by gallardop622
Read & Sound: Interactive EPUB and PDF Narrator
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are the main business applications for the Read & Sound: Interactive EPUB and PDF Narrator?
Read & Sound: Interactive EPUB and PDF Narrator has several key business applications:
How can Read & Sound: Interactive EPUB and PDF Narrator improve user engagement?
Read & Sound enhances user engagement in several ways:
What are the potential revenue streams for a business implementing Read & Sound?
Businesses implementing Read & Sound can explore various revenue streams:
How can I implement file upload functionality in Read & Sound?
File upload functionality is already implemented in the Read & Sound template. Here's a breakdown of the relevant code:
In routes.py
, there's an upload route:
python
@app.route("/upload", methods=['POST'])
def upload_file():
if 'file' not in request.files:
return redirect(url_for('home_route'))
file = request.files['file']
if file.filename == '' or not allowed_file(file.filename):
return redirect(url_for('home_route'))
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
file.save(file_path)
# TODO: Process the file and extract content
return redirect(url_for('home_route'))
This route handles file uploads, checks if the file is allowed, and saves it to the server. To enhance this for Read & Sound, you'd need to add file processing logic to extract content and prepare it for narration and sound effects.
How can I add audio playback functionality to Read & Sound?
To add audio playback functionality to Read & Sound, you'll need to implement it in the frontend JavaScript. Here's a basic example of how you might start:
In reader.js
, you could add:
```javascript let audioContext; let audioSource;
function initAudio() { audioContext = new (window.AudioContext || window.webkitAudioContext)(); }
function playAudio(audioBuffer) { if (audioSource) { audioSource.stop(); } audioSource = audioContext.createBufferSource(); audioSource.buffer = audioBuffer; audioSource.connect(audioContext.destination); audioSource.start(); }
function loadAndPlayAudio(url) { fetch(url) .then(response => response.arrayBuffer()) .then(arrayBuffer => audioContext.decodeAudioData(arrayBuffer)) .then(audioBuffer => playAudio(audioBuffer)) .catch(error => console.error('Error:', error)); }
// Initialize audio when the page loads document.addEventListener('DOMContentLoaded', initAudio); ```
This sets up basic audio functionality. You'd need to call loadAndPlayAudio
with the URL of your narration audio files when appropriate in your Read & Sound application. Remember to also implement controls for play, pause, and stop functionality to give users full control over the audio playback.
Created: | Last Updated:
Here's a step-by-step guide for using the Read & Sound: Interactive EPUB and PDF Narrator template:
Introduction
The Read & Sound: Interactive EPUB and PDF Narrator template provides an interactive EPUB and PDF reader with audio narration and sound effects based on text content. This template allows you to create a web application where users can upload EPUB or PDF files, read them, and potentially enjoy audio narration and sound effects.
Getting Started
- Click "Start with this Template" to begin using the Read & Sound template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and run the application.
-
Once the deployment is complete, you will receive a dedicated server link to access the web application.
Using the Application
-
Open the provided server link in your web browser to access the Read & Sound application.
-
You will see a welcome page with an upload form for EPUB or PDF files.
-
To use the application:
- Click the "Choose File" button to select an EPUB or PDF file from your device.
-
Click the "Upload" button to submit the file.
-
After uploading, the application will process the file and display its content in the reader section.
Next Steps for Enhancement
While the current template provides a basic structure for file upload and display, there are several areas where you can enhance the application:
- Implement file reading functionality in the
reader.js
file to parse and display EPUB or PDF content. - Add audio narration features by integrating a text-to-speech service.
- Incorporate sound effects based on the content of the text.
- Enhance the user interface with more interactive elements and styling.
Note on File Processing
The current implementation saves the uploaded file but does not process its content. To fully realize the application's potential, you'll need to implement file parsing and content extraction in the upload_file
route in routes.py
.
By following these steps, you'll have a basic web application for uploading EPUB and PDF files. To achieve the full functionality of an interactive reader with audio narration and sound effects, you'll need to expand on this template by implementing the additional features mentioned above.
Template Benefits
-
Enhanced Accessibility: This template provides an audio narration feature for EPUB and PDF files, making books and documents more accessible to visually impaired users or those who prefer auditory learning.
-
Improved User Engagement: By incorporating sound effects based on text content, the template creates a more immersive reading experience, potentially increasing user engagement and retention of information.
-
Multi-Platform Compatibility: The responsive design ensures the application works seamlessly across desktop and mobile devices, expanding the potential user base and improving accessibility.
-
Scalable Architecture: The use of Flask, SQLAlchemy, and a modular structure allows for easy scaling and addition of new features, making it adaptable to growing business needs.
-
Educational Tool: This template can be particularly valuable in educational settings, helping students with different learning styles engage with textbooks, study materials, and literature in a more interactive way.
Technologies
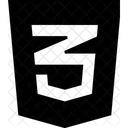
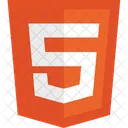
