by ekssp121
Telegram Text File Monitor Bot
from utils import print_setup_instructions
import os
import logging
import requests
from telegram import Update, InlineKeyboardButton, InlineKeyboardMarkup
from telegram.ext import Updater, CommandHandler, MessageHandler, Filters, CallbackContext, CallbackQueryHandler
from abilities import upload_file_to_storage, url_for_uploaded_file
TELEGRAM_API_TOKEN = os.getenv('TELEGRAM_API_TOKEN')
TELEGRAM_TARGET_CHANNEL = os.getenv('TELEGRAM_TARGET_CHANNEL')
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
def get_main_menu_keyboard():
"""Create the main menu keyboard."""
keyboard = [
[InlineKeyboardButton("➕ Add to Group", callback_data='join')],
[InlineKeyboardButton("📋 My Groups", callback_data='groups')],
[InlineKeyboardButton("ℹ️ Help", callback_data='help')]
]
return InlineKeyboardMarkup(keyboard)
def get_back_to_menu_keyboard():
Frequently Asked Questions
What are some business applications for the Telegram Text File Monitor Bot?
The Telegram Text File Monitor Bot has several potential business applications:
- Document Management: Companies can use it to automatically collect and centralize text files shared across different group chats.
- Customer Support: Support teams can monitor incoming text files (e.g., logs or error reports) from customer groups and forward them to a dedicated channel for quick review.
- Project Management: Teams can use it to gather and compile text-based project updates or reports shared in various group chats.
- Market Research: Businesses can collect text-based survey responses or feedback shared in community groups.
- Compliance Monitoring: Organizations can use it to track and archive specific text-based communications for regulatory purposes.
How can the Telegram Text File Monitor Bot improve workflow efficiency?
The Telegram Text File Monitor Bot can significantly improve workflow efficiency by:
- Automating the collection and centralization of text files, reducing manual effort.
- Ensuring important information isn't missed by forwarding all relevant files to a designated channel.
- Allowing team members to share information in their preferred groups while still maintaining a centralized repository.
- Providing a simple way to monitor multiple groups without needing to actively participate in each one.
- Enabling quick access to all shared text files in one place, saving time on information retrieval.
Can the Telegram Text File Monitor Bot be customized for specific industries or use cases?
Yes, the Telegram Text File Monitor Bot can be customized for various industries and use cases. For example:
- In healthcare, it could be adapted to monitor and collect anonymized patient data shared as text files.
- For educational institutions, it could gather and compile student submissions or research notes.
- In the legal industry, it could be used to collect and archive case-related text documents shared across different team chats.
- For media organizations, it could monitor and compile news tips or story ideas shared as text files.
Customization would involve modifying the bot's code to handle specific file types, implement additional processing logic, or integrate with industry-specific systems.
How can I modify the Telegram Text File Monitor Bot to handle different file types?
To handle different file types, you can modify the handle_document
function in the main.py
file. Here's an example of how you could extend it to handle both .txt and .csv files:
```python def handle_document(update: Update, context: CallbackContext) -> None: if not TELEGRAM_TARGET_CHANNEL: logger.error("TELEGRAM_TARGET_CHANNEL is not set") return
message = update.message
document = message.document
allowed_extensions = ('.txt', '.csv')
if not document.file_name.lower().endswith(allowed_extensions):
return
try:
file = context.bot.get_file(document.file_id)
response = requests.get(file.file_path)
if response.status_code == 200:
content = response.content.decode('utf-8')
file_type = 'text' if document.file_name.lower().endswith('.txt') else 'CSV'
context.bot.send_message(
chat_id=TELEGRAM_TARGET_CHANNEL,
text=f"New {file_type} file from {message.chat.title}\n\nContent:\n{content}"
)
logger.info(f"Successfully forwarded {file_type} file from {message.chat.title}")
else:
logger.error(f"Failed to download file: {response.status_code}")
except Exception as e:
logger.error(f"Error processing document: {str(e)}")
```
This modification allows the bot to handle both .txt and .csv files, distinguishing between them in the forwarded message.
How can I add a feature to the Telegram Text File Monitor Bot to summarize the content of text files?
