by moonboy
AI Note Chat
import logging
from flask import Flask, url_for, request, session
from gunicorn.app.base import BaseApplication
from authentication import auth, auth_required
from models.models import db, User
from abilities import apply_sqlite_migrations
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
# Import routes here to avoid circular imports
from routes.routes import routes as routes_blueprint
app.register_blueprint(routes_blueprint)
Frequently Asked Questions
How can AI Note Chat benefit businesses in terms of knowledge management?
AI Note Chat offers significant advantages for businesses in knowledge management. By leveraging AI to create, search, and summarize notes, it streamlines information capture and retrieval. For instance, employees can quickly jot down meeting notes or project ideas, and the AI assistant can help organize and extract key points. This improves knowledge retention and sharing across teams. The chat interface of AI Note Chat also allows for natural language queries, making it easier for staff to find relevant information without needing to remember exact keywords or file locations.
What industries could benefit most from implementing AI Note Chat?
AI Note Chat is particularly valuable for knowledge-intensive industries such as: - Research and Development: Scientists and researchers can use it to document experiments and findings. - Legal Services: Lawyers can organize case notes and quickly retrieve relevant precedents. - Education: Teachers and students can create and manage study notes more effectively. - Healthcare: Medical professionals can maintain patient notes and access medical information rapidly. - Journalism: Reporters can organize interview notes and story ideas efficiently. These industries deal with large amounts of information daily, and AI Note Chat's ability to intelligently organize and retrieve data can significantly boost productivity.
How does AI Note Chat's interactive editing feature enhance user experience?
The interactive editing feature in AI Note Chat significantly enhances user experience by allowing real-time collaboration between the user and the AI. Users can request changes or improvements to their notes using natural language, and the AI can suggest edits or expansions. This feature is particularly useful for refining ideas, expanding on concepts, or restructuring information. For example, a user might say, "Can you reorganize this note into bullet points?" or "Expand on the third paragraph with more details." This interactive approach makes note-taking more dynamic and efficient, helping users create higher-quality content with less effort.
How does AI Note Chat handle note searching and what code is responsible for this functionality?
AI Note Chat uses an intelligent search function that goes beyond simple keyword matching. It employs natural language processing to understand the context of the user's query and searches through both the content and AI-generated keywords of the notes. Here's a snippet of the code responsible for this functionality:
```python def search_relevant_notes(user_id, search_terms): search_conditions = [] for term in search_terms: term_lower = term.lower() word_pattern = f'% {term_lower} %' start_pattern = f'{term_lower} %' end_pattern = f'% {term_lower}' exact_pattern = term_lower
content_conditions = [
Note.content.ilike(pattern) for pattern in
[word_pattern, start_pattern, end_pattern, exact_pattern]
]
keyword_conditions = [
Note.keywords.ilike(f'%{term_lower}%')
]
term_condition = db.or_(*content_conditions, *keyword_conditions)
search_conditions.append(term_condition)
combined_search = db.or_(*search_conditions)
relevant_notes = Note.query.filter(
Note.user_id == user_id,
Note.is_archived == False,
combined_search
).order_by(Note.created_at.desc()).all()
return relevant_notes
```
This function creates complex SQL queries to search for notes based on various patterns and conditions, ensuring that relevant notes are retrieved even if the search terms don't exactly match the note content.
Can you explain how AI Note Chat generates keywords for notes and show the relevant code?
AI Note Chat uses an AI model to generate keywords for each note, which enhances searchability and helps in categorizing the notes. Here's the code snippet responsible for keyword generation:
```python def generate_keywords(content): response_schema = { 'type': 'object', 'properties': { 'keywords': { 'type': 'array', 'items': {'type': 'string'}, 'description': 'List of relevant keywords extracted from the note content' } }, 'required': ['keywords'] }
result = llm(
prompt=f"""Analyze the following note content and extract relevant keywords for search:
{content}
Generate a list of keywords that would be useful for searching this note. Include: - Main topics and themes - Important entities (people, places, organizations) - Key actions and concepts - Relevant synonyms and related terms
Make keywords specific enough to be meaningful but general enough to match similar content. Each keyword can be a single word or a short phrase. Aim for 5-10 keywords that best represent the note's content.""", response_schema=response_schema, image_url=None, model=None, temperature=0.5 )
return ','.join(result['keywords'])
```
This function uses a language model (LLM) to analyze the note content and generate relevant keywords. The AI is prompted to consider various aspects of the content, including main topics, entities, and key concepts. The generated keywords are then joined into a comma-separated string and stored with the note, enhancing the search capabilities of AI Note Chat.
Created: | Last Updated:
Here's a step-by-step guide for using the AI Note Chat template:
Introduction
The AI Note Chat template provides an AI-powered note-taking application with a chat interface. This app allows users to create, search, and summarize personal notes through interactive conversations. Users can also edit notes and engage in discussions about individual notes.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
After starting with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment of the app and launch the Lazy CLI.
Using the App
Once the app is deployed, you'll be provided with a server link to access the application. The app consists of several key features:
-
Chat Interface: Users can interact with the AI assistant to create, search, and manage notes.
-
Note Creation: Users can create new notes by asking the AI to "create a note" or "save this information".
-
Note Search: The AI can search through existing notes based on user queries.
-
Note Editing: Users can edit notes directly from the chat interface.
-
Note Archiving: Notes can be archived or unarchived as needed.
-
AI-Assisted Editing: The app provides AI suggestions for note modifications.
To use these features:
- Open the provided server link in your web browser.
- Log in to the application (authentication is handled automatically by the Lazy platform).
- Start chatting with the AI assistant to manage your notes.
Example interactions:
- To create a note: "Create a note about my daily tasks"
- To search notes: "What notes do I have about books?"
- To edit a note: Use the edit button that appears with note previews
Integrating the App
This application is designed to be used as a standalone web application and does not require integration with external tools or services.
By following these steps, you'll have a fully functional AI-powered note-taking application deployed and ready to use through the Lazy platform.
Template Benefits
-
Enhanced Productivity: This AI-powered note-taking app streamlines the process of creating, organizing, and retrieving information, allowing users to quickly capture ideas and access relevant notes through natural language interactions.
-
Improved Knowledge Management: The intelligent search and summarization features help users efficiently manage and leverage their personal knowledge base, making it easier to recall and utilize information across various projects or tasks.
-
Personalized Learning Assistant: The AI chat interface can act as a study aid or personal tutor, helping users review and understand their notes through interactive conversations, which can enhance learning retention and comprehension.
-
Time-Saving Organization: The automatic keyword generation and archiving features help users keep their notes organized without manual effort, saving time and reducing cognitive load in managing information.
-
Versatile Business Application: This template can be adapted for various professional contexts, such as customer relationship management, project documentation, or employee onboarding, providing an intuitive and intelligent system for capturing and retrieving important information.
Technologies
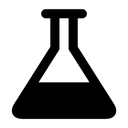

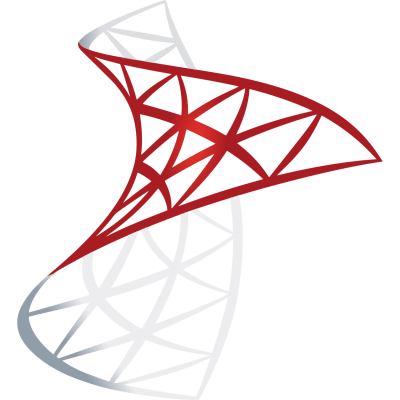