by Lazy Sloth
Basic Slack Bot
import os
import re
from slack_bolt import App
from slack_bolt.adapter.socket_mode import SocketModeHandler
from abilities import llm_prompt
# Install the Slack app and get xoxb- token in advance
app = App(token=os.environ["SLACK_BOT_TOKEN"])
@app.event("app_mention")
def event_test(body, say, event):
say("Hi I'm the slack bot", thread_ts=event['ts'])
if __name__ == "__main__":
SocketModeHandler(app, os.environ["SLACK_APP_TOKEN"]).start()
Frequently Asked Questions
What are some potential business applications for this Basic Slack Bot?
The Basic Slack Bot template provides a foundation for various business applications. Some potential use cases include: - Customer support: Automate responses to common queries - Project management: Provide status updates or reminders - Employee onboarding: Guide new hires through company processes - Internal communications: Distribute company-wide announcements - Workflow automation: Trigger actions based on specific messages or events
How can this Basic Slack Bot improve team productivity?
The Basic Slack Bot can enhance team productivity by: - Automating routine tasks and queries - Providing instant responses to common questions - Facilitating information sharing across teams - Streamlining communication processes - Reducing the need for manual interventions in repetitive tasks
What industries could benefit most from implementing this Basic Slack Bot?
While the Basic Slack Bot can be adapted for various industries, it could be particularly beneficial for: - Technology companies with distributed teams - Customer service-oriented businesses - Project-based industries like marketing or consulting - Educational institutions for student support - Human resources departments in large corporations
How can I extend the Basic Slack Bot to handle more complex interactions?
To enhance the Basic Slack Bot for more complex interactions, you can:
Can the Basic Slack Bot template be modified to work with other messaging platforms?
While the Basic Slack Bot template is specifically designed for Slack, the core concept can be adapted for other platforms. You'd need to replace the Slack-specific libraries and adjust the event handling. For example, to adapt it for Discord, you might use the discord.py library:
```python import discord from discord.ext import commands
bot = commands.Bot(command_prefix='!')
@bot.event async def on_message(message): if bot.user.mentioned_in(message): await message.channel.send("Hi, I'm the Discord bot!")
bot.run('YOUR_DISCORD_TOKEN') ```
Remember to adjust the authentication and event handling methods according to the chosen platform's API.
Created: | Last Updated:
Introduction to the Basic Slack Bot Template
Welcome to the Basic Slack Bot template! This template provides a simple starting point for creating a Slack bot that responds with a greeting when mentioned in a Slack workspace. Whether you're looking to build a more complex bot or just want to learn the ropes of Slack bot development, this template is a great place to start.
Getting Started with the Template
To begin using this template, click on "Start with this Template" on the Lazy platform. This will set up the template in your Lazy Builder interface, pre-populating the code so you can start customizing your Slack bot right away.
Initial Setup
Before you can test and use your Slack bot, you'll need to set up a couple of environment secrets within the Lazy Builder. These secrets are the Slack Bot Token (`SLACK_BOT_TOKEN`) and the Slack App Token (`SLACK_APP_TOKEN`). Here's how to acquire these tokens:
- Go to the Slack API website and create a new app.
- From the 'OAuth \& Permissions' page, add the necessary bot scopes (like `chat:write`) and install the app to your workspace to get the `SLACK_BOT_TOKEN`.
- For the `SLACK_APP_TOKEN`, you'll need to enable Socket Mode in your Slack app settings and generate an app-level token.
- Once you have both tokens, go to the Environment Secrets tab in the Lazy Builder and add them as `SLACK_BOT_TOKEN` and `SLACK_APP_TOKEN` respectively.
With these tokens set, your Slack bot will be able to authenticate with Slack's servers and respond to events in your workspace.
Test: Pressing the Test Button
After setting up your environment secrets, it's time to test your Slack bot. Press the "Test" button in the Lazy Builder. This will deploy your app and start the bot in the background. You won't need to provide any additional user input at this stage.
Using the Slack Bot
Once your bot is running, you can go to your Slack workspace and mention your bot in any channel or direct message. To do this, type `@YourBotName` followed by any message. Your bot should respond with "Hi I'm the slack bot" in the thread of the mention.
Integrating the Slack Bot
After confirming that your bot responds to mentions, you can integrate it further into your Slack workspace or external tools. For example, you might want to set up more event listeners for different types of Slack events, or you might want to use the bot to interact with other APIs and services.
If you plan to integrate with other APIs, remember to add any necessary tokens or keys as environment secrets in the Lazy Builder, just like you did with the Slack tokens.
That's it! You've successfully set up and tested your Basic Slack Bot using the Lazy template. From here, you can continue to expand on the bot's abilities and customize it to fit your needs.
Here are 5 key business benefits for this template:
Template Benefits
-
Improved Team Communication: This Slack bot can enhance internal communication by providing instant responses to mentions, facilitating quicker information exchange and collaboration among team members.
-
Automation of Routine Tasks: The bot can be easily expanded to automate repetitive tasks, such as answering frequently asked questions or providing status updates, saving time and increasing productivity.
-
Enhanced Customer Support: When adapted for customer-facing channels, this bot can provide immediate responses to customer inquiries, improving response times and customer satisfaction.
-
Scalable Information Dissemination: The bot can be programmed to distribute important announcements or updates across multiple Slack channels simultaneously, ensuring consistent and timely information sharing.
-
Integration Potential: This template serves as a foundation for integrating various business tools and services with Slack, creating a centralized hub for workflow management and data access.
Technologies
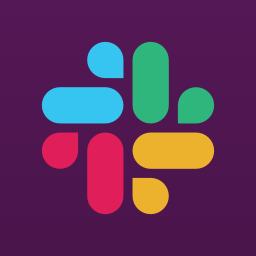