Text Input CSV to Excel Converter
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are some business applications for the CSV to Excel Converter?
The CSV to Excel Converter has numerous business applications across various industries. For example: - Financial departments can quickly convert CSV reports into Excel format for analysis and presentation. - Marketing teams can transform CSV data exports from analytics tools into Excel spreadsheets for campaign reporting. - HR departments can convert CSV employee data into Excel for easier manipulation and visualization. - Sales teams can convert CSV lead lists into Excel format for better organization and tracking.
How can the CSV to Excel Converter improve productivity in a business setting?
The CSV to Excel Converter can significantly boost productivity by: - Eliminating manual data entry and formatting when moving data from CSV to Excel. - Reducing the time spent on data conversion tasks, allowing employees to focus on analysis and decision-making. - Minimizing errors that can occur during manual data transfer between formats. - Providing a quick and accessible tool for all employees, regardless of their technical expertise.
Can the CSV to Excel Converter be customized for specific business needs?
Yes, the CSV to Excel Converter can be customized to meet specific business requirements. For instance: - Additional data processing steps can be added to the conversion process. - The user interface can be tailored to match company branding. - Integration with other business systems or databases can be implemented. - Specific Excel formatting or formulas can be applied automatically during the conversion process.
How can I add custom Excel formatting to the converted file in the CSV to Excel Converter?
You can customize the Excel formatting by modifying the convert_csv
function in the routes.py
file. Here's an example of how to add basic formatting:
```python @app.route("/convert", methods=["POST"]) def convert_csv(): try: csv_text = request.json.get("csv_text", "") df = pd.read_csv(io.StringIO(csv_text))
excel_buffer = io.BytesIO()
with pd.ExcelWriter(excel_buffer, engine='xlsxwriter') as writer:
df.to_excel(writer, index=False, sheet_name='Sheet1')
# Get the xlsxwriter workbook and worksheet objects
workbook = writer.book
worksheet = writer.sheets['Sheet1']
# Add a format for the header
header_format = workbook.add_format({
'bold': True,
'text_wrap': True,
'valign': 'top',
'fg_color': '#D7E4BC',
'border': 1
})
# Write the column headers with the format
for col_num, value in enumerate(df.columns.values):
worksheet.write(0, col_num, value, header_format)
excel_buffer.seek(0)
return send_file(excel_buffer, mimetype='application/vnd.openxmlformats-officedocument.spreadsheetml.sheet',
as_attachment=True, download_name='converted.xlsx')
except Exception as e:
logger.error(f"Error converting CSV: {str(e)}")
return jsonify({"error": str(e)}), 400
```
This code adds a formatted header row to the Excel file during the conversion process.
How can I extend the CSV to Excel Converter to handle larger file sizes?
To handle larger file sizes in the CSV to Excel Converter, you can implement chunked processing and streaming. Here's an example of how to modify the convert_csv
function to handle larger files:
```python from flask import Response import csv
@app.route("/convert", methods=["POST"]) def convert_csv(): try: csv_text = request.json.get("csv_text", "") csv_file = io.StringIO(csv_text) csv_reader = csv.reader(csv_file)
def generate():
excel_buffer = io.BytesIO()
workbook = xlsxwriter.Workbook(excel_buffer, {'in_memory': True})
worksheet = workbook.add_worksheet()
for row_index, row in enumerate(csv_reader):
for col_index, cell in enumerate(row):
worksheet.write(row_index, col_index, cell)
if row_index % 1000 == 0: # Flush every 1000 rows
excel_buffer.seek(0)
data = excel_buffer.getvalue()
excel_buffer.seek(0)
excel_buffer.truncate()
yield data
workbook.close()
excel_buffer.seek(0)
yield excel_buffer.getvalue()
return Response(generate(), mimetype='application/vnd.openxmlformats-officedocument.spreadsheetml.sheet',
headers={"Content-Disposition": "attachment;filename=converted.xlsx"})
except Exception as e:
logger.error(f"Error converting CSV: {str(e)}")
return jsonify({"error": str(e)}), 400
```
This implementation uses a generator function to process the CSV data in chunks and stream the Excel file to the client, allowing for better memory management when dealing with large files in the CSV to Excel Converter.
Created: | Last Updated:
Here's a step-by-step guide for using the CSV to Excel Converter template:
Introduction
The CSV to Excel Converter is a web application that allows users to easily convert CSV-formatted text into downloadable Excel files. This template provides a simple interface for users to input their CSV data and receive a converted Excel file with just a click.
Getting Started
- Click "Start with this Template" to begin using the CSV to Excel Converter template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and launch the application.
-
Once the deployment is complete, Lazy will provide you with a dedicated server link to access the web application.
Using the CSV to Excel Converter
-
Open the provided server link in your web browser to access the CSV to Excel Converter interface.
-
You'll see a text area where you can enter your CSV data. The placeholder text provides an example of the expected format:
Name,Age,City
John,25,New York
Jane,30,London
-
Enter your CSV data into the text area, following the format shown in the example.
-
Once you've entered your CSV data, click the "Download Excel" button.
-
The application will process your CSV data and generate an Excel file. Your browser will automatically download the converted file named "converted.xlsx".
-
Open the downloaded Excel file to verify that your CSV data has been correctly converted.
Additional Notes
- The application supports multiple rows and columns of CSV data.
- Make sure your CSV data is properly formatted with commas separating each value and new lines for each row.
- If you encounter any issues with the conversion, double-check that your CSV data is correctly formatted and try again.
By following these steps, you can easily convert CSV data to Excel format using this template. The application provides a straightforward way to transform your data without the need for complex software or manual conversion processes.
Template Benefits
-
Improved Data Processing Efficiency: This template allows users to quickly convert CSV data to Excel format, streamlining data processing workflows and saving time for businesses that frequently work with different data formats.
-
Enhanced Data Accessibility: By providing an easy-to-use web interface for CSV to Excel conversion, this tool makes data more accessible to team members who are more comfortable working with Excel spreadsheets, potentially improving data analysis and decision-making processes.
-
Cost-Effective Solution: As a self-hosted web application, this template offers a free alternative to paid CSV-to-Excel conversion services, reducing software expenses for businesses, especially small to medium-sized enterprises.
-
Increased Data Security: By allowing in-house conversion of sensitive CSV data to Excel format, companies can maintain better control over their information, reducing the risk of data breaches that might occur when using third-party conversion services.
-
Flexible Integration Potential: The modular structure of this template makes it easy to integrate the CSV-to-Excel conversion functionality into existing business applications or workflows, enhancing the overall efficiency of data management systems.
Technologies
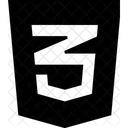
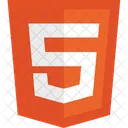