by sujal.singh
Online video Converter
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
from flask import request, jsonify, send_file
import yt_dlp
import os
import re
from urllib.parse import urlparse, parse_qs
from youtube_transcript_api import YouTubeTranscriptApi
from fpdf import FPDF
import traceback
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
from urllib.parse import urlparse
@app.route('/get_qualities', methods=['POST'])
def get_qualities():
url = request.json.get('url')
Frequently Asked Questions
What are the main features of the Online Video Converter app?
The Online Video Converter app offers several key features: - Supports multiple video sources (YouTube, Facebook, Instagram, etc.) - Converts videos to MP4, MP3, and AVI formats - Provides video quality selection options - Offers a transcription feature that generates a PDF transcript - Includes an auto-download feature when a valid YouTube URL is pasted
How can businesses benefit from integrating this Online Video Converter into their workflow?
Businesses can benefit from the Online Video Converter in several ways: - Content creators can easily download and repurpose video content from various platforms - Marketing teams can extract audio from videos for podcast creation - Training departments can generate transcripts of video content for accessibility and documentation - Social media managers can download and edit content for cross-platform sharing
What are the potential monetization strategies for the Online Video Converter app?
There are several potential monetization strategies: - Freemium model: Offer basic conversions for free, with premium features like batch processing or higher quality outputs - Subscription service: Provide unlimited conversions for a monthly fee - API access: Allow developers to integrate the conversion functionality into their own applications for a fee - White-label solution: Offer a customizable version of the app for businesses to use under their own branding
How does the Online Video Converter handle different video qualities?
The Online Video Converter dynamically fetches available video qualities using the yt_dlp
library. Here's a code snippet demonstrating this functionality:
```python @app.route('/get_qualities', methods=['POST']) def get_qualities(): url = request.json.get('url') if not url: return jsonify({'error': 'No URL provided'}), 400
try:
with yt_dlp.YoutubeDL() as ydl:
info = ydl.extract_info(url, download=False)
formats = info['formats']
available_qualities = []
for f in formats:
if f.get('height'):
quality = f"{f['height']}p"
file_size = f.get('filesize_approx', 0) / (1024 * 1024) # Convert to MB
available_qualities.append({'quality': quality, 'size': f"{file_size:.2f} MB"})
available_qualities = sorted(available_qualities, key=lambda x: int(x['quality'][:-1]), reverse=True)
return jsonify({'qualities': available_qualities})
except Exception as e:
logger.error(f"Error in get_qualities: {str(e)}")
return jsonify({'error': f'Failed to fetch video info: {str(e)}'}), 500
```
This function retrieves available video qualities and their approximate file sizes, allowing users to choose their preferred quality.
Can you explain how the transcription feature works in the Online Video Converter?
The transcription feature uses the youtube_transcript_api
library to fetch the transcript and then generates a PDF using the fpdf
library. Here's a code snippet demonstrating this functionality:
```python @app.route('/transcribe', methods=['POST']) def transcribe_youtube(): url = request.json.get('url') if not url: return jsonify({'error': 'No URL provided'}), 400
video_id = extract_video_id(url)
if not video_id:
return jsonify({'error': 'Could not extract video ID'}), 400
try:
transcript = YouTubeTranscriptApi.get_transcript(video_id)
pdf_filename = generate_pdf(transcript, video_id)
return send_file(pdf_filename, as_attachment=True, download_name=f"{video_id}.pdf")
except Exception as e:
logger.error(f"Error transcribing video: {str(e)}", exc_info=True)
return jsonify({'error': f'Failed to transcribe video: {str(e)}'}), 500
```
This function extracts the video ID, fetches the transcript, generates a PDF, and sends it as a downloadable file to the user. The Online Video Converter's transcription feature can be particularly useful for content creators, researchers, and accessibility purposes.
Created: | Last Updated:
Here's a step-by-step guide for using the Online Video Converter App template:
Introduction
This template provides an Online Video Converter App that allows users to input a URL (from platforms like YouTube, Facebook, Instagram, and many more) and download the video as an MP4, MP3, or AVI file. It also offers a transcription feature to generate a PDF transcript of the video content.
Getting Started
-
Click "Start with this Template" to begin using the Online Video Converter App template in Lazy.
-
Press the "Test" button to deploy the app and launch the Lazy CLI.
Using the App
Once the app is deployed, you'll be provided with a server link to access the web interface. Follow these steps to use the app:
-
Open the provided server link in your web browser.
-
You'll see a simple interface with the following elements:
- A textarea to paste video URLs
- A dropdown to select the output format (MP4, MP3, AVI, or Transcription)
- A quality selection dropdown
-
A "Convert" button
-
Paste one or more video URLs into the textarea, one per line.
-
Select the desired output format from the dropdown menu:
- MP4: Video file
- MP3: Audio file
- AVI: Video file
-
Transcription: PDF transcript of the video
-
If you selected MP4 or AVI, choose the desired quality from the quality dropdown. This option will be disabled for MP3 and Transcription formats.
-
Click the "Convert" button to start the conversion process.
-
Wait for the conversion to complete. The app will display a "Processing... Please wait." message during this time.
-
Once the conversion is finished, the file will automatically download to your device.
Additional Features
-
Auto-Download on Link Copy: If you copy a YouTube URL to your clipboard and paste it anywhere on the page, the app will detect it and ask if you want to start the download process immediately.
-
Dynamic Quality Options: When you paste a YouTube URL, the app will fetch available quality options for that specific video and populate the quality dropdown accordingly.
Integration
This app is designed to be used as a standalone web application. There's no need for additional integration steps with external services. Users can simply access the provided server link to use the Online Video Converter App directly in their web browser.
By following these steps, you'll have a fully functional Online Video Converter App that allows users to easily download and convert videos from various platforms.
Template Benefits
-
Versatile Media Conversion: This template offers a powerful tool for converting online videos from various platforms into different formats (MP4, MP3, AVI), catering to diverse user needs and preferences.
-
Transcription Service: The ability to generate PDF transcripts from YouTube videos adds significant value, making it useful for accessibility, content analysis, and educational purposes.
-
User-Friendly Interface: With a responsive design and intuitive controls, the template provides a seamless experience across devices, potentially increasing user engagement and retention.
-
Scalable Architecture: Built with Flask and Gunicorn, the application is designed to handle multiple concurrent users efficiently, making it suitable for high-traffic scenarios.
-
Monetization Potential: The template can be easily adapted into a freemium model, offering basic conversions for free and premium features (like higher quality downloads or batch processing) for paid users, creating a revenue stream.
Technologies
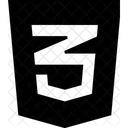
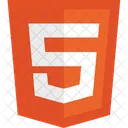