Control de Expedientes Judiciales
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
from flask import jsonify, request
from models import Expediente
from database import db
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
@app.route('/api/expedientes', methods=['POST'])
def crear_expediente():
data = request.json
nuevo_expediente = Expediente(
numero_expediente=data['numeroExpediente'],
partes=data['partes'],
fecha_inicio=data['fechaInicio'],
estado=data['estado']
)
db.session.add(nuevo_expediente)
Frequently Asked Questions
How can the Control de Expedientes Judiciales application benefit a law firm?
The Control de Expedientes Judiciales application can significantly improve a law firm's efficiency by providing a centralized system for managing judicial case files. It allows for easy registration of new cases, tracking of ongoing cases, and quick retrieval of case information. This streamlined process can save time, reduce errors, and enhance overall case management, ultimately leading to better client service and improved productivity within the firm.
Is it possible to customize the Control de Expedientes Judiciales template for different types of legal practices?
Yes, the Control de Expedientes Judiciales template is designed to be flexible and can be customized for various legal practices. While the current implementation includes basic fields such as case number, involved parties, start date, and status, you can easily modify the Expediente
model in models.py
to include additional fields specific to your practice area. For example, a criminal law practice might add fields for charges or sentencing information, while a civil litigation practice could include fields for damages sought or settlement offers.
How does the Control de Expedientes Judiciales application ensure data security for sensitive case information?
The Control de Expedientes Judiciales application takes several measures to ensure data security. It uses SQLite as the database, which provides built-in security features. Additionally, the application can be further secured by implementing user authentication, encrypting sensitive data, and setting up proper access controls. It's important to note that for production use, you should consider using a more robust database system like PostgreSQL and implement additional security measures such as HTTPS and regular backups.
How can I add a search functionality to the Control de Expedientes Judiciales application?
To add a search functionality to the Control de Expedientes Judiciales application, you can modify the obtener_expedientes
route in main.py
to accept search parameters. Here's an example of how you could implement a simple search by case number:
python
@app.route('/api/expedientes', methods=['GET'])
def obtener_expedientes():
search_query = request.args.get('search', '')
expedientes = Expediente.query.filter(Expediente.numero_expediente.like(f'%{search_query}%')).all()
return jsonify([{
'id': e.id,
'numeroExpediente': e.numero_expediente,
'partes': e.partes,
'fechaInicio': e.fecha_inicio.isoformat(),
'estado': e.estado
} for e in expedientes])
You would also need to update the frontend in home.js
to send the search query to this endpoint and display the results.
How can I extend the Control de Expedientes Judiciales template to include file uploads for each case?
To include file uploads for each case in the Control de Expedientes Judiciales template, you'll need to make several changes. First, update the Expediente
model in models.py
to include a relationship with a new Archivo
model:
```python from database import db from datetime import datetime
class Expediente(db.Model): # ... existing fields ... archivos = db.relationship('Archivo', backref='expediente', lazy=True)
class Archivo(db.Model): id = db.Column(db.Integer, primary_key=True) nombre = db.Column(db.String(255), nullable=False) ruta = db.Column(db.String(255), nullable=False) expediente_id = db.Column(db.Integer, db.ForeignKey('expediente.id'), nullable=False) fecha_subida = db.Column(db.DateTime, default=datetime.utcnow) ```
Then, create a new route in main.py
to handle file uploads:
```python import os from werkzeug.utils import secure_filename
UPLOAD_FOLDER = 'uploads' app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
@app.route('/api/expedientes/
Finally, update the frontend to include a file upload form and functionality to send files to this new endpoint. This extension allows users to attach relevant documents to each case in the Control de Expedientes Judiciales system.
Created: | Last Updated:
Here's a step-by-step guide for using the Control de Expedientes Judiciales template:
Introduction
The Control de Expedientes Judiciales template provides a web application for managing and recording judicial case files in a law office. It offers functionality to list and store basic information for each case file.
Getting Started
- Click "Start with this Template" to begin using the Control de Expedientes Judiciales template in the Lazy Builder interface.
Test the Application
- Press the "Test" button to deploy the application and launch the Lazy CLI.
Using the Application
-
Once the application is deployed, Lazy will provide you with a dedicated server link to access the web interface.
-
Open the provided link in your web browser to access the Control de Expedientes Judiciales application.
-
The main page of the application will display a form for registering new case files and a table listing existing case files.
-
To register a new case file:
- Fill in the "Número de Expediente" (Case Number) field
- Enter the "Partes Involucradas" (Involved Parties)
- Select the "Fecha de Inicio" (Start Date)
- Choose the "Estado" (Status) from the dropdown menu
-
Click the "Registrar Expediente" (Register Case File) button
-
The newly registered case file will appear in the table below the form.
-
The table displays all registered case files with their respective details:
- Número de Expediente (Case Number)
- Partes Involucradas (Involved Parties)
- Fecha de Inicio (Start Date)
-
Estado (Status)
-
The application is responsive and works on both desktop and mobile devices. On smaller screens, a mobile menu is available for navigation.
By following these steps, you can effectively use the Control de Expedientes Judiciales template to manage and track judicial case files in your law office.
Here are 5 key business benefits for this "Control de Expedientes Judiciales" template:
Template Benefits
-
Improved Case Management Efficiency: This template provides a streamlined system for legal professionals to register, track, and manage judicial cases, significantly reducing administrative overhead and improving overall case management efficiency.
-
Enhanced Data Organization and Accessibility: By digitizing case information and providing a structured database, the template ensures that critical case details are easily accessible and well-organized, enabling quick retrieval and analysis of case information.
-
Increased Productivity: The user-friendly interface for case registration and listing allows legal staff to quickly input and view case information, saving time and increasing productivity in daily operations.
-
Better Case Status Tracking: With the ability to set and update case statuses (active, waiting, closed), legal teams can more effectively prioritize their workload and ensure timely follow-ups on pending cases.
-
Scalable and Customizable Solution: Built with modern web technologies and a modular structure, this template serves as a solid foundation that can be easily expanded and customized to meet the specific needs of different legal practices or judicial offices.
Technologies
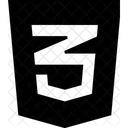
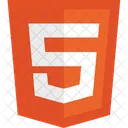
