by moonboy
Basic Telegram Bot with /start and /help commands
from utils import print_setup_instructions
import os
import logging
from telegram import Update
from telegram.ext import Updater, CommandHandler, MessageHandler, Filters, CallbackContext
TELEGRAM_API_TOKEN = os.getenv('TELEGRAM_API_TOKEN')
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
def start(update: Update, context: CallbackContext) -> None:
"""Send a message when the command /start is issued."""
user = update.effective_user
update.message.reply_text(f'Hi {user.first_name}! I am your friendly bot. Use /help to see what I can do.')
def help_command(update: Update, context: CallbackContext) -> None:
"""Send a message when the command /help is issued."""
help_text = """
Here are the commands I understand:
/start - Start the bot
/help - Show this help message
You can also send me any message and I'll echo it back to you!
Frequently Asked Questions
What are some potential business applications for this Basic Telegram Bot template?
The Basic Telegram Bot template can be used for various business applications, such as: - Customer support chatbots - Order tracking and notifications - Appointment scheduling and reminders - News and updates distribution - Simple surveys or feedback collection
How can I customize the bot's responses for my specific business needs?
To customize the Basic Telegram Bot for your business, you can modify the start
, help_command
, and echo
functions in the main.py
file. For example, you could add product information, FAQs, or integrate with your business's API to provide real-time data to users.
Is this Basic Telegram Bot template suitable for handling financial transactions?
While the Basic Telegram Bot template provides a good starting point, it's not recommended for handling financial transactions out of the box. For such sensitive operations, you would need to implement additional security measures, error handling, and potentially integrate with secure payment gateways.
How can I add a new command to the Basic Telegram Bot?
To add a new command to the Basic Telegram Bot, you need to create a new function and add a new CommandHandler in the setup_telegram_bot
function. Here's an example of adding a /price
command:
```python def price_command(update: Update, context: CallbackContext) -> None: update.message.reply_text("Our product costs $9.99!")
def setup_telegram_bot(): # ... existing code ... dispatcher.add_handler(CommandHandler("price", price_command)) # ... rest of the function ... ```
Can I use this Basic Telegram Bot template to send messages to users proactively?
Yes, you can use this template as a base and add functionality to send proactive messages. You'll need to store chat IDs of users who have interacted with your bot and then use the bot.send_message()
method. Here's a simple example:
```python from telegram import Bot
def send_broadcast(message: str): bot = Bot(token=TELEGRAM_API_TOKEN) user_chat_ids = [...] # Get this from your user database for chat_id in user_chat_ids: bot.send_message(chat_id=chat_id, text=message) ```
Remember to comply with Telegram's terms of service and get user consent before sending proactive messages.
Created: | Last Updated:
Here's a step-by-step guide for using the Basic Telegram Bot template with /start and /help commands:
Introduction
This template provides a simple starting point for creating a Telegram bot that responds to /start and /help commands, as well as echoes back any messages sent to it. It's an excellent foundation for building more complex Telegram bots.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Initial Setup
Before you can run your bot, you'll need to set up a Telegram Bot API Token:
- Open Telegram and search for the BotFather (@BotFather).
- Start a chat with BotFather and send the command
/newbot
. - Follow the prompts to create your new bot. Choose a name and username for your bot.
- Once completed, BotFather will provide you with an API token. Copy this token.
- In the Lazy Builder interface, go to the "Env Secrets" tab.
- Find the
TELEGRAM_API_TOKEN
secret and paste your API token as its value.
Test Your Bot
- Click the "Test" button in the Lazy Builder interface to start the deployment process.
- Wait for the deployment to complete. The Lazy CLI will display progress information.
Using Your Bot
Once the bot is running, you'll see a message in the Lazy CLI indicating that the bot is connected and ready to use. It will also provide a link to start chatting with your bot.
- Click the provided link or search for your bot's username in Telegram.
- Start a chat with your bot and try the following commands:
/start
: The bot will greet you with a welcome message./help
: The bot will display a list of available commands.- You can also send any message to the bot, and it will echo it back to you.
Customizing Your Bot
To customize your bot's functionality:
- Open the
main.py
file in the Lazy Builder interface. - Modify the
start
,help_command
, andecho
functions to change how your bot responds to different inputs. - Add new command handlers in the
setup_telegram_bot
function if you want to add more commands.
For example, to add a new /weather
command:
```python def weather(update: Update, context: CallbackContext) -> None: update.message.reply_text("The weather is sunny today!")
In the setup_telegram_bot function, add:
dispatcher.add_handler(CommandHandler("weather", weather)) ```
- After making changes, click the "Test" button again to redeploy your bot with the new functionality.
