Why Laravel is Ideal for Marketplace Development
Laravel offers a solid foundation for marketplace platforms to grow taking advantage of its expandable architecture and rich ecosystem. Its internal features which are responsible for managing queues, authentication, and database abstraction make it a perfect fit for technical marketplace requirements.
Key Features Of A Laravel Marketplace Template
Through the development of several marketplace platforms, we have discovered important features that serve as the bedrock of a thriving marketplace. Essentially, a successful marketplace will greatly benefit from the robust user role management necessary for differentiating between sellers, buyers, and administrators with clearly stated permissions and capabilities for specific roles.
The product management system has to be adjustable such that it can accommodate several types of listings ranging from physical products to digital goods or services. Support for multiple pricing models, tracking of inventory, and variant management is also included. The product management system is also responsible for handling different commission structures giving room for category or vendor-based fee calculations.
In marketplace environments, special focus should be given to the payment processing system. The system must be able to process split payments while making sure that vendors get their percentage and the platform also retains its share. A holding period for funds is essential in managing disputes and chargebacks efficiently.
A key feature essential for success in the marketplace is the communication feature. This feature incorporates messages between buyers and sellers, announcements on platforms, stock alerts, and automated notifications for order updates. A review and rating system that is properly designed will foster trust and give room for constructive feedback which helps in constant improvement.
Guide To Setting Up Your Laravel Marketplace Project
To set up a marketplace project, there's a need to start out with thorough planning and configuration. The initial and crucial step will be the choice of a perfect database structure that is expandable as your platform grows. A scalable approach makes modification of existing features or the addition of new ones easier without affecting other parts of the system.
In marketplace projects, environment configuration is of utmost importance as different environments require unique settings for email services, third-party integrations, and payment gateways. It is paramount to maintain a detailed environment template with clear documentation for each setting.
Monitoring and analytics should also be factored into the very first setup. This implies that proper logging systems, performance monitoring tools, and error tracking should be incorporated. When user behavior and system performance are properly understood, it will foster decisions that in turn lead to platform improvement.
Core Vendor Management
// app/Models/Vendor.php class Vendor extends Model { protected $fillable = [ 'business_name', 'user_id', 'status', 'commission_rate', 'payout_details', 'verification_status' ]; protected $casts = [ 'payout_details' => 'array', 'commission_rate' => 'float' ]; public function user() { return $this->belongsTo(User::class); } public function products() { return $this->hasMany(Product::class); } public function calculateEarnings($timeframe = null) { $query = $this->products() ->whereHas('orderItems', function($query) { $query->whereHas('order', function($q) { $q->where('status', 'completed'); }); }); if ($timeframe) { $query->where('created_at', '>=', $timeframe); } return $query->sum('total_earnings'); } }
Code Explanation: The Vendor model records seller information like business_name, user_id, and commission_rate, and also links to user accounts and products. The calculate-earnings method helps in processing orders, managing commissions, and ensuring financial transparency. It has been established that for flexibility and expandability in marketplace management, it is pertinent that vendor profiles be separated from user accounts.
Product Management System
// app/Models/Product.php class Product extends Model { protected $fillable = [ 'name', 'slug', 'description', 'price', 'vendor_id', 'category_id', 'stock_quantity', 'status' ]; public function vendor() { return $this->belongsTo(Vendor::class); } public function calculateFinalPrice() { $basePrice = $this->price; $platformFee = $this->vendor->commission_rate; return [ 'selling_price' => $basePrice, 'platform_fee' => $basePrice * ($platformFee / 100), 'vendor_earning' => $basePrice * (1 - $platformFee / 100) ]; } public function updateStock($quantity, $type = 'decrease') { DB::transaction(function() use ($quantity, $type) { if ($type === 'decrease') { if ($this->stock_quantity < $quantity) { throw new InsufficientStockException(); } $this->decrement('stock_quantity', $quantity); } else { $this->increment('stock_quantity', $quantity); } }); } }
Code Explanation: The Product model is responsible for storing basic product information, handling the calculation of prices using platform fees, maintaining vendor relationship management, and ensuring safe stock management through transactions.
Order Processing System
// app/Models/Order.php class Order extends Model { protected $fillable = [ 'user_id', 'total_amount', 'status', 'payment_status', 'shipping_address', 'billing_address' ]; public function items() { return $this->hasMany(OrderItem::class); } public function processOrder() { DB::transaction(function() { foreach ($this->items as $item) { // Update stock $item->product->updateStock($item->quantity); // Calculate commissions $pricing = $item->product->calculateFinalPrice(); $item->update([ 'platform_fee' => $pricing['platform_fee'], 'vendor_earning' => $pricing['vendor_earning'] ]); // Notify vendor event(new OrderReceivedEvent($item)); } $this->update(['status' => 'processing']); }); } }
Code Explanation: The Order processing system is responsible for processing multiple vendor orders, updating stock safely, sending notifications, handling commission calculations and documentation, and ensuring data integrity through database transactions.
