Open AI-Based Online SQL Query Code Generator
import os
import re
from abilities import llm_prompt
# Define the schema pattern for SQL
SCHEMA_PATTERN = r'CREATE\sTABLE|SELECT\s.*\sFROM\s.*|INSERT\sINTO\s.*|UPDATE\s.*\sSET\s.*|DELETE\sFROM\s.*'
def interpret_schema(schema):
# Use AI to interpret the schema and identify tables and columns
try:
# Call the AI with the schema, asking it to identify tables and columns
interpretation = llm_prompt(
prompt=f"Identify tables, columns, and relationships from this SQL schema: '{schema}'",
model="gpt-4-1106-preview"
)
return interpretation
except Exception as e:
logger.error(f"Error interpreting schema: {e}")
raise
def generate_sql_query(user_request, schema):
# Interpret the schema to find tables and columns
try:
tables_and_columns = interpret_schema(schema)
Frequently Asked Questions
How can the AI-Based Online SQL Query Code Generator benefit businesses without dedicated database administrators?
The AI-Based Online SQL Query Code Generator is an invaluable tool for businesses lacking dedicated database administrators. It allows non-technical staff to interact with databases using natural language, eliminating the need for extensive SQL knowledge. This democratization of data access can lead to more informed decision-making across all levels of an organization, as employees can easily retrieve the information they need without relying on IT departments or external consultants.
What industries could benefit most from using this AI-powered SQL query generator?
The AI-Based Online SQL Query Code Generator can be particularly beneficial for industries that deal with large amounts of data but may not have extensive IT resources. Some examples include:
- Small to medium-sized e-commerce businesses
- Healthcare providers managing patient records
- Educational institutions tracking student data
- Non-profit organizations handling donor information
- Real estate agencies managing property listings
These industries can leverage the tool to quickly generate SQL queries for data analysis, reporting, and decision-making without requiring specialized database expertise.
How does the AI-Based Online SQL Query Code Generator ensure data security and privacy?
The AI-Based Online SQL Query Code Generator prioritizes data security and privacy in several ways:
Can you explain how the `generate_sql_query` function works in the AI-Based Online SQL Query Code Generator?
Certainly! The generate_sql_query
function is a key component of the AI-Based Online SQL Query Code Generator. Here's a breakdown of its functionality:
python
def generate_sql_query(user_request, schema):
try:
tables_and_columns = interpret_schema(schema)
ai_generated_query = llm_prompt(
prompt=f"Generate an SQL query for the following request: '{user_request}' based on this schema: '{schema}'. Output the raw, SQL code without any explanation.",
model="gpt-4-1106-preview"
)
return ai_generated_query
except Exception as e:
logger.error(f"Error generating SQL query: {e}")
raise
This function takes two parameters: user_request
(the natural language query from the user) and schema
(the database schema). It first interprets the schema using the interpret_schema
function, then uses an AI model (GPT-4) to generate an SQL query based on the user's request and the interpreted schema. The function returns the raw SQL query without any additional explanation.
How can I extend the AI-Based Online SQL Query Code Generator to support more complex database operations?
To extend the AI-Based Online SQL Query Code Generator for more complex operations, you could modify the main.py
file. Here's an example of how you might add support for database modifications:
```python def modify_database(user_request, schema): try: modification_query = llm_prompt( prompt=f"Generate an SQL query to modify the database as requested: '{user_request}' based on this schema: '{schema}'. Include necessary safety checks. Output the raw SQL code.", model="gpt-4-1106-preview" ) return modification_query except Exception as e: logger.error(f"Error generating database modification query: {e}") raise
# In the main() function, add: if user_query_request.lower().startswith("modify:"): query = modify_database(user_query_request[7:], schema_text) print("Caution: This query will modify the database.") print(query) confirmation = input("Do you want to proceed? (y/n) ").strip().lower() if confirmation == 'y': # Execute the query (implementation depends on your database setup) print("Database modified.") ```
This extension allows users to request database modifications by prefixing their request with "modify:". It includes a confirmation step to prevent accidental modifications. Remember to implement proper security measures and thoroughly test any modifications to the AI-Based Online SQL Query Code Generator before using in a production environment.