To add a summarization feature, you could integrate a text summarization library like sumy
into the bot. Here's an example of how you might modify the handle_document
function to include a summary:
```python from sumy.parsers.plaintext import PlaintextParser from sumy.nlp.tokenizers import Tokenizer from sumy.summarizers.lex_rank import LexRankSummarizer
def summarize_text(text, sentences_count=3): parser = PlaintextParser.from_string(text, Tokenizer("english")) summarizer = LexRankSummarizer() summary = summarizer(parser.document, sentences_count) return " ".join([str(sentence) for sentence in summary])
def handle_document(update: Update, context: CallbackContext) -> None: # ... (existing code)
try:
file = context.bot.get_file(document.file_id)
response = requests.get(file.file_path)
if response.status_code == 200:
content = response.content.decode('utf-8')
summary = summarize_text(content)
context.bot.send_message(
chat_id=TELEGRAM_TARGET_CHANNEL,
text=f"New text file from {message.chat.title}\n\nSummary:\n{summary}\n\nFull Content:\n{content}"
)
logger.info(f"Successfully forwarded and summarized text file from {message.chat.title}")
else:
logger.error(f"Failed to download file: {response.status_code}")
except Exception as e:
logger.error(f"Error processing document: {str(e)}")
```
This modification adds a summary of the text file content to the forwarded message, making it easier for users to quickly understand the main points of longer documents. Remember to add sumy
to your requirements.txt
file if you implement this feature.
Created: | Last Updated:
Here's a step-by-step guide for using the Telegram Text File Monitor Bot template:
Introduction
This template provides a Telegram bot that monitors text files in public groups and forwards their contents to a designated channel. It's perfect for teams or communities that want to centralize and track important text-based information shared across multiple groups.
Getting Started
To begin using this template:
- Click "Start with this Template" in the Lazy Builder interface.
Initial Setup
Before running the bot, you need to set up a few things in Telegram and configure the necessary environment secrets:
- Create a new Telegram bot:
- Open Telegram and search for "@BotFather"
- Start a chat and send the command
/newbot
- Follow the prompts to choose a name and username for your bot
-
BotFather will provide you with an API token. Save this for later.
-
Create a channel for forwarded messages:
- Create a new channel in Telegram
- Add your bot as an administrator to this channel
-
Get the channel's username or ID (e.g., @your_channel or -1001234567890)
-
Set up environment secrets:
- In the Lazy Builder, go to the "Env Secrets" tab
- Add the following secrets:
TELEGRAM_API_TOKEN
: Paste the API token you received from BotFatherTELEGRAM_TARGET_CHANNEL
: Enter the username or ID of your target channel
Test the Bot
Once you've set up the environment secrets:
- Click the "Test" button in the Lazy Builder interface
- Wait for the deployment to complete
Using the Bot
After successful deployment, you'll see a message in the Lazy CLI with a link to your bot. Here's how to use it:
- Click the link or search for your bot's username in Telegram
- Start a chat with the bot and send the
/start
command - Use the menu buttons to navigate the bot's features:
- "Add to Group": Get instructions on how to add the bot to a group
- "My Groups": View a list of groups the bot is monitoring
- "Help": Get more information about the bot's features
To add the bot to a group:
- Make sure your group is public
- Get the group's invite link or username
- In a private chat with the bot, use the command:
/join <group_link_or_username>
For example:/join https://t.me/your_group
or/join @your_group
Once added to a group, the bot will automatically monitor and forward any .txt files sent to that group to your designated channel.
Integrating the Bot
This bot works standalone within Telegram and doesn't require additional integration steps. However, to make the most of it:
- Add the bot to all relevant public groups you want to monitor
- Ensure team members know to share important information as .txt files in these groups
- Use the designated channel to keep track of all forwarded text file contents
By following these steps, you'll have a fully functional Telegram bot that helps centralize and monitor text file contents across multiple groups, streamlining information management for your team or community.
Here are 5 key business benefits for this Telegram Text File Monitor Bot template:
Template Benefits
-
Automated Information Gathering: Businesses can use this bot to automatically collect and centralize text-based information shared across multiple Telegram groups, streamlining data collection processes.
-
Enhanced Communication Monitoring: Organizations can effortlessly track important text file communications across various teams or departments, ensuring critical information is not missed.
-
Improved Compliance and Record-Keeping: The bot's ability to forward text file contents to a designated channel aids in maintaining accurate records for compliance, auditing, or historical reference purposes.
-
Increased Productivity: By automating the process of monitoring and forwarding text files, employees can focus on more value-added tasks, reducing manual effort and increasing overall productivity.
