Reddit Forum Finder
from flask import Flask, render_template_string, redirect, url_for, request, session, flash
from flask_sqlalchemy import SQLAlchemy
from werkzeug.security import generate_password_hash, check_password_hash
import os
from abilities import apply_sqlite_migrations
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
app.config['SECRET_KEY'] = os.urandom(24)
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
password = db.Column(db.String(200), nullable=False)
@app.route('/')
def home():
html = '''
<!DOCTYPE html>
<html lang="en">
<head>
Frequently Asked Questions
How can businesses leverage the Reddit Forum Finder to enhance their marketing strategies?
The Reddit Forum Finder can be a powerful tool for businesses looking to expand their online presence. By using this app, companies can easily identify relevant subreddits where their target audience is active. This allows for more targeted content marketing, community engagement, and even customer support. For example, a tech startup could use the Reddit Forum Finder to discover niche subreddits related to their product, enabling them to participate in discussions, share valuable insights, and subtly promote their brand.
What are the potential applications of the Reddit Forum Finder in academic research?
The Reddit Forum Finder can be invaluable for academic researchers studying online communities, social behavior, or specific topics. Researchers can use this tool to quickly identify relevant subreddits for data collection, sentiment analysis, or participant recruitment. For instance, a sociologist studying the impact of technology on society could use the Reddit Forum Finder to locate subreddits discussing emerging technologies and their societal implications, providing a rich source of qualitative data for their research.
How can the Reddit Forum Finder be monetized as a standalone product?
The Reddit Forum Finder could be monetized through several strategies:
How can I modify the Reddit Forum Finder to include additional information about each subreddit in the search results?
To include additional information in the search results, you can modify the fetch_subreddits
function in the main.py file. For example, to add the creation date of each subreddit, you can update the function as follows:
python
def fetch_subreddits(query):
# ... existing code ...
subreddits = [
{
'name': item['data']['display_name'],
'title': item['data']['title'],
'description': item['data']['public_description'],
'subscribers': item['data']['subscribers'],
'url': f"https://www.reddit.com{item['data']['url']}",
'created_utc': time.strftime('%Y-%m-%d', time.localtime(item['data']['created_utc']))
}
for item in data['data']['children']
]
# ... rest of the function ...
Then, update the HTML template in the dashboard
route to display this new information:
```html
```
This modification will add the creation date to each subreddit card in the search results of the Reddit Forum Finder.
How can I implement user-specific search history in the Reddit Forum Finder?
To implement user-specific search history, you'll need to create a new database model for search history and modify the dashboard route. Here's how you can do it:
First, add a new model in main.py
:
```python class SearchHistory(db.Model): id = db.Column(db.Integer, primary_key=True) user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False) query = db.Column(db.String(200), nullable=False) timestamp = db.Column(db.DateTime, default=datetime.utcnow)
user = db.relationship('User', backref=db.backref('searches', lazy=True))
```
Then, modify the dashboard
route to save searches and display history:
```python @app.route('/dashboard', methods=['GET', 'POST']) def dashboard(): if 'user_id' not in session: return redirect(url_for('login')) user = db.session.get(User, session['user_id']) search_results = None if request.method == 'POST': search_query = request.form['search_query'] search_results = fetch_subreddits(search_query)
# Save search to history
new_search = SearchHistory(user_id=user.id, query=search_query)
db.session.add(new_search)
db.session.commit()
# Fetch user's search history
search_history = SearchHistory.query.filter_by(user_id=user.id).order_by(SearchHistory.timestamp.desc()).limit(5).all()
# Add search history to the HTML template context
return render_template_string(html, user=user, search_results=search_results, search_history=search_history)
```
Finally, update the HTML template to display the search history:
```html
Recent Searches
-
{% for search in search_history %}
- {{ search.query }} - {{ search.timestamp.strftime('%Y-%m-%d %H:%M') }} {% endfor %}
```
These modifications will add user-specific search history functionality to the Reddit Forum Finder, enhancing its usability and personalization.
Created: | Last Updated:
Here's a step-by-step guide for using the Reddit Forum Finder template:
Introduction
The Reddit Forum Finder is a web application that allows users to search for relevant subreddits based on their interests. It features user authentication, a dashboard for searching subreddits, and an admin panel for managing users and content.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Setting Up Environment Variables
This template requires two environment variables to be set up:
- Navigate to the Environment Secrets tab in the Lazy Builder.
- Add the following secrets:
Client ID
: Your Reddit API client IDClient Secret
: Your Reddit API client secret
To obtain these credentials:
- Go to https://www.reddit.com/prefs/apps
- Click "Create App" or "Create Another App"
- Fill in the details:
- Name: Choose a name for your app
- App type: Select "Script"
- Description: Optional
- About URL: Optional
- Redirect URI: Use
http://localhost:8080
- Click "Create app"
- You'll see your client ID under the app name, and your client secret will be listed as "secret"
Testing the Application
Once you've set up the environment variables:
- Click the "Test" button in the Lazy Builder interface.
- The application will start deploying, and you'll see the progress in the Lazy CLI.
Using the Application
After successful deployment, you'll receive a dedicated server link to access the application. The application includes the following features:
- Home page with options to log in or sign up
- User authentication (login and signup)
- Dashboard for searching subreddits
- Admin center for user management and content moderation
To use the application:
- Open the provided server link in your web browser.
- Create a new account or log in if you already have one.
- Once logged in, you'll be directed to the dashboard.
- Use the search bar to find subreddits based on your interests.
- View the search results, including subreddit names, descriptions, and subscriber counts.
- Click on the "Visit Subreddit" link to open the subreddit in a new tab.
The admin center can be accessed through the settings menu on the dashboard. It provides options for user management, content moderation, and system settings.
By following these steps, you'll have a fully functional Reddit Forum Finder application up and running, allowing users to easily discover relevant subreddits based on their interests.
Here are 5 key business benefits for this Reddit Forum Finder template:
Template Benefits
-
Enhanced User Engagement: By providing an easy way for users to discover relevant subreddits, this app can increase user engagement and time spent on the platform, potentially leading to higher ad revenue or subscription conversions.
-
Targeted Marketing Opportunities: Businesses can use this tool to identify niche communities related to their products or services, allowing for more targeted and effective marketing campaigns on Reddit.
-
Market Research Tool: Companies can leverage this app to gain insights into consumer trends, opinions, and discussions across various subreddits, informing product development and marketing strategies.
-
Community Building: The template provides a foundation for creating a centralized hub where users can discover and join communities aligned with their interests, fostering stronger online connections and brand loyalty.
-
Scalable Admin Functionality: With built-in user management and content moderation features, this template offers a scalable solution for businesses looking to create and manage their own forum-based platforms or community sites.
Technologies
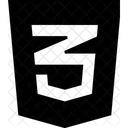
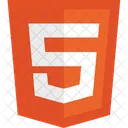