by UnityAI
DuckDuckGo URL Indexer
import logging
import os
import requests
from typing import Optional
from fastapi import FastAPI, HTTPException
from fastapi.responses import RedirectResponse
from pydantic import BaseModel, HttpUrl
from abilities import apply_sqlite_migrations
from models import Base, engine, URL
from sqlalchemy.orm import Session
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
class URLSubmission(BaseModel):
url: HttpUrl
title: Optional[str] = None
description: Optional[str] = None
def submit_to_duckduckgo(url: str) -> bool:
"""Submit a URL to DuckDuckGo for indexing."""
try:
Frequently Asked Questions
What is the main purpose of the DuckDuckGo URL Indexer?
The DuckDuckGo URL Indexer is a FastAPI service designed to submit and index URLs for DuckDuckGo's search engine. It allows users to submit URLs along with optional titles and descriptions, which are then stored in a local database and submitted to DuckDuckGo for indexing. This tool can be particularly useful for website owners, SEO professionals, or anyone looking to improve their site's visibility on DuckDuckGo.
How can businesses benefit from using the DuckDuckGo URL Indexer?
Businesses can leverage the DuckDuckGo URL Indexer to: - Improve their website's visibility on DuckDuckGo's search engine - Quickly submit new pages or content for indexing - Track which URLs have been successfully indexed - Manage metadata (titles and descriptions) for their submitted URLs This can lead to increased organic traffic from DuckDuckGo and better overall search engine optimization.
Is the DuckDuckGo URL Indexer suitable for large-scale URL submission?
While the DuckDuckGo URL Indexer can handle multiple URL submissions, it's primarily designed for smaller-scale operations. For large-scale URL submission, you might need to implement additional features such as rate limiting, batch processing, or integration with a more robust database system. However, for small to medium-sized websites or periodic submissions, the current implementation of the DuckDuckGo URL Indexer should suffice.
How can I extend the DuckDuckGo URL Indexer to include additional metadata for submitted URLs?
To include additional metadata for submitted URLs, you'll need to modify both the URL
model in models.py
and the URLSubmission
Pydantic model in main.py
. Here's an example of how you could add a category
field:
In models.py
:
```python
class URL(Base):
tablename = 'urls'
id = Column(Integer, primary_key=True)
url = Column(String, unique=True, nullable=False)
title = Column(String, nullable=True)
description = Column(String, nullable=True)
indexed = Column(Boolean, default=False)
category = Column(String, nullable=True) # New field
```
In main.py
:
python
class URLSubmission(BaseModel):
url: HttpUrl
title: Optional[str] = None
description: Optional[str] = None
category: Optional[str] = None # New field
Don't forget to create a new migration file to add the category
column to the existing urls
table.
How can I modify the DuckDuckGo URL Indexer to support multiple search engines?
To support multiple search engines, you can create separate functions for each search engine's submission process and modify the submit_url
endpoint to use these functions. Here's a basic example:
```python def submit_to_google(url: str) -> bool: # Implement Google submission logic pass
def submit_to_bing(url: str) -> bool: # Implement Bing submission logic pass
@app.post("/submit") def submit_url(submission: URLSubmission, engines: List[str] = Query(default=["duckduckgo"])): try: with Session(engine) as session: url = URL( url=str(submission.url), title=submission.title, description=submission.description, indexed=False ) session.add(url) session.commit()
indexed_engines = []
for engine in engines:
if engine == "duckduckgo":
indexed = submit_to_duckduckgo(str(submission.url))
elif engine == "google":
indexed = submit_to_google(str(submission.url))
elif engine == "bing":
indexed = submit_to_bing(str(submission.url))
if indexed:
indexed_engines.append(engine)
url.indexed = len(indexed_engines) > 0
session.commit()
return {
"message": "URL submitted successfully",
"id": url.id,
"indexed_engines": indexed_engines
}
except Exception as e:
logger.error(f"Error submitting URL: {e}")
raise HTTPException(status_code=500, detail="Error submitting URL")
```
This modification allows the DuckDuckGo URL Indexer to submit URLs to multiple search engines based on the user's request.
