by egetsemani
My Daily Planner
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the Daily Planner app benefit small businesses or freelancers?
The Daily Planner app can significantly improve productivity for small businesses and freelancers by providing a simple, intuitive interface for task management. Users can easily organize their daily tasks, prioritize work, and maintain a clear overview of their schedule. This can lead to better time management, reduced stress, and increased efficiency in completing projects and meeting deadlines.
Can the Daily Planner be customized for specific industries or professions?
Absolutely! The Daily Planner's modular design allows for easy customization to suit various industries or professions. For example, a law firm could add case management features, while a creative agency might include project milestones and client deadlines. The app's flexible structure makes it simple to adapt the planner to specific needs while maintaining its core functionality.
What are the potential monetization strategies for the Daily Planner app?
There are several monetization strategies that could be implemented for the Daily Planner:
How can I add a new task to the Daily Planner's task list?
To add a new task to the Daily Planner, you'll need to implement a function to handle task creation and update the tasks list. Here's an example of how you could modify the home.js
file to add this functionality:
```javascript document.addEventListener('DOMContentLoaded', () => { // ... existing code ...
const addTaskBtn = document.querySelector('.add-task-btn');
const tasksList = document.getElementById('tasks-list');
addTaskBtn.addEventListener('click', () => {
const taskText = prompt('Enter a new task:');
if (taskText) {
const taskItem = document.createElement('div');
taskItem.classList.add('task-item');
taskItem.innerHTML = `
<input type="checkbox" id="task-${Date.now()}">
<label for="task-${Date.now()}">${taskText}</label>
`;
tasksList.appendChild(taskItem);
// Remove the "No tasks" message if it exists
const noTasksMessage = tasksList.querySelector('.no-tasks-message');
if (noTasksMessage) {
noTasksMessage.remove();
}
}
});
}); ```
This code adds an event listener to the "+" button, prompts the user for a new task, and adds it to the task list.
How can I implement user authentication in the Daily Planner app?
To implement user authentication in the Daily Planner, you can use Flask-Login, a popular extension for handling user sessions. Here's a basic example of how to set it up:
First, install Flask-Login:
pip install flask-login
Then, in your app_init.py
file, add the following:
```python from flask_login import LoginManager, UserMixin
def create_initialized_flask_app(): app = Flask(name, static_folder='static') # ... existing code ...
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
password = db.Column(db.String(120), nullable=False)
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
# ... rest of the function ...
return app
```
This sets up a basic User model and configures Flask-Login. You'll need to create additional routes for login, logout, and registration, as well as protect your existing routes with the @login_required
decorator to ensure only authenticated users can access the Daily Planner features.
Created: | Last Updated:
Here's a step-by-step guide on how to use the My Daily Planner template:
Introduction
The My Daily Planner template provides a user-friendly daily planner app featuring a basic task list view to help users organize their daily tasks. This template includes a responsive web interface with both desktop and mobile views, allowing users to easily manage their tasks on various devices.
Getting Started
To begin using this template, follow these steps:
-
Click "Start with this Template" to load the template into your Lazy Builder interface.
-
Press the "Test" button to initiate the deployment process. This will launch the Lazy CLI and begin setting up your app.
Using the App
Once the app is deployed, you'll be provided with a dedicated server link to access your Daily Planner. Here's how to use the interface:
-
Open the provided link in your web browser.
-
You'll see the main planner interface, which includes:
- The current date displayed at the top
- A "Today's Tasks" section
-
An "Add Task" button ("+")
-
To add a new task:
- Click the "+" button
-
Enter your task details (Note: The actual task addition functionality is not implemented in this template and would need to be added separately)
-
The app is responsive, so you can use it on both desktop and mobile devices:
- On desktop, you'll see a full navigation menu
- On mobile, you'll see a hamburger menu icon that expands to show navigation options
Customizing the App
While this template provides a basic structure for a daily planner, you may want to extend its functionality. Here are some areas you might consider customizing:
- Implement the task addition functionality
- Add a backend API to store and retrieve tasks
- Implement task editing and deletion features
- Add user authentication to allow multiple users to have their own planners
To make these customizations, you'll need to modify the existing code within the Lazy Builder interface. Focus on the following files:
home.html
: To adjust the frontend structurehome.js
: To add client-side functionality for task managementroutes.py
: To add backend routes for task CRUD operationsmodels.py
: To define database models for tasks
Remember to test your changes frequently using the "Test" button to ensure your app continues to function as expected.
By following these steps and customizing the template to your needs, you can create a fully functional daily planner app using the Lazy platform.
Here are 5 key business benefits for this Daily Planner template:
Template Benefits
-
Improved Productivity: By providing a clean, user-friendly interface for daily task management, this planner helps users organize their day more effectively, potentially increasing overall productivity.
-
Mobile Responsiveness: The template includes both desktop and mobile layouts, ensuring a seamless experience across devices. This flexibility can lead to increased user engagement and retention.
-
Easy Customization: With a modular structure and separate CSS file, businesses can quickly customize the look and feel to match their brand, saving time and resources in development.
-
Scalability: The use of Flask and SQLAlchemy provides a solid foundation for future feature additions, such as user accounts, task sharing, or integration with other productivity tools.
-
Cost-Effective Solution: As a ready-to-use template, it offers a quick starting point for businesses looking to launch a task management tool, reducing initial development costs and time-to-market.
Technologies
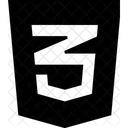
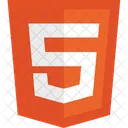