by xeulim
3D Web Game Starter with Three.js
from flask import Flask, render_template, send_from_directory
import os
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/static/<path:path>')
def send_static(path):
return send_from_directory('static', path)
# Create buildings route to serve building models
@app.route('/buildings')
def buildings():
return render_template('buildings.html')
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080)
Frequently Asked Questions
Develop white-label solutions for gaming and education platforms Q3: What gives this template a competitive advantage in the market?
The 3D Web Game Starter stands out because it: - Combines modern web technologies (Three.js, Flask) with battle royale mechanics - Includes a complete building system for virtual environments - Features a modular design that's easily expandable - Provides cross-platform compatibility through web browsers - Includes both first-person and third-person camera modes
Q4: How can I add custom collision detection to the buildings in this template?
A: You can implement building collision detection by adding the following code to the updatePlayerMovement
function in player.js
:
```javascript function checkBuildingCollision(newPosition) { const buildings = scene.children.filter(child => child.userData.type === 'building' );
for (const building of buildings) {
const boundingBox = new THREE.Box3().setFromObject(building);
const playerBox = new THREE.Box3().setFromCenterAndSize(
newPosition,
new THREE.Vector3(0.5, PLAYER_HEIGHT, 0.5)
);
if (boundingBox.intersectsBox(playerBox)) {
return true;
}
}
return false;
} ```
Q5: How do I implement custom weapon models in the template?
A: You can add custom weapon models by extending the inventory system in items.js
. Here's an example:
```javascript inventory.createCustomWeapon = function(name, config) { const weaponGroup = new THREE.Group();
// Create main body
const bodyGeometry = new THREE.BoxGeometry(
config.length,
config.height,
config.width
);
const bodyMaterial = new THREE.MeshStandardMaterial({
color: config.color,
metalness: config.metalness || 0.8,
roughness: config.roughness || 0.3
});
const body = new THREE.Mesh(bodyGeometry, bodyMaterial);
weaponGroup.add(body);
// Add weapon to inventory
this.weaponModels[name] = weaponGroup;
this.weaponAmmo[name] = config.defaultAmmo || 30;
return weaponGroup;
}; ```
Created: | Last Updated:
How to Use the 3D Web Game Starter Template
This template provides a basic 3D web game environment using Three.js with Flask backend. It includes features like player movement, item pickup, health system, and building exploration.
Getting Started
- Click "Start with this Template" to begin
Test the Application
- Click the "Test" button
- Once deployed, you'll receive a server link to access the game interface
Using the Game Interface
The game includes several features:
- WASD keys for movement
- Mouse for looking around
- Space bar for jumping
- C key for holding crouch
- Control key for toggle crouch
- E key for picking up items
- T key for toggling between first and third person view
- Number keys (1-3) for weapon selection
- Q key for bandages
- F key for medkits
- X key for running
- Escape key to pause the game
The interface displays: * FPS counter in top left * Speed display in top right * Health bar in bottom left * Action bars for weapons and items in bottom center
The game environment includes: * Interactive items to pick up (weapons, bandages, medkits) * Various buildings to explore (Administrative Building, Warehouse, Residential Building) * Dynamic zone system that shrinks over time * Airdrop system that periodically spawns supplies
You can also view the building models separately by clicking the "View Buildings" link in the top right corner of the game interface.
This template serves as a foundation for building more complex 3D web games with features like inventory management, combat systems, and environmental interaction.
Template Benefits
- Rapid Game Development Foundation
- Provides a complete foundation for developing 3D web-based games
- Reduces development time with pre-built essential components
-
Includes core gaming features like physics, inventory systems, and player controls
-
Interactive Training Simulations
- Perfect base for creating corporate training simulations
- Can be adapted for safety training, equipment operation, or procedure walkthroughs
-
Supports realistic 3D environments for immersive learning experiences
-
Virtual Property Showcasing
- Excellent starting point for real estate virtual tours
- Building visualization system for architecture firms
-
Interactive 3D product demonstrations for e-commerce
-
Educational Platform Development
- Framework for creating interactive 3D educational content
- Can be used to develop virtual laboratories or historical reconstructions
-
Supports engaging STEM learning experiences through 3D visualization
-
Prototype Testing Environment
- Ideal for testing product designs in a virtual space
- Allows for rapid iteration of user interface concepts
- Provides immediate visual feedback for stakeholder presentations
Technologies
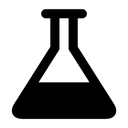
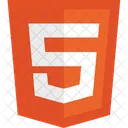
