by turnerquan83
SyntaxError Debugger
import os
from flask import Flask, render_template_string
import discord
from threading import Thread
app = Flask(__name__)
# Discord bot setup
intents = discord.Intents.default()
intents.message_content = True
bot = discord.Client(intents=intents)
@bot.event
async def on_ready():
print(f'{bot.user} has connected to Discord!')
@bot.event
async def on_message(message):
if message.author == bot.user:
return
if message.content.startswith('!hello'):
await message.channel.send('Hello!')
Frequently Asked Questions
What is the main purpose of the SyntaxError Debugger template?
The SyntaxError Debugger template is designed to create a Discord bot that can run alongside a web server. Its primary purpose is to provide a simple framework for building a Discord bot with basic functionality while also offering a web interface to monitor the bot's status. This template can be used as a starting point for more complex Discord bots or as a learning tool for developers new to Discord bot development.
How can businesses benefit from using the SyntaxError Debugger template?
Businesses can benefit from the SyntaxError Debugger template in several ways: - It provides a quick way to set up a Discord bot for customer support or community engagement. - The web interface allows for easy monitoring of the bot's status, which is useful for maintaining service reliability. - The template can be extended to include custom commands tailored to the business's specific needs, such as product inquiries or FAQ responses.
Can the SyntaxError Debugger template be used for educational purposes?
Absolutely! The SyntaxError Debugger template is an excellent tool for educational purposes. It can be used in coding bootcamps or computer science courses to teach students about: - Discord bot development - Web server creation with Flask - Multithreading in Python - Integration of different services (Discord API and web server)
How can I add more commands to the Discord bot in the SyntaxError Debugger template?
To add more commands to the Discord bot in the SyntaxError Debugger template, you can extend the on_message
event handler. Here's an example of how to add a new command:
```python @bot.event async def on_message(message): if message.author == bot.user: return
if message.content.startswith('!hello'):
await message.channel.send('Hello!')
# New command
elif message.content.startswith('!ping'):
await message.channel.send('Pong!')
```
This example adds a new !ping
command that responds with "Pong!" when a user types !ping
in the Discord server.
How can I customize the web interface in the SyntaxError Debugger template?
To customize the web interface in the SyntaxError Debugger template, you can modify the HTML string in the home()
function. Here's an example of how to add a custom status message:
python
@app.route('/')
def home():
status_message = "Bot is running smoothly!"
html = f'''
<!DOCTYPE html>
<html>
<head>
<title>Discord Bot Status</title>
<style>
/* ... existing styles ... */
</style>
</head>
<body>
<h1>Discord Bot Status</h1>
<div class="status">
<p>{status_message}</p>
<p>Use !hello in your Discord server to test the bot.</p>
</div>
</body>
</html>
'''
return render_template_string(html)
This example demonstrates how to add a custom status message to the web interface. You can further customize the HTML and CSS to match your desired design and include additional information about the bot's status or functionality.
Created: | Last Updated:
Here's a step-by-step guide on how to use the provided code template for the SyntaxError Debugger app:
Introduction
This template sets up a Discord bot that responds to a simple command and provides a web interface to show the bot's status. The bot will respond to the "!hello" command in Discord, and the web interface will display a simple status message.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Initial Setup
Before testing the app, you need to set up a Discord bot and obtain its token:
- Go to the Discord Developer Portal (https://discord.com/developers/applications).
- Click "New Application" and give it a name.
- Navigate to the "Bot" section in the left sidebar.
- Click "Add Bot" and confirm.
- Under the bot's username, click "Reset Token" and copy the new token.
- In the Lazy Builder, go to the Environment Secrets tab.
- Add a new secret with the key
DISCORD_TOKEN
and paste your bot token as the value.
Test the App
- Click the "Test" button in the Lazy Builder interface to start the deployment process.
Using the App
Once the app is deployed, you can use it in two ways:
- Discord Bot:
- Invite the bot to your Discord server using the OAuth2 URL from the Discord Developer Portal.
- In any channel where the bot has access, type
!hello
. -
The bot should respond with "Hello!".
-
Web Interface:
- The Lazy CLI will provide you with a dedicated server link for the web interface.
- Open this link in your browser to see the Discord Bot Status page.
Integrating the App
This template doesn't require any additional integration steps. The Discord bot and web interface are ready to use once deployed.
To expand the functionality of this template, you can:
- Add more commands to the Discord bot by modifying the
on_message
event handler. - Enhance the web interface by adding more information or interactive elements.
Remember that any changes to the code will require redeploying the app using the "Test" button in the Lazy Builder interface.
Template Benefits
-
Integrated Discord Bot and Web Interface: This template combines a Discord bot with a web application, allowing businesses to manage and monitor their bot's status through a user-friendly web interface. This integration enhances operational efficiency and provides real-time visibility into the bot's functionality.
-
Scalable Communication Platform: By leveraging Discord's infrastructure, businesses can create a scalable communication platform that can handle large numbers of users and messages. This is particularly beneficial for customer support, community management, or internal team collaboration.
-
Customizable Automation: The template provides a foundation for building custom bot commands, enabling businesses to automate repetitive tasks, distribute information, or create interactive experiences tailored to their specific needs.
-
Cost-Effective Development: Using this template as a starting point significantly reduces development time and costs. It provides the basic structure for both the Discord bot and web interface, allowing developers to focus on implementing business-specific features rather than setting up the infrastructure.
-
Multi-Platform Accessibility: The combination of a Discord bot and web interface ensures that the service is accessible through multiple channels. Users can interact with the bot directly in Discord, while administrators can monitor and manage the bot through the web interface, providing flexibility in how the service is used and maintained.
Technologies
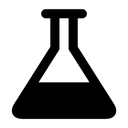
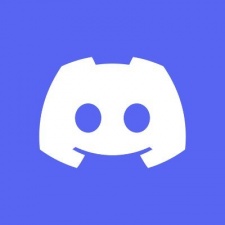