OpenAI GPT-4o Reminders WhatsApp bot
from typing import List
from db_utils import get_db_connection
from encryption_utils import decrypt
from db_utils import check_first_communication, insert_first_communication, insert_reminder, retrieve_reminders, delete_reminder, retrieve_all_reminders, update_timezone, retrieve_timezone, set_next_reminder_time
from time import sleep
import threading
from encryption_utils import encrypt
from timezone_finder import convert_timezone
from abilities import llm_prompt
from datetime import datetime, timedelta
from nlp_processing import process_reminder_input, process_timezone, process_action
from abilities import key_value_storage
import logging
import calendar
import requests
import json
import os
from twilio.twiml.messaging_response import MessagingResponse
from twilio.rest import Client
from flask import Flask, render_template, request
from gunicorn.app.base import BaseApplication
import random
import sqlite3
Frequently Asked Questions
How can businesses benefit from using the GPT-4o Reminders WhatsApp bot?
The GPT-4o Reminders WhatsApp bot offers several benefits for businesses: - Improved customer engagement through a familiar platform (WhatsApp) - Automated reminder system that reduces missed appointments or deadlines - Natural language processing capabilities that make it easy for customers to set reminders - Customizable reminders for various business needs (e.g., appointment reminders, payment due dates, follow-ups) - Potential for increased customer satisfaction and loyalty through timely, personalized reminders
What are some potential applications of the GPT-4o Reminders WhatsApp bot across different industries?
The GPT-4o Reminders WhatsApp bot can be applied in various industries: - Healthcare: Appointment reminders, medication schedules - Finance: Bill payment reminders, investment deadlines - Education: Assignment due dates, exam schedules - Retail: Sale notifications, order status updates - Hospitality: Reservation reminders, check-in/check-out times - Personal services: Haircut appointments, gym class schedules The bot's flexibility allows it to be adapted to many different use cases across industries.
How does the GPT-4o Reminders WhatsApp bot handle data privacy and security?
The GPT-4o Reminders WhatsApp bot takes several measures to ensure data privacy and security: - Encryption: User data is encrypted using AES encryption before being stored in the database - Secure communication: The bot uses Twilio's secure WhatsApp Business API for messaging - Limited data storage: Only necessary information is stored (phone numbers, reminders, timezones) - Environment variables: Sensitive information like API keys are stored as environment variables - HTTPS: The web application uses HTTPS for secure communication These measures help protect user data and comply with privacy regulations.
How can I customize the reminder processing in the GPT-4o Reminders WhatsApp bot?
You can customize the reminder processing by modifying the process_reminder_input
function in the nlp_processing.py
file. Here's an example of how you might add custom logic for specific types of reminders:
```python def process_reminder_input(input_text, phoneNumber, timezone): current_datetime = datetime.now().strftime("%Y-%m-%d %H:%M") prompt = f"Given the current datetime {current_datetime}, create a list of reminders from: '{input_text}'. "
# Add custom logic for specific reminder types
if "birthday" in input_text.lower():
prompt += "For birthdays, always set recurrent to True and recurrence_type to 'yearly'. "
elif "weekly meeting" in input_text.lower():
prompt += "For weekly meetings, set recurrent to True and recurrence_type to 'weekly'. "
# Rest of the function remains the same
response = client.chat.completions.create(
model="gpt-4o",
messages=[
{"role": "user", "content": prompt},
],
response_model=ReminderList,
)
return response
```
This customization allows the GPT-4o Reminders WhatsApp bot to handle specific types of reminders in a predefined way.
How can I extend the GPT-4o Reminders WhatsApp bot to support multiple languages?