Created: | Last Updated:
Here's a step-by-step guide on how to use the AI-Based Online SQL Query Code Generator template:
Introduction
The AI-Based Online SQL Query Code Generator is a powerful tool that allows you to generate SQL queries using natural language. This template simplifies database management and helps fulfill data analytics requests by interpreting your everyday language and converting it into SQL code.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Press the "Test" button in the Lazy Builder interface.
- This will launch the Lazy CLI, where you'll be prompted to provide the necessary input.
Entering Input
When the Lazy CLI appears, you'll need to provide the following information:
- Enter the SQL code for the database schema when prompted.
- Next, you'll be asked what you want to retrieve from the database. Use everyday language to describe your query.
Using the App
After entering your query request, the app will:
- Generate and display the SQL query based on your input.
- Ask if you want an explanation of the generated code.
- If you type 'y' or 'yes', it will provide an explanation.
- If you type 'n' or 'no', it will skip the explanation.
- Ask if you want to quit or retrieve something else from the database.
- Press 'q' to quit the application.
- Press 'n' to make another query.
Example Usage
Here's an example of how you might interact with the app:
``` Please enter the SQL code for the database schema: CREATE TABLE employees (id INT, name VARCHAR(50), department VARCHAR(50), salary INT);
What do you want to retrieve from the database? (use everyday language) Show me all employees in the IT department
SELECT * FROM employees WHERE department = 'IT';
Would you like an explanation of the code? (y/n) y
This SQL query selects all columns (*) from the 'employees' table where the 'department' column is equal to 'IT'. It will return all information for employees who work in the IT department.
Press 'q' to quit, press 'n' to retrieve something else from the database: n
What do you want to retrieve from the database? (use everyday language) Find the highest paid employee
SELECT name, salary FROM employees ORDER BY salary DESC LIMIT 1;
Would you like an explanation of the code? (y/n) n
Press 'q' to quit, press 'n' to retrieve something else from the database: q ```
This template provides an intuitive way to generate SQL queries without needing to know the exact syntax. It's particularly useful for those who are not SQL experts but need to work with databases regularly.
Template Benefits
-
Simplified Database Querying: This template enables non-technical users to generate SQL queries using natural language, significantly reducing the learning curve for database interactions and improving overall productivity.
-
Improved Data Accessibility: By bridging the gap between everyday language and SQL, this tool democratizes data access within an organization, allowing more employees to retrieve valuable insights without relying on dedicated database administrators.
-
Enhanced Decision-Making: With easier access to database information, business users can make data-driven decisions more quickly and efficiently, leading to improved strategic planning and operational effectiveness.
-
Reduced IT Workload: By empowering end-users to generate their own SQL queries, this template alleviates the burden on IT departments, freeing up technical resources for more complex tasks and reducing bottlenecks in data retrieval processes.
-
Accelerated Data Analysis: The ability to quickly generate and understand SQL queries facilitates faster data analysis, enabling businesses to respond more rapidly to market changes, customer needs, and emerging trends.
Technologies
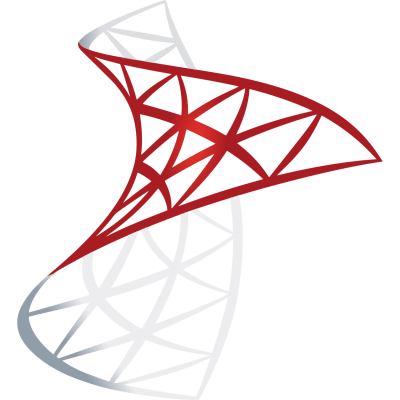