That's it! You now have a working Telegram bot that you can customize and expand upon. Happy building!
Template Benefits
-
Rapid Customer Support: Implement a quick-response system for customer inquiries, providing instant answers to common questions and routing complex issues to human agents, improving customer satisfaction and reducing support costs.
-
Automated Notifications: Set up a notification system for businesses to send updates, alerts, or reminders to customers or employees, enhancing communication efficiency and ensuring important information reaches the right people promptly.
-
Lead Generation and Qualification: Create an interactive bot that can engage potential customers, collect initial information, and qualify leads before human intervention, streamlining the sales process and improving conversion rates.
-
Internal Communication Tool: Develop a company-wide communication bot for sharing announcements, collecting feedback, or facilitating quick polls, improving internal information flow and employee engagement.
-
Order Management: Implement a bot that can handle basic order inquiries, track shipments, or process simple transactions, reducing the workload on customer service teams and providing 24/7 availability for customers.
Technologies
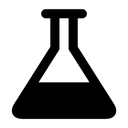
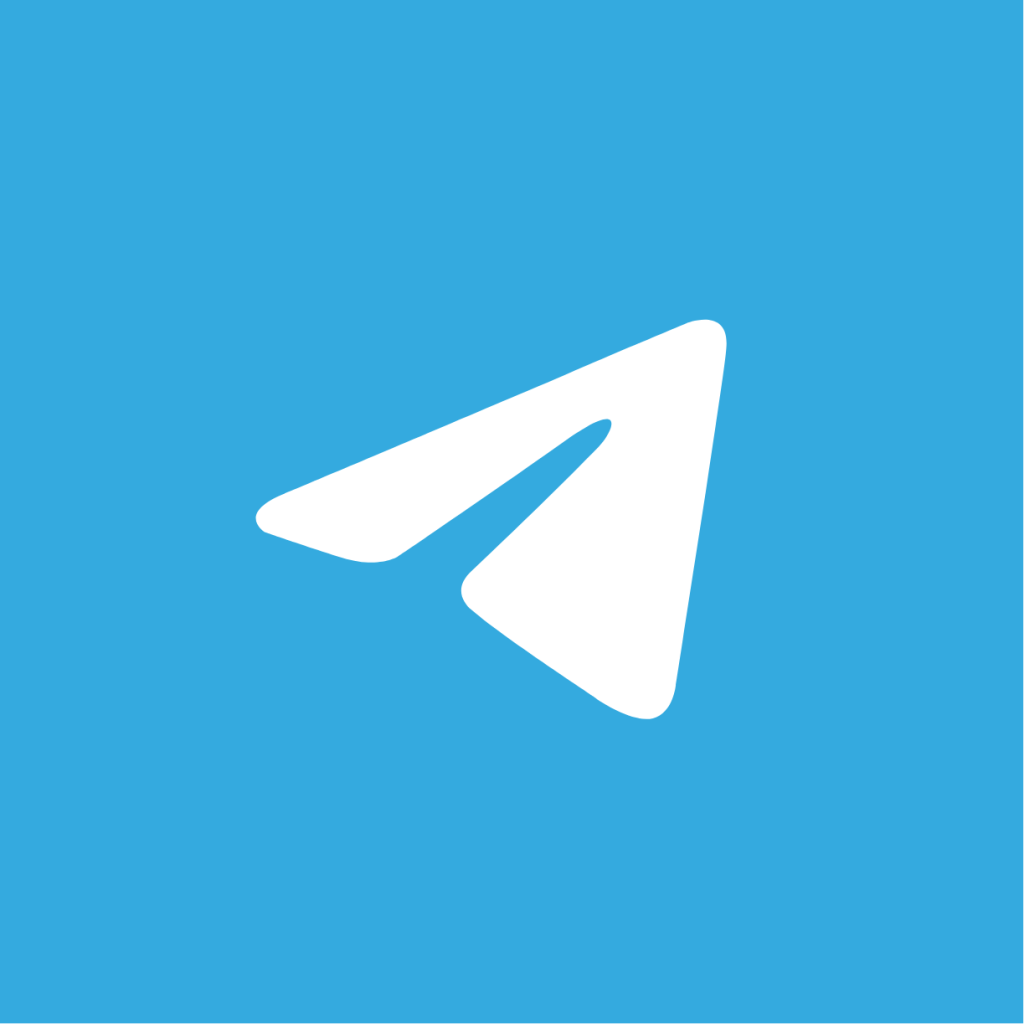