To support multiple languages in the GPT-4o Reminders WhatsApp bot, you can modify the process_action
function in nlp_processing.py
. Here's an example of how you might implement this:
```python def process_action(input_text): prompt = f'''Based on the following user message in any language: "{input_text}", determine if the user wants to set a timezone/location, create a reminder, list reminders, or stop a reminder. Respond in the following JSON format: {{"action": "configure_timezone"}}, {{"action": "create"}}, {{"action": "list"}}, or {{"action": "stop"}}. If no action fits, use {{"action":"info"}}. Detect the language and include it in the response as "detected_language".'''
response = client.chat.completions.create(
model="gpt-4o",
messages=[
{"role": "user", "content": prompt},
],
response_model=UserActionWithLanguage,
)
return response
```
You would also need to create a new UserActionWithLanguage
model in reminder_structure.py
:
```python class UserActionWithLanguage(BaseModel): action: str detected_language: str
@validator('action')
def validate_action(cls, v):
valid_actions = ["configure_timezone", "create", "list", "stop", "info"]
if v not in valid_actions:
raise ValueError(f"Invalid action. Must be one of: {', '.join(valid_actions)}")
return v
```
This modification allows the GPT-4o Reminders WhatsApp bot to detect the language of the input and potentially respond in the same language, making it more accessible to users worldwide.
Created: | Last Updated:
Introduction to the GPT-4o Reminders WhatsApp Bot Template
The GPT-4o Reminders WhatsApp Bot is an innovative template that allows builders to create a WhatsApp bot capable of setting and managing reminders through chat. This bot leverages the power of GPT-4o to understand natural language inputs, making it easy for users to interact with and set reminders just by sending a message. Whether it's a one-time reminder or a recurring notification, this bot can handle it all seamlessly.
Getting Started with the Template
To begin building your own WhatsApp reminder bot, click
on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy or paste any code manually.
Initial Setup: Adding Environment Secrets
Before testing your bot, you'll need to set up some environment secrets within the Lazy Builder. These are crucial for the bot to interact with the Twilio API and to encrypt sensitive data.
Remember, these credentials are sensitive and should be kept secure.
Test: Deploying the App
Once you've set up the necessary environment secrets, you can deploy your app by clicking the
button. This will launch the Lazy CLI, and the deployment process will begin.
Entering Input: Providing User Input
If the bot requires any user input, the Lazy CLI will prompt you to provide it after pressing the
button. Follow the instructions in the CLI to enter the necessary information.
Using the App
After deployment, the Lazy platform will provide you with a dedicated server link. This link is where you can interact with your WhatsApp bot. Send a message to your WhatsApp number connected with Twilio, and the bot will respond accordingly, allowing you to set and manage reminders.
Integrating the App
To integrate the WhatsApp bot into your service, you may need to add the server link provided by Lazy to your Twilio WhatsApp sandbox or configure it in your Twilio settings. Follow these steps to complete the integration:
This will ensure that when users send a message to your WhatsApp number, Twilio will forward the message to your bot, and the bot will handle the reminders accordingly.
Sample API Request and Response
If you wish to interact with the bot's API directly, you can use the server link to send HTTP POST requests. Here's a sample request to set a reminder:
POST /wa HTTP/1.1
Host: [Your Server Link]
Content-Type: application/x-www-form-urlencoded
Body: From=whatsapp%3A%2B[YourPhoneNumber]&Body=Remind+me+to+call+John+tomorrow+at+9+AM
A successful response from the bot will confirm that the reminder has been set.
For further guidance and documentation on the Twilio API, you can refer to the official
.
By following these steps, you can create a fully functional WhatsApp reminder bot that helps users manage their tasks efficiently. Enjoy building with Lazy!
Here are the top 5 business benefits or applications of this GPT-4o Reminders WhatsApp bot template:
Template Benefits
-
Enhanced Customer Engagement: This WhatsApp bot provides a convenient and familiar platform for users to set reminders, improving customer engagement and satisfaction.
-
Increased Productivity: By automating the reminder process, businesses can help their clients or employees stay on top of important tasks and deadlines, boosting overall productivity.
-
Scalable Customer Service: The bot can handle multiple users simultaneously, providing 24/7 reminder services without the need for human intervention, thus reducing operational costs.
-
Personalized User Experience: Leveraging GPT-4o's natural language processing capabilities, the bot can understand and respond to user requests in a more human-like manner, creating a personalized experience.
