lazy platform. This will set up the template in your Lazy Builder interface, ready for customization and deployment.
Initial Setup: Adding Environment Secrets
Before testing and deploying your app, you need to set up the required environment secrets. These are:
1. `STRIPE_SECRET_KEY`: Your Stripe secret API key, which you can find in your Stripe dashboard under Developers > API keys. 2. `YOUR_DOMAIN`: The domain where your WordPress site is hosted (e.g., https://www.yourwebsite.com).
To add these environment secrets in the Lazy Builder:
- Navigate to the Environment Secrets tab within the Lazy Builder. - Click on "Add Secret" and enter `STRIPE_SECRET_KEY` as the name and your Stripe secret API key as the value. - Add another secret with the name `YOUR_DOMAIN` and your website's domain as the value.
Test: Pressing the Test Button
Once you have added the environment secrets, press the "Test" button in the Lazy Builder. This will deploy your app and launch the Lazy CLI. If the app requires any user input, you will be prompted to provide it through the CLI.
Using the App
After testing, Lazy will provide you with a dedicated server link to use the API. This link is crucial for integrating the backend service with your WordPress site.
Integrating the App into WordPress
To integrate the backend service into your WordPress site, follow these steps:
1. Insert the provided frontend code into your WordPress payment page. You should place this script just before the `` tag in the HTML of your page.
2. Replace `"PUBLISHABLE STRIPE API KEY"` with your actual publishable API key from Stripe.
3. Replace `"LAZY SERVER LINK"` with the endpoint URL of your published app that you received after pressing the test button.
4. Replace `"PRICE_ID"` with the actual price ID you want to use for the transaction. You can find this in your Stripe dashboard under Products.
Here is the frontend code snippet you'll need to add to your WordPress page:
`
`
By following these steps, you will have successfully integrated a custom Stripe payment gateway into your WordPress site using the Lazy template. Your users will now be able to make secure payments directly on your site.
Template Benefits
-
Seamless E-commerce Integration: This template allows businesses to easily integrate a robust payment system into their WordPress sites, enabling smooth transactions and improving the overall shopping experience for customers.
-
Customizable Payment Solutions: With the ability to set different price points and subscription models, businesses can tailor their payment options to suit various products, services, or membership tiers, increasing flexibility in their offerings.
-
Enhanced Marketing Insights: The inclusion of UTM parameter tracking provides valuable data on marketing campaign effectiveness, allowing businesses to optimize their marketing strategies and improve ROI.
-
Improved Security and Compliance: By leveraging Stripe's secure payment infrastructure, businesses can ensure PCI compliance and protect sensitive customer data, building trust with their clientele.
-
Scalability and Performance: The use of FastAPI for the backend ensures high performance and scalability, allowing the payment system to handle increased traffic as the business grows without compromising on speed or reliability.
Technologies
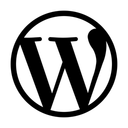
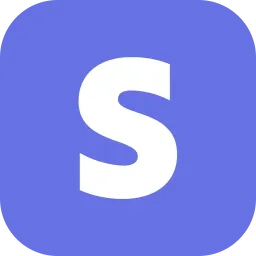
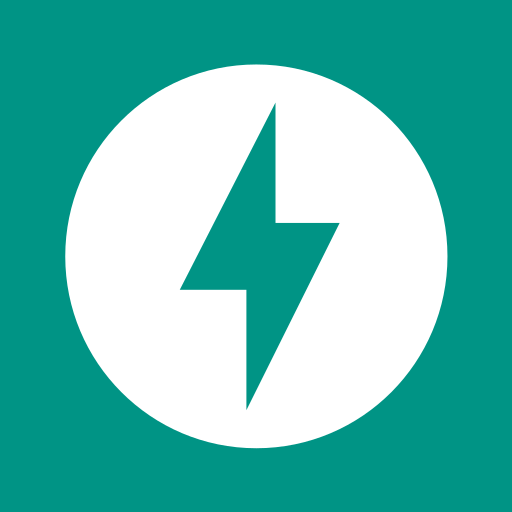