VK Video Downloader
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can businesses benefit from using the VK Video Downloader template?
The VK Video Downloader template offers several benefits for businesses: - Content curation: Companies can easily download and archive relevant VK videos for market research, competitor analysis, or social media content creation. - Marketing material: Businesses can download user-generated content or influencer videos (with permission) for use in marketing campaigns. - Training and internal communications: HR departments can save educational or company-related videos from VK for employee training or internal presentations.
Is the VK Video Downloader template compliant with VK's terms of service?
While the VK Video Downloader template provides the technical functionality to download videos, it's crucial for users to ensure they comply with VK's terms of service and copyright laws. Businesses should: - Only download videos they have the right to use - Obtain permission from content creators when necessary - Use downloaded content in accordance with VK's policies and applicable laws It's recommended to review VK's terms of service and consult with legal counsel before implementing the VK Video Downloader for commercial purposes.
Can the VK Video Downloader template be customized for specific business needs?
Yes, the VK Video Downloader template is highly customizable. Businesses can: - Modify the user interface to match their brand identity - Integrate it into existing web applications or internal tools - Add features like user authentication, download history, or video categorization - Implement additional video processing capabilities, such as format conversion or thumbnail generation
How can I modify the VK Video Downloader template to support multiple video quality options?
To add support for multiple video quality options, you can modify the download_video
function in routes.py
. Here's an example of how you might implement this:
```python @app.route("/download", methods=['POST']) def download_video(): data = request.json video_url = data.get('url') quality = data.get('quality', '720p') # Default to 720p if not specified
if not video_url:
return jsonify({"success": False, "error": "No URL provided"}), 400
try:
# Implement logic to fetch available quality options
quality_options = get_video_quality_options(video_url)
if quality not in quality_options:
return jsonify({"success": False, "error": f"Quality {quality} not available"}), 400
download_url = get_download_url(video_url, quality)
return jsonify({"success": True, "download_url": download_url}), 200
except Exception as e:
logger.error(f"Error processing video URL: {str(e)}")
return jsonify({"success": False, "error": "An error occurred while processing the video URL"}), 500
```
You would also need to implement the get_video_quality_options
and get_download_url
functions to interact with VK's API and retrieve the appropriate download links.
How can I implement a rate limiter in the VK Video Downloader template to prevent abuse?
To implement a rate limiter, you can use the Flask-Limiter extension. First, install it with pip install Flask-Limiter
, then modify your app_init.py
file:
```python from flask_limiter import Limiter from flask_limiter.util import get_remote_address
def create_initialized_flask_app(): app = Flask(name, static_folder='static') # ... existing initialization code ...
limiter = Limiter(
app,
key_func=get_remote_address,
default_limits=["200 per day", "50 per hour"]
)
@app.route("/download", methods=['POST'])
@limiter.limit("10 per minute")
def download_video():
# ... existing download_video code ...
return app
```
This example sets a global limit of 200 requests per day and 50 per hour, with a specific limit of 10 downloads per minute for the /download
endpoint. Adjust these limits as needed for your VK Video Downloader implementation.
Created: | Last Updated:
Here's a step-by-step guide for using the VK Video Downloader template:
Introduction
The VK Video Downloader template provides a web application that allows users to download videos from VK.com by inputting a video link and clicking a download button. The app supports downloading videos in 720p quality.
Getting Started
- Click "Start with this Template" to begin using the VK Video Downloader template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the VK Video Downloader
-
Once the application is deployed, you'll receive a dedicated server link to access the web interface.
-
Open the provided link in your web browser to access the VK Video Downloader interface.
-
In the web interface, you'll see a text input field and a "Download" button.
-
To download a VK video:
- Paste the VK video link into the input field.
-
Click the "Download" button.
-
The application will process the request and initiate the download of the video in 720p quality.
Important Notes
- The current implementation simulates the download process. In a production environment, you would need to implement the actual VK video download logic.
- The application uses a SQLite database to track migrations. No additional database setup is required.
- The app is designed to run on the Lazy platform, so you don't need to worry about local environment setup or dependencies.
By following these steps, you'll have a functional VK Video Downloader web application running on the Lazy platform. Users can easily input VK video links and download videos through the provided interface.
Template Benefits
-
Streamlined User Experience: The template provides a simple, intuitive interface for users to easily input VK video URLs and initiate downloads, enhancing user satisfaction and potentially increasing repeat usage.
-
Mobile-Responsive Design: With separate mobile and desktop header components, the template ensures a seamless experience across various devices, expanding the potential user base and improving accessibility.
-
Scalable Architecture: The use of Flask, Gunicorn, and SQLAlchemy creates a robust, scalable backend structure that can handle increased traffic and future feature expansions, supporting business growth.
-
Easy Maintenance and Updates: The modular structure of the codebase, including separate files for routes, database operations, and app initialization, allows for easier maintenance, debugging, and feature updates, reducing long-term development costs.
-
Data Collection Potential: The implementation of a database with migration support enables the possibility of collecting user data and download statistics, which can be valuable for business intelligence, marketing strategies, and service improvements.
Technologies
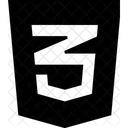
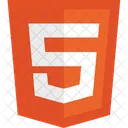