Cricket Clash: The Ultimate Text-Based Cricket Game
import logging
from flask import Flask, url_for, request, session, jsonify
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from authentication import auth, auth_required
from models import db, User, Game, GameState
from abilities import apply_sqlite_migrations
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
app.register_blueprint(auth)
Frequently Asked Questions
How can Cricket Clash be monetized as a web-based game?
Cricket Clash offers several monetization opportunities:
What are the potential target markets for Cricket Clash?
Cricket Clash has broad appeal to several target markets:
How can Cricket Clash leverage user data to improve the game and business?
Cricket Clash can utilize user data in several ways:
How can I implement a leaderboard feature in Cricket Clash?
To add a leaderboard to Cricket Clash, you can modify the models.py
file to include a new table for storing user scores, and update the routes.py
file to include a new route for displaying the leaderboard. Here's an example:
In models.py
, add:
```python class Leaderboard(db.Model): id = db.Column(db.Integer, primary_key=True) user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False) score = db.Column(db.Integer, nullable=False) timestamp = db.Column(db.DateTime, default=datetime.utcnow)
user = db.relationship('User', backref=db.backref('leaderboard_entries', lazy=True))
```
In routes.py
, add:
python
@routes.route("/leaderboard")
def leaderboard_route():
top_scores = db.session.query(User, func.max(Leaderboard.score).label('max_score')).\
join(Leaderboard).\
group_by(User.id).\
order_by(desc('max_score')).\
limit(10).all()
return render_template("leaderboard.html", top_scores=top_scores)
This implementation allows Cricket Clash to display a leaderboard of the top 10 players based on their highest scores.
How can I implement a simple AI opponent in Cricket Clash?
To add a simple AI opponent in Cricket Clash, you can modify the play_ball
function in routes.py
. Here's an example of how you could implement this:
```python import random
def ai_play(): return random.choice(['defensive', 'normal', 'aggressive'])
@routes.route("/api/game/
player_action = request.json.get('action')
ai_action = ai_play()
# Compare player_action and ai_action to determine the outcome
if player_action == ai_action:
outcome = "It's a dot ball!"
elif (player_action == 'aggressive' and ai_action == 'defensive') or \
(player_action == 'normal' and ai_action == 'aggressive') or \
(player_action == 'defensive' and ai_action == 'normal'):
outcome = "You scored runs!"
current_state['score'] += random.randint(1, 6)
else:
outcome = "You're out!"
current_state['wickets'] += 1
current_state['last_ball'] = outcome
# ... rest of the existing code ...
```
This implementation adds a simple AI opponent that randomly chooses its action, and then compares it with the player's action to determine the outcome. This enhances Cricket Clash by providing a more interactive and challenging gameplay experience.
Created: | Last Updated:
Here's a step-by-step guide for using the Cricket Clash: The Ultimate Text-Based Cricket Game template:
Introduction
Cricket Clash is an exciting web-based, text-based cricket game featuring multiplayer gameplay, user authentication, advanced mechanics, a customizable dashboard, tournaments, and player stats. This template provides a solid foundation for building a comprehensive cricket game experience.
Getting Started
-
Click "Start with this Template" to begin working with the Cricket Clash template in the Lazy Builder interface.
-
Press the "Test" button to deploy the application and launch the Lazy CLI.
Using the App
Once the app is deployed, you can access the game through the provided server link. The game features the following components:
- Landing Page: Introduces players to Cricket Clash and its features.
- User Authentication: Players can log in to access the game.
- Home Page: Displays player statistics and allows starting a new match.
- Game Page: The main interface for playing cricket matches.
Playing a Match
- On the Home Page, click the "Start New Match" button to begin a game.
- On the Game Page, you'll see the following elements:
- Score, wickets, and overs information
- Commentary box
- Power and Timing meters
-
Shot selection buttons (Defensive, Normal, Aggressive)
-
To play a ball:
- Press the spacebar to start the Power meter
- Press spacebar again to stop the Power meter and start the Timing meter
-
Press spacebar a third time to stop the Timing meter and execute the shot
-
The game will calculate the outcome based on your shot selection, power, and timing.
-
Continue playing until you reach the target score, lose all wickets, or complete all overs.
Customization and Expansion
To further develop Cricket Clash, consider the following enhancements:
- Implement a multiplayer system for player-vs-player matches.
- Add a tournament mode with brackets and schedules.
- Expand player statistics and create leaderboards.
- Introduce more advanced cricket mechanics, such as fielding positions and bowling variations.
- Develop a player progression system with skills and upgrades.
By using this template as a starting point, you can create a fully-featured cricket game with rich gameplay and engaging user experiences.
Template Benefits
-
Engaging User Experience: This template provides an immersive cricket game experience, which can attract and retain users, potentially increasing user engagement and time spent on the platform.
-
Monetization Opportunities: The game structure allows for various monetization strategies, such as in-game purchases, premium features, or subscription-based access to tournaments, creating revenue streams for the business.
-
Data Collection and Analytics: With user authentication and gameplay tracking, the template enables valuable data collection on user behavior and preferences, which can be used for targeted marketing, game improvements, and business insights.
-
Scalable Architecture: The template's use of Flask, SQLAlchemy, and Gunicorn provides a solid foundation for a scalable web application, allowing the business to grow and expand the user base without major architectural changes.
-
Cross-Platform Accessibility: Being a web-based game, it's accessible across various devices and platforms, maximizing the potential user base and reducing development costs compared to native mobile apps.
Technologies
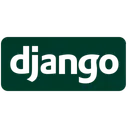
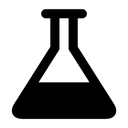
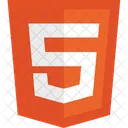

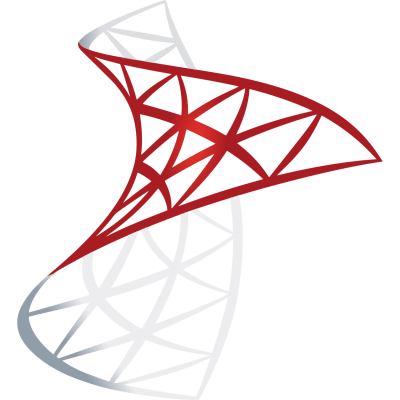