by charlierozco
AI Text Summarization and Rephrasing API
import logging
from fastapi import FastAPI, HTTPException, Request
from fastapi.responses import RedirectResponse, HTMLResponse
from fastapi.staticfiles import StaticFiles
from fastapi.templating import Jinja2Templates
from pydantic import BaseModel
from abilities import llm
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
app.mount("/static", StaticFiles(directory="static"), name="static")
templates = Jinja2Templates(directory="templates")
class TextInput(BaseModel):
text: str
@app.get("/", response_class=HTMLResponse)
async def root(request: Request):
return templates.TemplateResponse("index.html", {"request": request})
@app.post("/rephrase")
async def rephrase_text(input_data: TextInput):
Frequently Asked Questions
What are some potential business applications for this Text Summarization and Rephrasing API?
The Text Summarization and Rephrasing API has numerous business applications across various industries:
How can this API improve productivity in a business setting?
The Text Summarization and Rephrasing API can significantly boost productivity by:
What are the scalability considerations for deploying this API in a high-traffic environment?
When deploying the Text Summarization and Rephrasing API in a high-traffic environment, consider the following:
How can I modify the API to accept longer texts for summarization?
To accept longer texts for summarization, you can modify the TextInput
model and adjust the summarization prompt. Here's an example:
```python from pydantic import BaseModel, Field
class TextInput(BaseModel): text: str = Field(..., max_length=10000) # Increase max_length as needed
@app.post("/summarize") async def summarize_text(input_data: TextInput): try: response = llm( prompt=f"Summarize the following text in 3-5 key points:\n\n{input_data.text}", response_schema={ "type": "object", "properties": { "summary": {"type": "array", "items": {"type": "string"}} }, "required": ["summary"] }, temperature=0.2, model="gpt-4o" ) return {"original_text": input_data.text, "summary": response["summary"]} except Exception as e: logger.error(f"Error in summarizing text: {str(e)}") raise HTTPException(status_code=500, detail="An error occurred while processing your request") ```
This modification allows for longer input texts and returns the summary as a list of key points.
How can I add a new endpoint to the Text Summarization and Rephrasing API for sentiment analysis?
To add a sentiment analysis endpoint, you can create a new route in the FastAPI application. Here's an example:
python
@app.post("/sentiment")
async def analyze_sentiment(input_data: TextInput):
try:
response = llm(
prompt=f"Analyze the sentiment of the following text and categorize it as positive, negative, or neutral:\n\n{input_data.text}",
response_schema={
"type": "object",
"properties": {
"sentiment": {"type": "string", "enum": ["positive", "negative", "neutral"]},
"confidence": {"type": "number", "minimum": 0, "maximum": 1}
},
"required": ["sentiment", "confidence"]
},
temperature=0.2,
model="gpt-4o"
)
return {"original_text": input_data.text, "sentiment": response["sentiment"], "confidence": response["confidence"]}
except Exception as e:
logger.error(f"Error in analyzing sentiment: {str(e)}")
raise HTTPException(status_code=500, detail="An error occurred while processing your request")
This new endpoint will analyze the sentiment of the input text and return the sentiment category along with a confidence score.
Created: | Last Updated:
Here's a step-by-step guide for using the Text Summarization and Rephrasing API template:
Introduction
This template provides a FastAPI application that offers text summarization and rephrasing capabilities using a language model. The app includes a simple HTML interface for users to input text and receive processed results.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and run the application.
-
Once the deployment is complete, you will receive two links through the Lazy CLI:
- A server link to access the HTML interface
- A docs link (e.g.,
http://your-server-link/docs
) to view the FastAPI documentation
Using the Application
-
Open the provided server link in your web browser to access the HTML interface.
-
In the interface, you'll see a text area where you can enter the text you want to process.
-
After entering your text, you have two options:
- Click the "Rephrase" button to get a rephrased version of your text
-
Click the "Summarize" button to get a summary of your text (limited to 200 words)
-
The processed text will appear below the buttons after a short processing time.
API Integration
If you want to integrate this text processing capability into another application, you can use the API endpoints directly:
Rephrase Endpoint
To rephrase text, send a POST request to the /rephrase
endpoint:
``` POST /rephrase Content-Type: application/json
{ "text": "Your text to be rephrased goes here." } ```
Sample response:
json
{
"original_text": "Your text to be rephrased goes here.",
"rephrased_text": "The rephrased version of your text will appear here."
}
Summarize Endpoint
To summarize text, send a POST request to the /summarize
endpoint:
``` POST /summarize Content-Type: application/json
{ "text": "Your text to be summarized goes here. It can be much longer than this example." } ```
Sample response:
json
{
"original_text": "Your text to be summarized goes here. It can be much longer than this example.",
"summary": "A concise summary of your text will appear here, limited to about 200 words."
}
You can use these API endpoints to integrate text rephrasing and summarization capabilities into your own applications or services.
Template Benefits
-
Improved Content Creation Efficiency: This template can significantly speed up content creation processes by automatically rephrasing and summarizing text, saving time for writers, marketers, and content creators.
-
Enhanced Customer Communication: Businesses can use this tool to quickly rephrase or summarize complex information, making it more accessible and understandable for customers, thereby improving communication and customer satisfaction.
-
Streamlined Document Processing: For companies dealing with large volumes of text data, this API can automate the summarization of lengthy documents, reports, or articles, facilitating faster information processing and decision-making.
-
SEO Optimization: The rephrasing functionality can help generate unique content variations for SEO purposes, potentially improving search engine rankings and online visibility for businesses.
-
Multilingual Adaptation: With minor modifications, this template could be extended to support multiple languages, enabling businesses to quickly adapt their content for international markets and expand their global reach.
Technologies
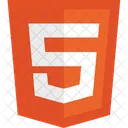