by lithun419
Chatbot Interface Builder
import logging
from flask import Flask, render_template, session
from flask_session import Session
from gunicorn.app.base import BaseApplication
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__)
# Configuring server-side session
app.config["SESSION_PERMANENT"] = False
app.config["SESSION_TYPE"] = "filesystem"
Session(app)
from abilities import llm
from flask import request, jsonify
@app.route("/")
def root_route():
return render_template("template.html")
@app.route("/send_message", methods=['POST'])
def send_message():
Frequently Asked Questions
How can businesses benefit from implementing the Chatbot Interface Builder?
The Chatbot Interface Builder offers numerous benefits for businesses. It provides a professional-looking, responsive web interface for AI-powered chatbots, which can enhance customer service, streamline support processes, and provide 24/7 assistance. By implementing this template, businesses can quickly deploy a customized chatbot solution that integrates seamlessly with their existing websites, improving user engagement and potentially reducing operational costs associated with customer support.
Can the Chatbot Interface Builder be customized for different industries?
Absolutely! The Chatbot Interface Builder is designed to be flexible and adaptable to various industries. While the template provides a sleek, modern design out of the box, it can be easily customized to match specific brand guidelines or industry requirements. For example, a healthcare provider could modify the color scheme and add industry-specific prompts, while a retail business might integrate product recommendations into the chat flow. The template's modular structure allows for easy modifications to suit diverse business needs.
What are the potential applications of the Chatbot Interface Builder in e-commerce?
In e-commerce, the Chatbot Interface Builder can be a game-changer. It can be used to create intelligent shopping assistants that help customers find products, answer questions about shipping and returns, and provide personalized recommendations. The chatbot can also handle order tracking, process simple returns, and even upsell or cross-sell products based on customer interactions. By implementing this template, e-commerce businesses can provide a more interactive and personalized shopping experience, potentially increasing conversion rates and customer satisfaction.
How can I modify the Chatbot Interface Builder to include custom styling for specific messages?
The Chatbot Interface Builder uses Tailwind CSS for styling, making it easy to customize the appearance of messages. To add custom styling for specific message types, you can modify the script.js
file. Here's an example of how you might add a new message type with custom styling:
```javascript const addMessage = (text, type) => { const message = document.createElement('div'); message.textContent = text; switch(type) { case 'user': message.className = 'p-2 my-2 text-right bg-blue-900 rounded'; break; case 'bot': message.className = 'p-2 my-2 text-left bg-purple-900 rounded'; break; case 'error': message.className = 'p-2 my-2 text-left bg-red-900 rounded text-yellow-300'; break; // Add more cases for different message types } chat.appendChild(message); };
// Usage addMessage('Hello, how can I help you?', 'bot'); addMessage('I have a question about my order', 'user'); addMessage('Sorry, there was an error processing your request', 'error'); ```
This modification allows you to easily add new message types with distinct styling, enhancing the visual communication in your chatbot interface.
How can I extend the Chatbot Interface Builder to support file uploads?
To add file upload functionality to the Chatbot Interface Builder, you'll need to modify both the frontend and backend. Here's a basic example of how you might implement this:
First, add a file input to the HTML in template.html
:
html
<input type="file" id="fileInput" class="w-full mt-2 p-2 border border-gray-700 rounded bg-gray-700 text-white">
<button id="uploadButton" class="w-full mt-2 bg-green-600 hover:bg-green-700 text-white font-bold py-2 px-4 rounded">
Upload File
</button>
Then, modify script.js
to handle file uploads:
```javascript const uploadButton = document.getElementById('uploadButton'); const fileInput = document.getElementById('fileInput');
uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; if (file) { const formData = new FormData(); formData.append('file', file);
fetch('/upload_file', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => {
addMessage(`File uploaded: ${data.filename}`, 'system');
})
.catch(error => {
console.error('Error:', error);
addMessage('Error uploading file', 'error');
});
}
}); ```
Finally, add a new route in main.py
to handle file uploads:
```python import os from werkzeug.utils import secure_filename
UPLOAD_FOLDER = 'uploads' app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
@app.route('/upload_file', methods=['POST']) def upload_file(): if 'file' not in request.files: return jsonify({'error': 'No file part'}), 400 file = request.files['file'] if file.filename == '': return jsonify({'error': 'No selected file'}), 400 if file: filename = secure_filename(file.filename) file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename)) return jsonify({'filename': filename}), 200 ```
This implementation allows users to upload files through the Chatbot Interface Builder, extending its functionality for more complex interactions.
Created: | Last Updated:
Here's a step-by-step guide for using the Chatbot Interface Builder template:
Introduction
This template provides a web interface for a chatbot using an AI language model. It features a clean, responsive design with Tailwind CSS styling and a Flask backend. The chatbot maintains conversation history and uses the GPT-4 model for generating responses.
Getting Started
- Click "Start with this Template" to begin using the Chatbot Interface Builder in your Lazy project.
Test the Application
-
Press the "Test" button in the Lazy interface to deploy and run the application.
-
Once deployed, Lazy will provide you with a server link to access your chatbot interface.
Using the Chatbot Interface
-
Open the provided server link in your web browser to access the chatbot interface.
-
You'll see a chat window with an input field at the bottom.
-
Type your message in the input field and press "Send" or hit Enter to send your message to the chatbot.
-
The chatbot will process your message and respond, maintaining the conversation history for context.
Customizing the Chatbot
To customize the chatbot's appearance or behavior, you can modify the following files:
template.html
: Adjust the HTML structure and Tailwind CSS classes to change the layout and styling.script.js
: Modify the JavaScript to alter the chat interaction behavior.main.py
: Update the Flask routes or chatbot logic in the backend.
For example, to change the chatbot's name, you can update the header in template.html
:
html
<span class="ml-3 text-xl">Your Custom Chatbot Name</span>
Integrating the Chatbot
This chatbot interface is designed to work as a standalone web application. You can embed it into an existing website by using an iframe or by incorporating the HTML, CSS, and JavaScript into your site's structure.
To embed the chatbot using an iframe, use the following HTML code, replacing YOUR_SERVER_LINK
with the link provided by Lazy:
```html
```
Remember to adjust the width and height attributes as needed to fit your website's design.
By following these steps, you'll have a functional AI-powered chatbot interface up and running, which you can further customize to suit your specific needs.
Here are 5 key business benefits for this Chatbot Interface Builder template:
Template Benefits
-
Rapid Deployment of AI Customer Support: Businesses can quickly implement an AI-powered customer support chatbot, reducing response times and improving customer satisfaction without the need for extensive development resources.
-
Customizable User Interface: The template uses Tailwind CSS, allowing for easy customization of the chatbot's appearance to match brand guidelines and create a cohesive user experience across platforms.
-
Scalable Architecture: Built with Flask and Gunicorn, the template provides a solid foundation for scaling the chatbot service as user demand grows, ensuring consistent performance under increasing loads.
-
Enhanced User Engagement: The real-time chat interface with typing indicators creates a more engaging and interactive experience for users, potentially increasing time spent on the site and improving conversion rates.
-
Conversation History Management: The implementation of session-based conversation history allows for more contextually relevant responses, leading to improved accuracy in addressing user queries and a more personalized interaction.
Technologies
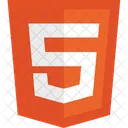