-
Scalable Information Management: As the bot can be easily added to multiple groups, it provides a scalable solution for businesses to manage and monitor text-based information across a growing number of communication channels.
Technologies
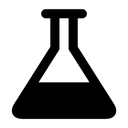
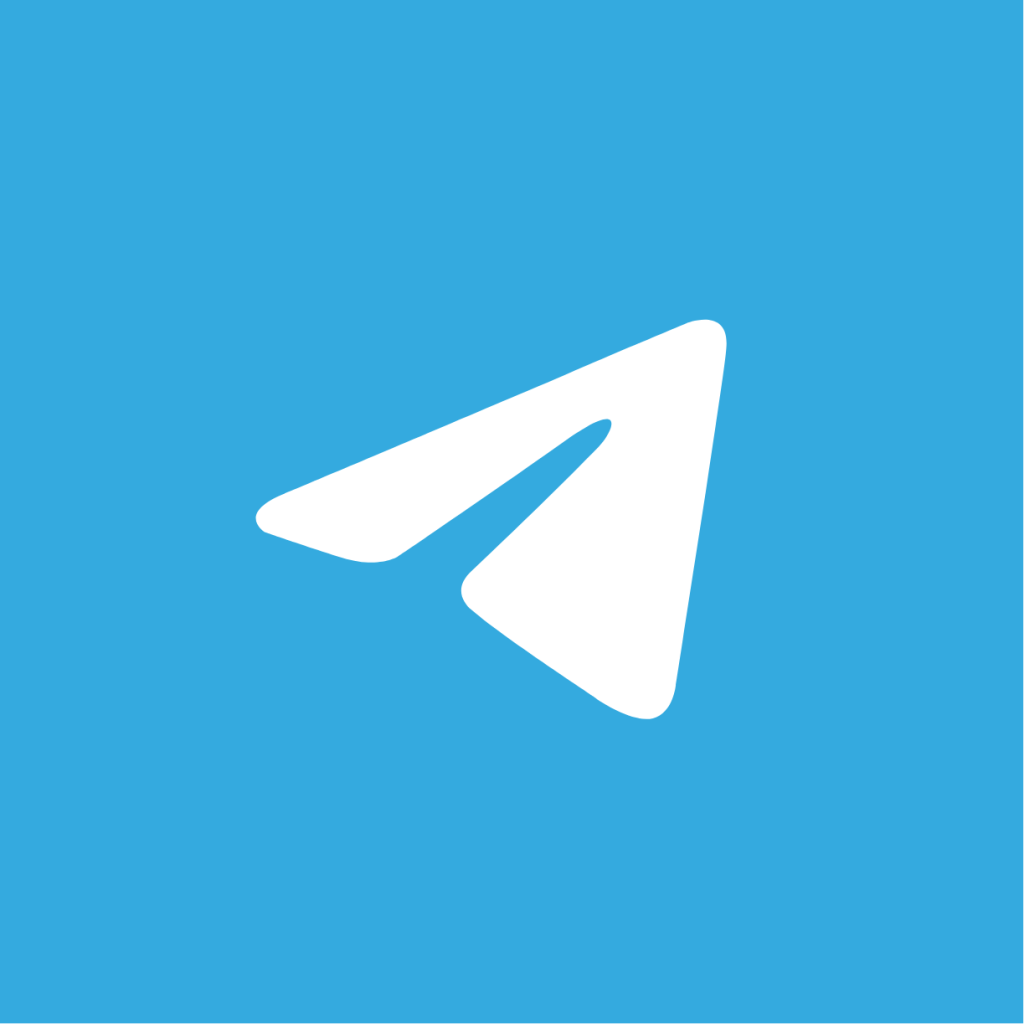

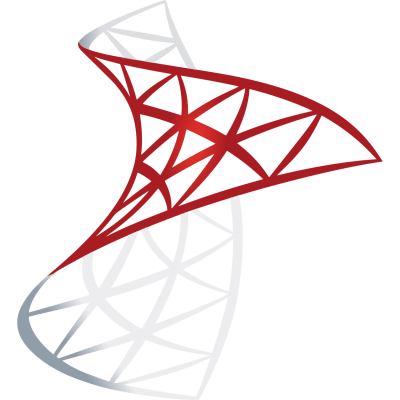