Simple Multiplayer Telegram game
import logging
from flask import Flask, render_template
from gunicorn.app.base import BaseApplication
from routes import create_app, db
import os
app = create_app()
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
db.drop_all()
db.create_all()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
Created: | Last Updated:
Introduction to the Simple Multiplayer Telegram Game Template
This template provides a simple frontend for a game where users can upvote and downvote the most popular word in their country, learn about the flags of other countries, and view what other people voted for on a leaderboard. The app is built using Flask and integrates with Telegram for user authentication.
Clicking Start with this Template
To get started with this template, click the "Start with this Template" button.
Test
Press the "Test" button to begin the deployment of the app. The Lazy CLI will launch, and you will be prompted for any required user input.
Entering Input
The app requires user input through the CLI for the following fields: - `username`: The word you want to use. - `country`: Your country. - `telegram_user_id`: Your Telegram user ID.
When prompted, provide the necessary input values.
Using the App
Once the app is deployed, you can interact with it through the following pages:
- **Home Page**: This is where users can sign in with their chosen word and country. ```html
Select your country
Sign In
```
- **Upvote Page**: After signing in, users can upvote or downvote their chosen word. ```html Downvote Upvote ```
- **Leaderboard Page**: Users can view the leaderboard to see the most popular words. ```html
| Username | Country | Upvotes | | --- | --- | --- |
```
Integrating the App
To integrate the app with external tools, follow these steps:
1. **Telegram Integration**: Ensure that the Telegram Web App library is included in your HTML. ```html
```
2. **Country Data**: The app fetches country data from the REST Countries API. Ensure that the API endpoint is accessible. ```javascript fetch('https://restcountries.com/v3.1/all') ```
3. **API Endpoints**: The app provides several API endpoints for upvoting, downvoting, and fetching leaderboard updates. Here are some examples: - Increment Upvotes: ```http POST /upvotes/increment/{username}/{country} ``` Sample Request: ```json { "username": "exampleUser", "country": "exampleCountry" } ``` Sample Response: ```json { "upvotes": 10 } ```
- Decrement Upvotes: ```http POST /upvotes/decrement/{username}/{country} ``` Sample Request: ```json { "username": "exampleUser", "country": "exampleCountry" } ``` Sample Response: ```json { "upvotes": 8 } ```
- Get Upvotes: ```http GET /upvotes/{username}/{country} ``` Sample Response: ```json { "upvotes": 10 } ```
- Leaderboard Updates: ```http POST /leaderboard/updates ``` Sample Response: ```json [ { "username": "exampleUser", "country": "exampleCountry", "upvotes": 10, "flag_url": "https://example.com/flag.png" } ] ```
By following these steps, you can successfully deploy and integrate the Simple Multiplayer Telegram Game template using Lazy. Enjoy building your app!
Technologies
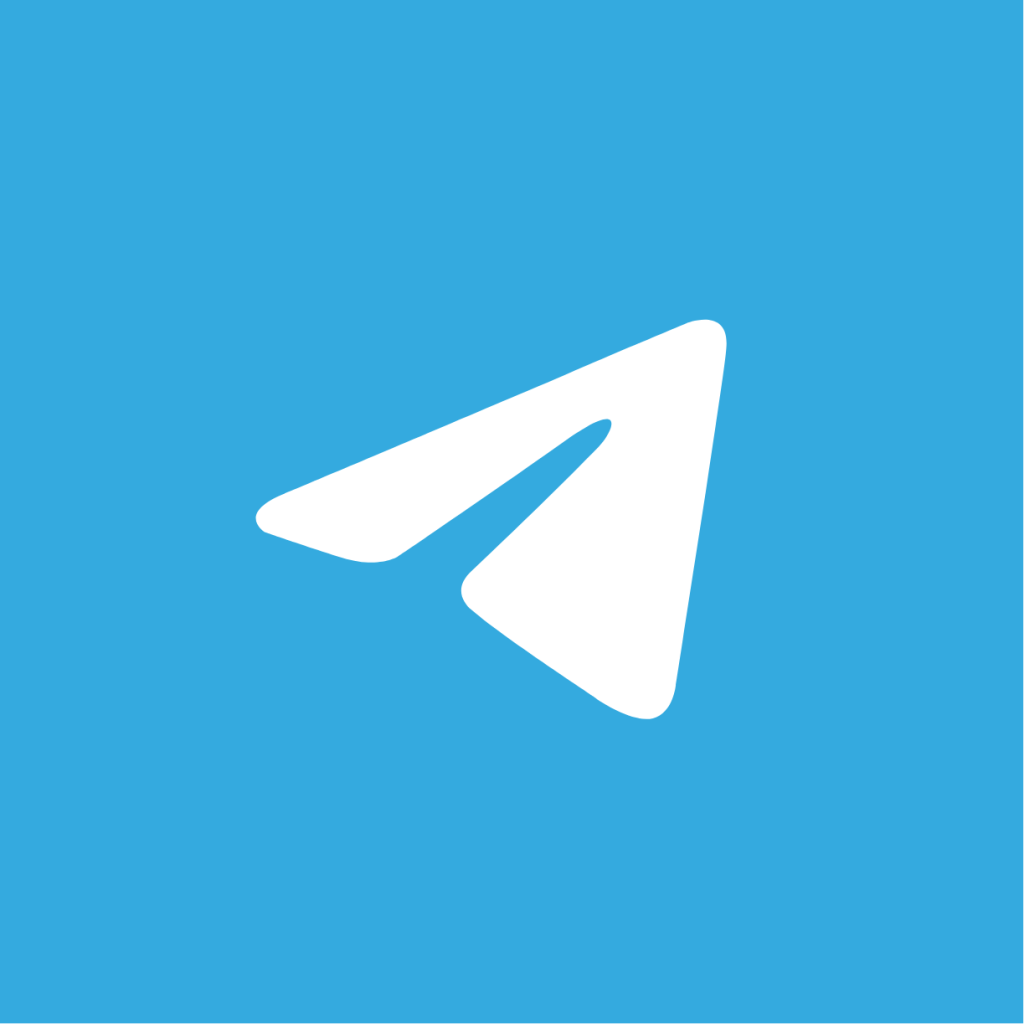

