by davi
Simple Slack Bot
import logging
import os
import re
from slack_bolt import App
from slack_bolt.adapter.socket_mode import SocketModeHandler
from abilities import apply_sqlite_migrations
from abilities import llm_prompt
from models import Base, engine
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Install the Slack app and get xoxb- token in advance
app = App(token=os.environ["SLACK_BOT_TOKEN"])
@app.event("app_mention")
def event_test(body, say, event):
say("Hi I'm the slack bot", thread_ts=event['ts'])
if __name__ == "__main__":
apply_sqlite_migrations(engine, Base, 'migrations')
SocketModeHandler(app, os.environ["SLACK_APP_TOKEN"]).start()
Frequently Asked Questions
What are some potential business applications for this Basic Slack Bot?
The Basic Slack Bot template provides a foundation for various business applications. Some potential use cases include: - Customer support: Enhance the bot to answer frequently asked questions or route inquiries to the appropriate team. - Project management: Develop the bot to provide project updates, deadlines, or task assignments within Slack channels. - Employee onboarding: Customize the bot to guide new hires through company policies and procedures. - Meeting scheduling: Extend the bot's capabilities to help coordinate and schedule team meetings. - Company announcements: Use the bot to disseminate important company-wide information or updates.
How can this Basic Slack Bot improve team productivity?
The Basic Slack Bot can be enhanced to boost team productivity in several ways: - Automate routine tasks: Develop the bot to handle repetitive tasks like data entry or report generation. - Provide quick access to information: Extend the bot to retrieve and share important data or documents on demand. - Facilitate communication: Customize the bot to relay messages between teams or departments. - Monitor project progress: Enhance the bot to track and report on project milestones and deadlines. - Streamline workflows: Develop integrations with other tools to create a more seamless work environment.
What industries could benefit most from implementing this Basic Slack Bot?
The Basic Slack Bot template can be adapted for various industries, including: - Technology: For managing development processes, bug tracking, or deployment notifications. - Healthcare: To assist with patient appointment reminders or medication schedules. - Education: For student support, assignment submissions, or course announcements. - Finance: To provide real-time market updates or automate financial reporting. - Retail: For inventory management, sales reports, or customer service inquiries.
How can I extend the Basic Slack Bot to handle more complex interactions?
To handle more complex interactions, you can enhance the Basic Slack Bot by adding new event listeners and implementing more sophisticated logic. For example, you could add a message handler to process user commands:
```python @app.message(re.compile("^!command (.*)")) def handle_command(say, context): command = context['matches'][0] # Process the command and generate a response response = process_command(command) say(response)
def process_command(command): # Implement your command processing logic here return f"Processed command: {command}" ```
This example adds a message handler that responds to messages starting with "!command" and processes the subsequent text.
How can I integrate a database with the Basic Slack Bot for persistent storage?
The Basic Slack Bot template already includes SQLAlchemy for database integration. To add a new model and use it in your bot, follow these steps:
Created: | Last Updated:
Here's a step-by-step guide on how to use the Basic Slack Bot template:
Introduction
This template provides a simple starting point for creating a Slack bot that responds to mentions. The bot will reply with a greeting message when it's mentioned in a Slack channel.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Initial Setup
Before testing the bot, you need to set up a Slack app and obtain the necessary tokens. Follow these steps:
- Go to the Slack API website (https://api.slack.com/apps) and sign in to your Slack workspace.
- Click "Create New App" and choose "From scratch."
- Give your app a name and select the workspace where you want to install it.
- In the "Add features and functionality" section, click on "Bots."
- Click "Add a Bot User" and configure the bot's display name and username.
- Go to "OAuth & Permissions" in the sidebar.
- Under "Scopes," add the following Bot Token Scopes:
app_mentions:read
chat:write
- Scroll up to "OAuth Tokens for Your Workspace" and click "Install to Workspace."
- After installation, you'll see a "Bot User OAuth Token" starting with
xoxb-
. Copy this token. - Go back to the "Basic Information" page for your app.
- Under "App-Level Tokens," click "Generate Token and Scopes."
- Name the token (e.g., "socket mode"), add the
connections:write
scope, and click "Generate." - Copy the generated App-Level Token starting with
xapp-
.
Now, set up the environment secrets in the Lazy Builder:
- Go to the "Environment Secrets" tab in the Lazy Builder interface.
- Add a new secret named
SLACK_BOT_TOKEN
and paste the Bot User OAuth Token (starting withxoxb-
). - Add another secret named
SLACK_APP_TOKEN
and paste the App-Level Token (starting withxapp-
).
Test
Click the "Test" button in the Lazy Builder interface to start the deployment process.
