2Lazy4YouTube
import discord
from discord import app_commands
import os
import yt_dlp
import discord.opus
from discord.ext import commands
import asyncio
intents = discord.Intents.default()
intents.messages = True
intents.message_content = True
bot = commands.Bot(command_prefix='/', intents=intents)
bot_token = os.environ.get('DISCORD_BOT_TOKEN')
@bot.event
async def on_ready():
print(f'Logged in as {bot.user.name}')
await bot.tree.sync()
await bot.change_presence(activity=discord.CustomActivity("Waiting for music to play 🙂"))
async def get_interaction(guild):
class DummyInteraction:
def __init__(self, guild):
Frequently Asked Questions
How can 2Lazy4YouTube benefit my Discord community?
2Lazy4YouTube can greatly enhance your Discord community's experience by providing seamless music streaming from YouTube. It allows users to easily play music in voice channels, creating a shared listening experience. This can be particularly useful for gaming communities, study groups, or social hangouts where background music adds to the atmosphere.
Are there any potential copyright concerns when using 2Lazy4YouTube?
While 2Lazy4YouTube provides a convenient way to stream music from YouTube, it's important to be aware of potential copyright issues. The bot itself doesn't circumvent any of YouTube's policies, but the use of copyrighted material without permission could potentially violate terms of service. It's recommended to use the bot responsibly and primarily for personal or fair use scenarios.
How can I customize 2Lazy4YouTube for my specific Discord server needs?
2Lazy4YouTube is designed to be flexible and customizable. You can modify the bot's behavior by adjusting the code. For example, you could add new commands, change the prefix, or integrate with other APIs. Here's an example of how you might add a new command to display the current song:
```python @bot.tree.command(name='now_playing') async def now_playing_command(interaction: discord.Interaction): """Displays the currently playing song.""" await interaction.response.defer()
if interaction.guild.voice_client and interaction.guild.voice_client.is_playing():
await interaction.followup.send(f"Currently playing: {bot.current_song}")
else:
await interaction.followup.send("No song is currently playing.")
```
What are the technical requirements for running 2Lazy4YouTube?
To run 2Lazy4YouTube, you'll need Python 3.7 or higher installed on your system. The bot also requires several dependencies, which are listed in the requirements.txt
file. These include discord.py
for Discord API interaction, yt-dlp
for YouTube downloading, and FFmpeg
for audio processing. You'll also need to set up a Discord bot token and ensure your bot has the necessary permissions in your Discord server.
How can I implement a queue system in 2Lazy4YouTube?
Adding a queue system to 2Lazy4YouTube would enhance its functionality, allowing users to line up multiple songs. Here's a basic example of how you might implement a queue:
```python from collections import deque
# Add this to your global variables song_queue = deque()
@bot.tree.command(name='add_to_queue') async def add_to_queue_command(interaction: discord.Interaction, url: str): """Adds a song to the queue.""" await interaction.response.defer()
# Add logic here to extract song info from URL
song_info = {"url": url, "title": "Song Title"} # Replace with actual info
song_queue.append(song_info)
await interaction.followup.send(f"Added {song_info['title']} to the queue.")
# Modify your play_command to check the queue when a song finishes def play_next(error): if error: print(f"Player error: {error}") if song_queue: next_song = song_queue.popleft() # Add logic here to play next_song['url']
# Use this callback in your play_command interaction.guild.voice_client.play(source, after=play_next) ```
This implementation adds a basic queue system to 2Lazy4YouTube, allowing users to add songs to a queue and automatically play the next song when one finishes.
Created: | Last Updated:
Introduction to the 2Lazy4YouTube Template
The 2Lazy4YouTube template allows you to create a Discord bot that streams music from YouTube directly into a Discord voice channel. This bot can play audio from YouTube videos or livestreams, adjust the volume, and stop the music when needed.
Clicking Start with this Template
To get started with the 2Lazy4YouTube template, click Start with this Template.
Test
Press the Test button to begin the deployment of the app. The Lazy CLI will handle the deployment process.
Entering Input
After pressing the Test button, you will be prompted to provide the following user input through the Lazy CLI:
- YouTube URL: The URL of the YouTube video or livestream you want to play.
- Volume Percentage: The volume level (0-100) at which you want the audio to play.
Using the App
Once the bot is deployed and running, you can interact with it using the following commands in your Discord server:
- /play_music: Plays audio from a YouTube URL in a voice channel.
-
Parameters:
url
: The YouTube URL of the video or livestream to play.volume_percentage
: The volume to play the audio at (0-100).
-
/change_volume: Changes the volume of the currently playing audio.
-
Parameters:
volume_percentage
: The new volume to set (0-100).
-
/stop_music: Stops the currently playing audio.
Integrating the App
To integrate the bot into your Discord server, follow these steps:
- Create a Discord Bot:
- Go to the Discord Developer Portal.
- Create a new application.
- Navigate to the "Bot" section and click "Add Bot".
-
Under the "TOKEN" section, click "Reset Token" to get your Discord bot token. Copy this token.
-
Set the Bot Token:
- In the Lazy Builder, go to the Environment Secrets tab.
-
Add a new secret with the key
DISCORD_BOT_TOKEN
and paste the copied bot token as the value. -
Invite the Bot to Your Server:
- In the Discord Developer Portal, navigate to the "OAuth2" section.
- Under "OAuth2 URL Generator", select the "bot" scope and the necessary permissions (e.g., "Send Messages", "Connect", "Speak").
-
Copy the generated URL and open it in your browser to invite the bot to your Discord server.
-
Run the Bot:
- Press the Test button in the Lazy Builder to deploy and run the bot.
By following these steps, you will have a fully functional Discord bot that can stream music from YouTube into your Discord server. Enjoy your music! 🎶
Here are 5 key business benefits for this template:
Template Benefits
-
Enhanced Team Collaboration: This Discord bot can be used in business settings to create a shared music experience during virtual meetings or work sessions, potentially boosting team morale and productivity.
-
Streamlined Audio Integration: The template provides a ready-to-use solution for integrating YouTube audio into Discord channels, saving development time and resources for businesses looking to add this functionality.
-
Customizable User Experience: With volume control and playback management features, the bot allows for a tailored audio experience, which can be valuable for businesses hosting online events or presentations.
-
Increased Engagement: By allowing easy access to a wide range of audio content, this bot can help keep users engaged in Discord servers, which could be beneficial for businesses using Discord as a community platform.
-
Potential for Monetization: The template could be adapted for premium features or subscription-based access, providing a potential revenue stream for businesses in the digital content or community management space.
Technologies
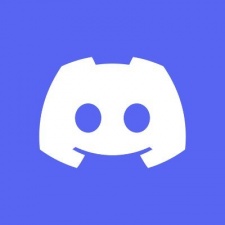