Production Tip: In order processing, always incorporate rollback mechanisms and robust error handling. I have been faced with scenarios where payment was successful but commission calculations or stock updates were not successful. The use of Laravel's database and proper exception handling helps to keep the system in a consistent state even when things go wrong. Furthermore, integrating detailed logging for each step in the order process is of immense benefit to customer support and debugging.
Essential Features of a Marketplace Platform
Advanced Security Features
The marketplace platform incorporates detailed security measures using SSL encryption, advanced fraud detection algorithms, and multi-factor authentication. It entails monitoring transactions in real time, detecting suspicious activities, and automatically flagging irregular patterns. Payment processing is safe as the system ensures confidential data transmission and strict adherence to PCI requirements for all financial transactions.
SEO and Marketing Tools
The platform implements detailed SEO optimization with the creation of automated meta tags and structured data markup. It generates SEO-friendly URLs automatically using the product and category names. The ability to incorporate also includes; affiliate program management, social media sharing, and market campaigning tracking. Scheduled promotions and automated email campaigns derived from user behavior are possible as a result of marketing automation tools.
User Reviews and Ratings
A complex and detailed review system avails customers the opportunity to give detailed product analysis with verified purchase badges and pictures attached. The review system includes product quality scores, seller ratings, and delivery performance indicators. The tools used in evaluating reviews do not only ensure that genuine feedback gets to potential buyers but also see to it that the quality of content is maintained.
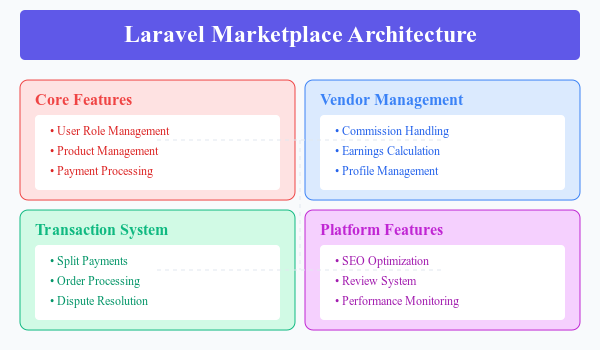
Handling Multi-Vendor Transactions
Payment Splitting
The automated payment distribution system is in charge of technical multi-vendor orders using configurable commission structures. The system is responsible for the instant splitting of payments, and calculation of vendor's earnings and platform fees automatically. Secure payment escrow guarantees buyer protection and maintains vendor cash flow simultaneously using the scheduled distribution of funds.
Order Fulfillment
Synchronized shipping management across several vendors guarantees the effective processing of orders. The system is responsible for tracking partial shipments, merging delivery notifications, and managing different methods of shipping for different vendors. Coordinated order status and automated inventory updates help to maintain precise stock levels across all vendors.
Dispute Resolution
An organized dispute management workflow resolves conflicts between buyers and sellers systematically. The platform makes use of an automated resolution path for frequent issues and provides an escalation route for more technical issues. Clear documentation standards and a deadline for response will guarantee swift and fair resolution.
Performance Optimization for Marketplaces
Scalability
Advanced caching strategies are instrumental in optimizing data queries and decreasing server load during peak traffic. The platform incorporates horizontal expansion using microservices architecture and ensuring independent scaling of key components. Query optimization and database sharding guarantees constant performance even as the volume of data increases.
Load Balancing
In peak periods, the sharing of traffic across several servers guarantees stable performance. Dynamic allocations of resources respond to traffic patterns with failover systems in place to ensure that service is always available. Content delivery networks also improve global access speeds thereby decreasing server load.
Mobile Optimization
Responsive design standards ensure that users have a constant experience across all devices. Touch-friendly interfaces, progressive image loading, and improved mobile checkout flows have been implemented by the platform. Performance monitoring tools track mobile-specific indicators to ensure real-time responses on all devices.
Frequently Asked Questions
How should I structure vendor payouts?
Make use of a flexible payout system that is capable of handling multiple payment frequencies and methods. Determine holding period for prevention of fraud and automated scheduling for regular payouts.
What's the best approach for handling product variations?
Adopt a flexible attribute system that can manage several product types. Make use of JSON columns for dynamic attributes and incorporate a precise validation for each product category.
How can I implement an efficient search system?
Laravel Scout with Algolia or Elasticsearch is effective for scalable search functionality. Incorporate filters and faceted search to enjoy a better user experience.
What's the recommended way to handle marketplace disputes?
Install a staged dispute resolution system with manual and automated processes. Maintain a comprehensive transaction log to foster easy resolution of disputes.
Final Thoughts
In building a thriving marketplace platform, the needs of vendors, customers, and platform administrators must be balanced. The focus should be on creating an expandable and maintainable system that can develop as your marketplace grows.
The success of the marketplace depends largely on the user's trust and experience. Constant monitoring and improvement of essential indicators will help us recognize opportunities for growth and areas that need improvement.