by Lazy Sloth
Create Connected Account with Stripe API
import os
import stripe
# Set the Stripe API key from environment variables
stripe.api_key = os.environ.get('STRIPE_API_KEY')
def create_connected_account(email, country, card_payments, transfers):
try:
account = stripe.Account.create(
type='custom',
country=country,
email=email,
capabilities={
'card_payments': {'requested': card_payments},
'transfers': {'requested': transfers},
}
)
return account
except stripe.error.StripeError as e:
# Handle error
return str(e)
def main():
Create Connected Account with Stripe API
Created: | Last Updated:
Introduction to the Create Connected Account with Stripe API Template
Welcome to the Create Connected Account with Stripe API template! This template is designed to help you seamlessly create custom connected accounts on Stripe, which is essential for platforms that need to process payments on behalf of their users. By using this template, you can quickly set up the backend functionality to register new Stripe connected accounts with capabilities such as card payments and transfers.
Clicking Start with this Template
To begin using this template, simply click on the "Start with this Template" button. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before you can test and use the app, you'll need to set up an environment secret for the Stripe API key. Here's how to do it:
- Log in to your Stripe account and navigate to the API section to find your API keys.
- Copy your Stripe API key. Make sure to use the test key if you're working in a development environment.
- In the Lazy Builder, go to the Environment Secrets tab.
- Create a new secret with the key name
STRIPE_API_KEY
and paste your Stripe API key as the value.
This environment secret will be used by the app to authenticate with the Stripe API.
Test: Pressing the Test Button
Once you have set up the environment secret, you can press the "Test" button. This will begin the deployment of the app and launch the Lazy CLI. If the app requires any user input, you will be prompted to provide it through the CLI interface.
Entering Input: Filling in User Input
After pressing the "Test" button, if the app requires user input, the Lazy CLI will prompt you to enter the necessary information. For this template, you will need to provide:
- Email address of the account holder
- Country of the account
- Whether card payments are enabled (true/false)
- Whether transfers are enabled (true/false)
These inputs correspond to the parameters in the create_connected_account
function within the code template.
Using the App
After providing the required input and successfully creating a connected account, the app will output the result in the CLI. If the account is created, you will see a message with the connected account's ID. If there is an error, the app will display the error message instead.
Integrating the App
Once you have successfully created a connected account, you can integrate this functionality into your platform or service. If you need to use the created Stripe account in your frontend or another service, you can store the account ID and use it in your Stripe API calls to process payments or manage the account.
If you need to make API calls to this service from an external tool, you can use the server link provided by the Lazy builder CLI after pressing the "Test" button. This link will allow you to interact with the app's API. If you're using FastAPI, you will also receive a docs link, which you can use to explore the API's endpoints and their specifications.
Remember to replace the test API key with your live Stripe API key in the environment secrets when you're ready to go live with your application.
That's it! You now have a working backend service to create Stripe connected accounts using the Lazy platform. Happy building!
Technologies
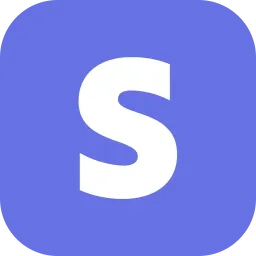
