by Lazy Sloth
Get Address from Longitude and Latitude using Google Maps API (Google Reverse Geolocation)
import os
import requests
from fastapi import FastAPI, Form, HTTPException
from pydantic import BaseModel
app = FastAPI()
# The builder needs to provide the Google Maps API key via the Env Secrets tab.
# The environment variable name should be GOOGLE_MAPS_API_KEY.
@app.post("/get_location", summary="Get Location Data", description="Fetches location data based on latitude and longitude using Google Maps API.")
async def get_location(latitude: str = Form(...), longitude: str = Form(...)):
google_maps_api_key = os.environ.get('GOOGLE_MAPS_API_KEY')
if not google_maps_api_key:
raise HTTPException(status_code=500, detail="Google Maps API key is not set.")
try:
# The Google Maps Geocoding API endpoint
url = f"https://maps.googleapis.com/maps/api/geocode/json?latlng={latitude},{longitude}&key={google_maps_api_key}"
response = requests.get(url)
response.raise_for_status()
location_data = response.json()
# TODO: Parse the Google Maps API response and return the necessary location data
return location_data
Get Address from Longitude and Latitude using Google Maps API (Google Reverse Geolocation)
Created: | Last Updated:
Introduction to the Get Address from Longitude and Latitude Template
Welcome to the Lazy template guide for fetching address details from longitude and latitude using the Google Maps API. This template is a FastAPI web server that provides an endpoint to retrieve location data in JSON format. It's perfect for builders looking to integrate reverse geolocation features into their applications without delving into the complexities of API integration.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will set up the template in your Lazy Builder interface, ready for customization and deployment.
Initial Setup: Adding Environment Secrets
Before you can use the template, you need to provide a Google Maps API key. This key is essential for the application to interact with the Google Maps API and fetch location data.
- First, you'll need to obtain a Google Maps API key. Visit the Google Cloud Platform, create a project, and enable the Geocoding API to get your key.
- Once you have your API key, go to the Environment Secrets tab within the Lazy Builder.
- Add a new secret with the name
GOOGLE_MAPS_API_KEY
and paste your Google Maps API key as the value.
Test: Deploying the App
With the API key set up as an environment secret, you're ready to deploy the app. Press the "Test" button on the Lazy platform. This will initiate the deployment process and launch the Lazy CLI.
Using the App
After deployment, Lazy will provide you with a dedicated server link to access the API. Additionally, since this template uses FastAPI, you will also receive a link to the API documentation, which is automatically generated and provides an interactive interface to test the API endpoints.
To fetch location data, make a POST request to the /get_location
endpoint with the latitude and longitude as form data. Here's a sample request using curl:
curl -X 'POST' \
'http://your-server-link/get_location' \
-H 'accept: application/json' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'latitude=YOUR_LATITUDE&longitude=YOUR_LONGITUDE'
Replace http://your-server-link
with the server link provided by Lazy, and YOUR_LATITUDE
and YOUR_LONGITUDE
with the actual coordinates.
A successful request will return JSON-formatted location data. Here's an example of what the response might look like:
{
"plus_code": {
"compound_code": "P27Q+MF New York, USA",
"global_code": "87G8P27Q+MF"
},
"results": [ ... ],
"status": "OK"
}
Integrating the App
If you wish to integrate this API into an external tool or a frontend application, you will need to use the server link provided by Lazy. For instance, you can make AJAX calls from a web application to the API endpoint to retrieve location data dynamically.
Remember to handle the API key securely and not expose it in client-side code. Always make server-to-server requests to the FastAPI endpoint you've deployed on Lazy to keep your API key confidential.
That's it! You now have a fully functional API to get address details from longitude and latitude using the Google Maps API, all set up and ready to be integrated into your application.
Technologies
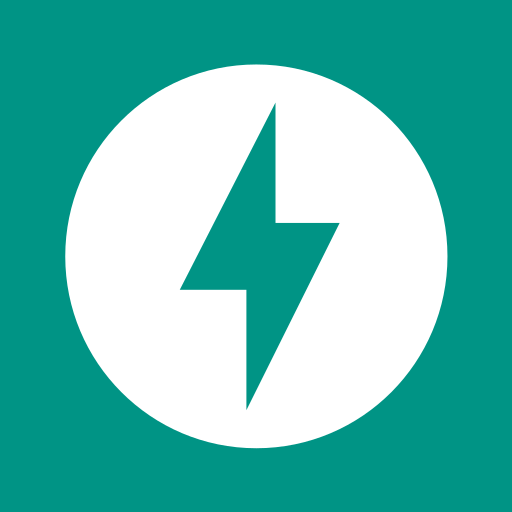