Created: | Last Updated:
Here's a step-by-step guide for using the DuckDuckGo URL Indexer template:
Introduction
The DuckDuckGo URL Indexer is a FastAPI service that allows you to submit and index URLs for DuckDuckGo. This template provides a simple way to create and manage a database of URLs, and automatically submit them to DuckDuckGo for indexing.
Getting Started
-
Click "Start with this Template" to begin using the DuckDuckGo URL Indexer template in Lazy.
-
Press the "Test" button to deploy the application and launch the Lazy CLI.
Using the App
Once the app is deployed, you'll receive a server link to access the API. The API provides two main endpoints:
/submit
: Submit a new URL for indexing/urls
: List all submitted URLs
Submitting a URL
To submit a URL, you can use the /submit
endpoint. Here's a sample request:
POST /submit
{
"url": "https://example.com",
"title": "Example Website",
"description": "This is an example website"
}
The API will return a response indicating whether the URL was successfully submitted and indexed:
{
"message": "URL submitted successfully",
"id": 1,
"indexed": true
}
Listing URLs
To view all submitted URLs, you can use the /urls
endpoint:
GET /urls
This will return a list of all submitted URLs, including their ID, URL, title, description, and indexing status.
Integrating the App
To integrate this URL indexer into your workflow:
-
Use the provided API server link to make requests to the
/submit
endpoint whenever you want to submit a new URL for indexing. -
You can periodically check the
/urls
endpoint to monitor the status of submitted URLs. -
If you're building a frontend application, you can create forms or interfaces that interact with these API endpoints to provide a user-friendly way to submit and view indexed URLs.
Remember that this app automatically submits URLs to DuckDuckGo for indexing, so there's no need for additional steps to get your URLs indexed by DuckDuckGo's search engine.
By following these steps, you'll have a fully functional DuckDuckGo URL Indexer up and running, ready to help you manage and index your URLs efficiently.
Here are 5 key business benefits for this DuckDuckGo URL Indexer template:
Template Benefits
-
Improved Search Engine Visibility: By automating URL submissions to DuckDuckGo, businesses can enhance their online presence and increase the likelihood of their content being discovered by users of this privacy-focused search engine.
-
Efficient Content Management: The template provides a structured way to store and manage URLs along with their metadata (title, description), making it easier for businesses to organize and track their web content.
-
API-Driven Workflow: With its FastAPI implementation, the template offers a modern, high-performance API that can be easily integrated into existing content management systems or marketing automation tools, streamlining the process of URL submission.
-
Scalable Database Solution: Utilizing SQLAlchemy and SQLite, the template provides a robust and scalable database solution that can grow with the business, handling large numbers of URL submissions efficiently.
-
Monitoring and Reporting: The template includes logging functionality and the ability to track whether URLs have been successfully indexed, enabling businesses to monitor the effectiveness of their submission strategy and generate reports on their web presence.
Technologies
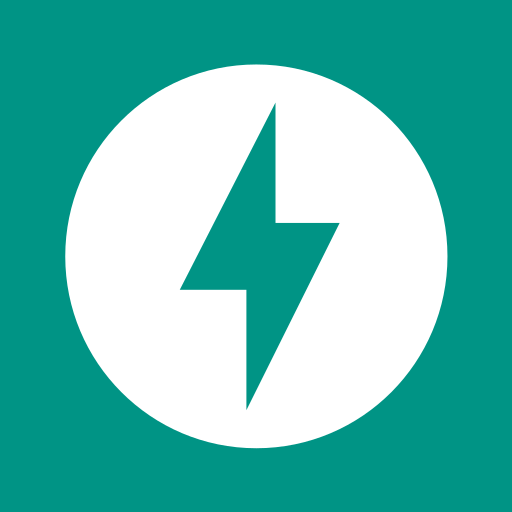