Using the App
Once the app is deployed:
- Invite the bot to a Slack channel in your workspace.
- Mention the bot in the channel using its name (e.g.,
@YourBotName
). - The bot should respond with "Hi I'm the slack bot" in a thread.
Integrating the App
To fully integrate the bot into your Slack workspace:
- Go back to your Slack App settings (https://api.slack.com/apps).
- Click on your app, then go to "Event Subscriptions" in the sidebar.
- Toggle "Enable Events" to On.
- In the "Subscribe to bot events" section, click "Add Bot User Event" and add the
app_mention
event. - Click "Save Changes" at the bottom of the page.
Your Slack bot is now set up and ready to respond to mentions in your Slack workspace.
Template Benefits
-
Rapid Prototyping: This template provides a quick starting point for businesses to develop and deploy a Slack bot, allowing for fast prototyping and testing of ideas without extensive setup.
-
Enhanced Team Communication: By integrating a custom bot into Slack, businesses can improve internal communication and automate responses to common queries, increasing overall team efficiency.
-
Scalable Foundation: The template includes a database setup with SQLAlchemy, allowing for easy expansion of the bot's capabilities to include data storage and retrieval as the business needs grow.
-
Customizable Automation: Businesses can easily modify the bot's responses and add new functionalities to automate repetitive tasks, freeing up employee time for more valuable work.
-
Improved Customer Service: By adapting this template, businesses can create a customer-facing bot in Slack channels shared with clients, providing instant responses to frequently asked questions and improving customer satisfaction.
Technologies
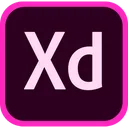
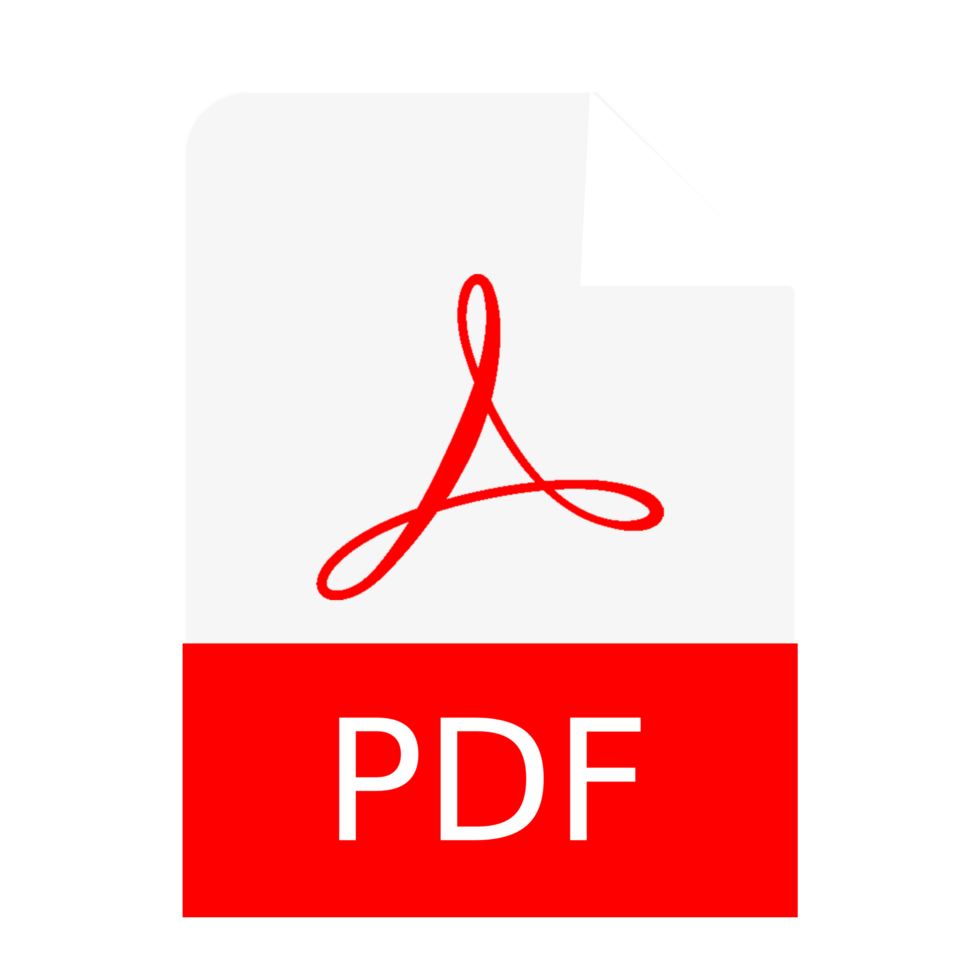

