by we
ASCII Flix
from flask import request, Flask, render_template
import logging
from gunicorn.app.base import BaseApplication
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__, template_folder='templates', static_folder='static')
# Temporary storage for demonstration purposes
movies = [] # List to store movie details
# TODO: Define a proper Chat class or structure for handling chat messages
chat_messages = [] # Temporary storage for chat messages
@app.route("/movie/<title>")
def movie(title):
movie = next((m for m in movies if m.title == title), None)
if movie:
return render_template("movie.html", movie=movie)
else:
return "Movie not found", 404
@app.route("/rate_movie", methods=["POST"])
Frequently Asked Questions
What are some potential business applications for ASCII Flix beyond entertainment?
ASCII Flix can be adapted for various business applications: - Educational platforms: Create ASCII animations for teaching complex concepts - Marketing: Develop unique ASCII-based advertisements or promotional content - Data visualization: Present data in an engaging ASCII format for reports or presentations - Internal communication: Use ASCII Flix for creative company announcements or team-building activities
How can ASCII Flix be monetized?
ASCII Flix offers several monetization opportunities: - Subscription model: Offer premium features or exclusive ASCII content - Pay-per-view: Charge for access to special ASCII movie premieres - Advertising: Display text-based ads within the ASCII movies or chat feature - Licensing: Allow other platforms to use ASCII Flix technology for a fee - Custom ASCII content creation: Offer services to create personalized ASCII animations for clients
What are the advantages of using ASCII art for movies compared to traditional video formats?
ASCII Flix's use of ASCII art for movies offers several advantages: - Lower bandwidth requirements, making it accessible in areas with limited internet - Unique, retro aesthetic that can appeal to niche audiences - Easier to create and edit content without expensive video equipment - Potential for interactive elements within the ASCII movies - Compatibility with a wide range of devices, including older hardware
How can I add a new route to display a list of all movies in ASCII Flix?
To add a new route for displaying all movies, you can modify the main.py
file:
python
@app.route("/movies")
def list_movies():
return render_template("movies.html", movies=movies)
Then create a new movies.html
template:
```html
All Movies
-
{% for movie in movies %}
- {{ movie.title }} {% endfor %}
```
This will create a new page listing all available movies in ASCII Flix.
How can I implement a search function in ASCII Flix to find specific movies?
To add a search function, you can create a new route and form in ASCII Flix:
In main.py
, add:
python
@app.route("/search", methods=["GET", "POST"])
def search():
if request.method == "POST":
query = request.form.get("query")
results = [movie for movie in movies if query.lower() in movie.title.lower()]
return render_template("search_results.html", results=results, query=query)
return render_template("search.html")
Create a search.html
template:
```html
Search ASCII Flix
```
And a search_results.html
template to display the results. This implementation allows users to search for movies in ASCII Flix by title.
Created: | Last Updated:
Here's a step-by-step guide for using the ASCII Flix template:
Introduction to ASCII Flix
ASCII Flix is a web application that allows users to watch, rate, and chat about ASCII movies. It provides a platform for viewing ASCII art movies, rating them, and engaging in discussions with other users. The app also supports connecting to a TV for an enhanced viewing experience.
Getting Started
- Click "Start with this Template" to begin using the ASCII Flix template in Lazy.
Test the Application
- Press the "Test" button to deploy the application and launch the Lazy CLI.
Using ASCII Flix
Once the application is deployed, you can access its features through the provided server link. Here's how to use the main functionalities:
Viewing Movies
- Navigate to the home page to see the list of available ASCII movies.
- Click on a movie title to view its details and ASCII art representation.
Rating Movies
- On a movie's page, you'll find a rating form.
- Enter a rating between 1 and 5 stars.
- Submit the rating to provide your feedback on the movie.
Chat Feature
- Access the chat feature through the "/chat" route.
- Enter your name and message in the provided form.
- Submit to send messages and engage in discussions with other users.
Contributing ASCII Movies
For contributors who want to submit ASCII movies:
- Prepare a text file containing the ASCII art movie (2000+ lines of text).
- Contact the administrator at <@200272755520700416> via direct message to submit the ASCII movie file.
Integrating with TV
While the code doesn't provide specific details for TV integration, you may want to explore options for displaying the ASCII Flix interface on a larger screen for a better viewing experience. This could involve:
- Using screen mirroring technologies from your device to a smart TV.
- Setting up a web browser on a TV-connected device to access the ASCII Flix application.
Remember that the exact method for TV integration may vary depending on your specific setup and available equipment.
By following these steps, you'll be able to use and enjoy the ASCII Flix application, watching and interacting with ASCII art movies in a unique and engaging way.
Template Benefits
-
Rapid Prototyping: This template provides a quick starting point for developing a web-based movie streaming and rating platform, allowing businesses to quickly prototype and test new ideas in the entertainment industry.
-
Community Engagement: The chat feature and movie rating system encourage user interaction and community building, which can lead to increased user retention and platform growth.
-
Scalable Architecture: The use of Flask and Gunicorn with multiple workers ensures that the application can handle increased traffic as the user base grows, making it suitable for businesses of various sizes.
-
Customizable Content: The ASCII movie format allows for easy content creation and submission, enabling businesses to quickly expand their library of offerings without the need for complex video production.
-
Cross-Platform Compatibility: The text-based nature of ASCII movies ensures that the content can be viewed on a wide range of devices, from smartphones to smart TVs, broadening the potential user base and market reach.
Technologies
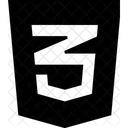
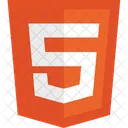
