by yasinay118
Calculator
import logging
import os
from flask import Flask, render_template, request
app = Flask(__name__)
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO)
@app.route('/', methods=['GET', 'POST'])
def calculator():
result = None
if request.method == 'POST':
try:
num1 = float(request.form['num1'])
num2 = float(request.form['num2'])
operation = request.form['operation']
if operation == 'add':
result = num1 + num2
elif operation == 'subtract':
result = num1 - num2
elif operation == 'multiply':
result = num1 * num2
elif operation == 'divide':
Frequently Asked Questions
How can this Calculator template be customized for specific business needs?
The Calculator template can be easily customized to suit various business needs. For example, you could modify it to create a financial calculator for loan payments, a pricing calculator for e-commerce sites, or a conversion tool for units of measurement. By adjusting the HTML and Python code, you can add new operations, change the user interface, or integrate it with other business systems.
What are some potential applications of this Calculator template in different industries?
The Calculator template has numerous applications across various industries: - Real Estate: Calculate mortgage payments or property valuations - Finance: Compute interest rates, investment returns, or tax calculations - Manufacturing: Estimate material costs or production outputs - Healthcare: Calculate BMI, drug dosages, or insurance copayments - Education: Create interactive math learning tools
How can the Calculator template improve customer engagement on a business website?
Integrating the Calculator template into a business website can significantly enhance customer engagement by providing interactive and valuable tools. It allows customers to perform quick calculations relevant to your products or services, increasing time spent on your site and potentially improving conversion rates. For example, an insurance company could use a modified version of the Calculator to help customers estimate their premiums.
How can I add more complex mathematical operations to the Calculator template?
To add more complex operations to the Calculator template, you can modify the Python code in main.py
. Here's an example of how to add a power operation:
```python @app.route('/', methods=['GET', 'POST']) def calculator(): result = None if request.method == 'POST': try: num1 = float(request.form['num1']) num2 = float(request.form['num2']) operation = request.form['operation']
# ... existing code ...
elif operation == 'power':
result = num1 num2
except ValueError:
return "Error: Please enter valid numbers!"
return render_template('calculator.html', result=result)
```
Don't forget to add the new operation to the HTML select element in calculator.html
:
html
<select id="operation" name="operation">
<!-- ... existing options ... -->
<option value="power">Power (^)</option>
</select>
How can I implement error handling for invalid inputs in the Calculator template?
The Calculator template already includes basic error handling, but you can enhance it further. Here's an example of how to implement more detailed error handling:
```python @app.route('/', methods=['GET', 'POST']) def calculator(): result = None error = None if request.method == 'POST': try: num1 = float(request.form['num1']) num2 = float(request.form['num2']) operation = request.form['operation']
if operation == 'divide' and num2 == 0:
error = "Error: Division by zero is not allowed!"
else:
# Perform calculation
# ... existing code ...
except ValueError:
error = "Error: Please enter valid numbers!"
return render_template('calculator.html', result=result, error=error)
```
Then, update the calculator.html
to display the error:
```html
{% if error %}
{{ error }}
{% endif %} ```
This approach provides more specific error messages and improves the user experience of the Calculator template.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Calculator App template:
Introduction
This template provides a simple web-based calculator application built with Flask. It allows users to perform basic arithmetic operations (addition, subtraction, multiplication, and division) through a user-friendly interface.
Getting Started
- Click "Start with this Template" to begin using the Calculator App template in Lazy.
Test the App
-
Press the "Test" button to deploy and run the application.
-
Once the deployment is complete, Lazy will provide you with a dedicated server link to access the calculator app.
Using the Calculator App
-
Open the provided server link in your web browser to access the calculator interface.
-
You'll see a simple form with the following elements:
- Two input fields for entering numbers
- A dropdown menu to select the operation
-
A "Calculate" button
-
To use the calculator:
- Enter the first number in the "First Number" field
- Enter the second number in the "Second Number" field
- Select the desired operation from the dropdown menu (Add, Subtract, Multiply, or Divide)
-
Click the "Calculate" button
-
The result of the calculation will be displayed below the form.
Notes
- The calculator handles basic error cases, such as division by zero or invalid input.
- The app uses Flask's built-in development server, which is suitable for testing but not for production use.
This Calculator App template provides a straightforward example of how to create a web-based application using Flask. You can use this as a starting point to build more complex web applications or to learn about handling form submissions and basic error handling in Flask.
Here are 5 key business benefits for this calculator template:
Template Benefits
-
Quick Financial Calculations: Businesses can use this calculator for rapid financial computations like profit margins, tax calculations, or budget adjustments, saving time and reducing errors.
-
Customer-Facing Tool: Companies can embed this calculator on their website to help customers estimate costs, loan payments, or product quantities, enhancing user experience and engagement.
-
Employee Productivity: This tool can boost workplace efficiency by providing a simple, accessible calculator for common calculations, reducing reliance on separate devices or software.
-
Customizable for Specific Industries: The template can be easily modified to include industry-specific calculations, such as construction material estimates or restaurant portion sizing.
-
Training and Education: Businesses can use this interactive calculator as part of employee training programs or customer education initiatives, making complex concepts more tangible and understandable.
Technologies
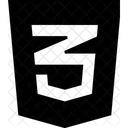
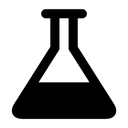
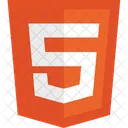
