by Muhammad
AI Specific Website Selenium Scraper
from abilities import llm_prompt
import logging
import uvicorn
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
from fastapi.templating import Jinja2Templates
from selenium_utils import SeleniumUtility
URL_TO_FETCH = "https://www.google.com"
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
templates = Jinja2Templates(directory="templates")
from fastapi import Request, Form, Depends
# Route for the index page
# Route for the index page
@app.get("/", response_class=HTMLResponse)
async def read_root(request: Request):
return templates.TemplateResponse("index.html", {"request": request})
Frequently Asked Questions
How can businesses benefit from using the AI Specific Website Scraper?
The AI Specific Website Scraper offers businesses a powerful tool for extracting targeted information from websites. By providing a URL and specifying the type of information needed, companies can quickly gather relevant data for market research, competitor analysis, or content aggregation. This automation saves time and resources compared to manual data collection, allowing businesses to make data-driven decisions more efficiently.
What are some practical applications of this AI Specific Website Scraper in different industries?
The AI Specific Website Scraper has versatile applications across various industries: - E-commerce: Monitoring competitor pricing and product information - Real estate: Gathering property listings and market trends - Finance: Extracting financial news and stock information - Recruitment: Collecting job postings and candidate information - Media: Aggregating news articles and content for analysis
How does the AI Specific Website Scraper handle different types of information extraction?
The AI Specific Website Scraper uses a combination of web scraping techniques and AI-powered content extraction. Users can specify the type of information they need (e.g., text, images) in the info_type
field. The system then uses Selenium to fetch the page content and BeautifulSoup for initial text extraction. Finally, it leverages an LLM (Language Model) to intelligently extract and format the requested information type from the scraped content.
Can you explain how the AI Specific Website Scraper handles URL submissions and content fetching?
Certainly! The AI Specific Website Scraper uses FastAPI to handle URL submissions and content fetching. Here's a code snippet demonstrating the process:
python
@app.post("/fetch", response_class=HTMLResponse)
async def fetch_content(request: Request, url: str = Form(...), info_type: str = Form(...)):
selenium_util = SeleniumUtility()
content = selenium_util.get_page_content(url) or "Failed to retrieve content"
text_content = selenium_util.extract_text_with_bs4(content)
extracted_info = llm_prompt(f"Extract {info_type} from the following content: {text_content}", model="gpt-3.5-turbo-1106", temperature=0.5)
return templates.TemplateResponse("fetch_content.html", {"request": request, "content": extracted_info, "info_type": info_type})
This function receives the URL and info_type from a form submission, uses Selenium to fetch the page content, extracts text using BeautifulSoup, and then uses an LLM to extract the specific information requested.
How can I customize the AI Specific Website Scraper to extract specific types of information?
To customize the AI Specific Website Scraper for specific information types, you can modify the llm_prompt
function call in the fetch_content
route. Here's an example of how you might customize it:
```python def custom_extraction(info_type, text_content): prompts = { "product_info": f"Extract product name, price, and description from: {text_content}", "contact_details": f"Extract phone numbers, email addresses, and physical addresses from: {text_content}", "news_summary": f"Summarize the main news points from: {text_content}" } return llm_prompt(prompts.get(info_type, f"Extract {info_type} from: {text_content}"), model="gpt-3.5-turbo-1106", temperature=0.5)
# In the fetch_content function: extracted_info = custom_extraction(info_type, text_content) ```
This allows you to define specific extraction tasks for different information types, making the AI Specific Website Scraper more versatile for various business needs.
Created: | Last Updated:
Introduction to the AI Specific Website Scraper Template
Welcome to the AI Specific Website Scraper template! This template is designed to help you build an application that can extract specific types of information from any given URL. Whether you need text or any other type of content, this template will guide you through setting up a web scraper that leverages the power of AI to fetch and display the content you need.
The application is built using FastAPI and integrates with a Selenium utility for web scraping, as well as a templating engine to render the content. It's perfect for non-technical builders who want to create software applications without worrying about the complexities of deployment and environment setup.
Getting Started with the Template
To begin using this template, simply click on "Start with this Template" in the Lazy builder interface. This will pre-populate the code in the Lazy Builder, so you won't need to copy or paste any code manually.
Test: Deploying the App
Once you've started with the template, the next step is to deploy the app. Press the "Test" button in the Lazy builder interface. This will initiate the deployment process and launch the Lazy CLI. The Lazy platform handles all the deployment details, so you don't need to install libraries or set up your environment.
Entering Input
After pressing the "Test" button, if the app requires any user input, the Lazy App's CLI interface will prompt you to provide it. This input could be the URL you want to scrape and the type of information you're looking to extract. Follow the prompts in the CLI to enter the necessary information.
Using the App
Once the app is deployed, you will be provided with a dedicated server link to interact with the app. Navigate to this link in your web browser to access the app's interface. Here, you can submit a URL and specify the type of information you want to extract, such as 'text'. After submitting the form, the app will display the extracted content.
If the app uses FastAPI, which it does in this case, you will also be provided with a link to the FastAPI documentation. This can be useful if you want to understand more about how the API works or if you plan to integrate the API into another service or frontend.
Integrating the App
If you wish to integrate the app's functionality into another tool or service, you may need to use the server link provided by Lazy. For example, you could add the API endpoints provided by the app to an external tool that requires API integration. Ensure you follow the specific instructions of the external tool for adding API endpoints.
If the app requires any external setup or integration, such as obtaining API keys or configuring webhooks, make sure to follow the steps provided by the external service to acquire these values. Then, you can enter them as user input when prompted by the Lazy CLI.
By following these steps, you can quickly set up and use the AI Specific Website Scraper template to extract information from websites without any technical hurdles. Enjoy building with Lazy!
Here are 5 key business benefits for this AI Specific Website Scraper template:
Template Benefits
-
Targeted Information Extraction: Businesses can quickly extract specific types of information from any website, saving time and effort in manual data gathering and research.
-
Competitive Intelligence: Companies can easily monitor competitor websites for pricing, product features, or other key information, enabling data-driven strategic decisions.
-
Market Research Automation: Marketing teams can automate the collection of industry trends, consumer opinions, and market data from various online sources to inform their strategies.
-
Content Aggregation: Content creators and publishers can efficiently gather relevant information from multiple sources to produce comprehensive reports or articles.
-
Lead Generation: Sales teams can extract contact information or business details from target company websites, streamlining the lead generation process and improving prospecting efficiency.
Technologies
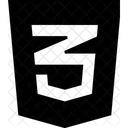
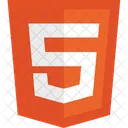