-
Data-Driven Insights: By analyzing the types of reminders set and user interactions, businesses can gain valuable insights into customer behavior and preferences, informing future product or service improvements.
Technologies
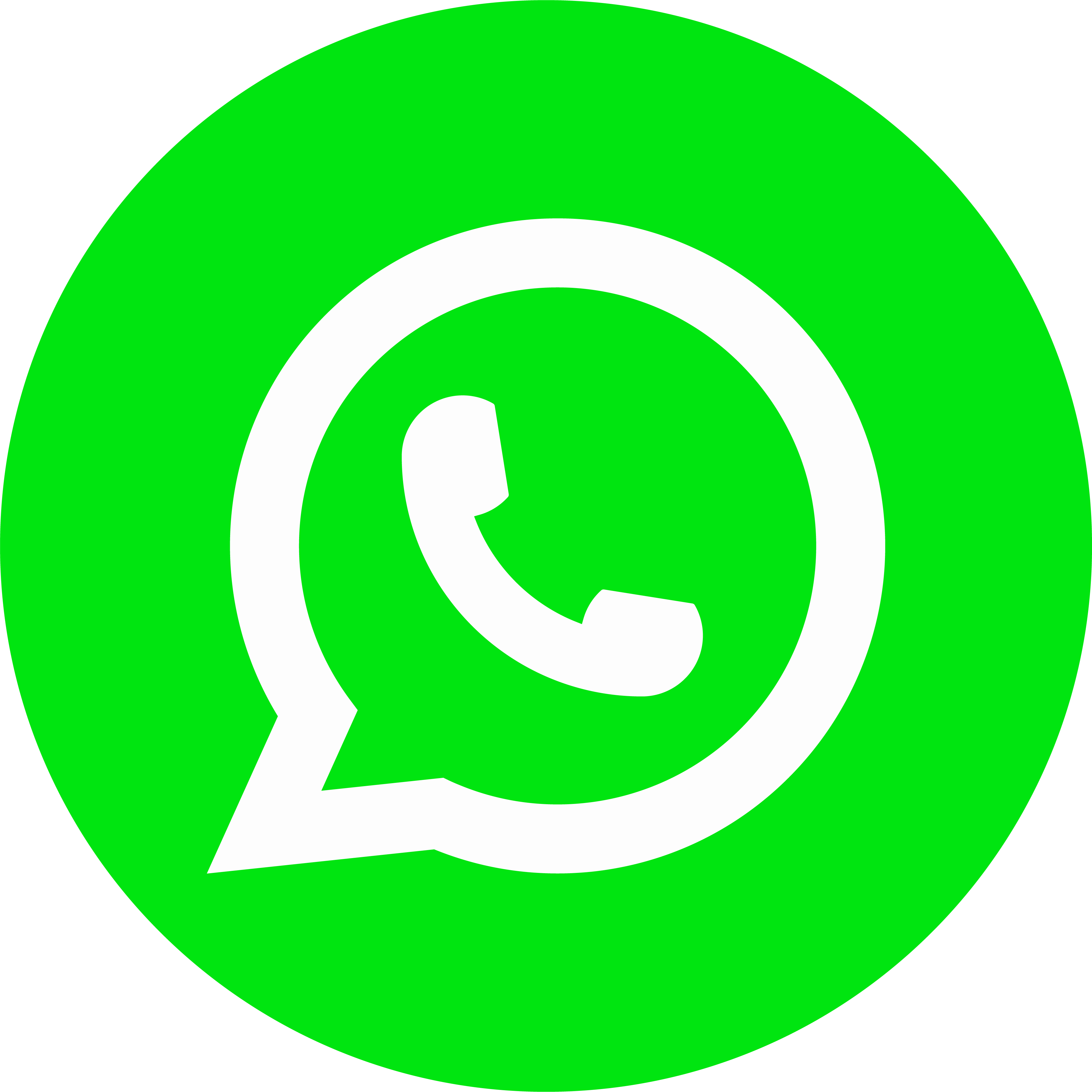