Mi Planificador Semanal de Comidas
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
¿Cómo puede "Mi Planificador Semanal de Comidas" beneficiar a los usuarios en su vida diaria?
"Mi Planificador Semanal de Comidas" puede mejorar significativamente la calidad de vida de los usuarios al ayudarles a organizar mejor sus comidas, ahorrar tiempo en la planificación y compras, y mantener una dieta más equilibrada. Al ofrecer opciones saludables y un contador de calorías, la aplicación fomenta hábitos alimenticios más saludables, lo que puede llevar a una mejor salud general y bienestar.
¿Cuál es el mercado objetivo para "Mi Planificador Semanal de Comidas" y cómo se puede monetizar?
El mercado objetivo principal para "Mi Planificador Semanal de Comidas" son adultos hispanohablantes interesados en mejorar sus hábitos alimenticios y optimizar su tiempo. Esto incluye profesionales ocupados, familias y personas conscientes de su salud. La monetización puede lograrse a través de un modelo freemium, donde las funciones básicas son gratuitas, pero se ofrecen características premium como planes de comidas personalizados o integración con servicios de entrega de alimentos por una suscripción mensual.
¿Cómo se puede implementar la funcionalidad de búsqueda de videos en "Mi Planificador Semanal de Comidas"?
Aunque "Mi Planificador Semanal de Comidas" no incluye actualmente una funcionalidad de búsqueda de videos, se podría implementar fácilmente utilizando la estructura existente en el archivo routes.py
. Por ejemplo, se podría modificar la ruta /search
para buscar recetas en video:
python
@app.route("/search", methods=['POST'])
def search_recipes():
query = request.json.get('query', '')
# Implementar lógica de búsqueda de recetas en video
recipe_results = [
{
'id': 'abc123',
'title': 'Ensalada Mediterránea',
'chef': 'Chef María',
'thumbnail': 'https://example.com/thumbnail.jpg'
}
]
return jsonify(recipe_results)
Esta funcionalidad permitiría a los usuarios buscar videos de recetas relacionadas con su plan de comidas.
¿Qué estrategias de marketing serían efectivas para promocionar "Mi Planificador Semanal de Comidas"?
Para promocionar "Mi Planificador Semanal de Comidas", se podrían implementar las siguientes estrategias: - Marketing de contenidos: crear un blog con consejos de nutrición y recetas saludables. - Colaboraciones con influencers de salud y fitness en redes sociales. - Campañas de publicidad dirigidas en plataformas como Facebook e Instagram. - Optimización para motores de búsqueda (SEO) para aumentar la visibilidad orgánica. - Programas de referidos para incentivar a los usuarios actuales a invitar a sus amigos.
¿Cómo se podría implementar un sistema de autenticación de usuarios en "Mi Planificador Semanal de Comidas"?
Para implementar un sistema de autenticación en "Mi Planificador Semanal de Comidas", se podría utilizar Flask-Login. Primero, se agregaría un modelo de Usuario a models.py
:
```python from flask_login import UserMixin
class User(UserMixin, db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(64), unique=True, nullable=False) password_hash = db.Column(db.String(128))
def set_password(self, password):
self.password_hash = generate_password_hash(password)
def check_password(self, password):
return check_password_hash(self.password_hash, password)
```
Luego, se agregarían rutas para login y registro en routes.py
:
```python from flask_login import login_user, logout_user, login_required
@app.route('/login', methods=['GET', 'POST']) def login(): # Implementar lógica de login pass
@app.route('/register', methods=['GET', 'POST']) def register(): # Implementar lógica de registro pass
@app.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('home')) ```
Esto permitiría a los usuarios crear cuentas personales y guardar sus planes de comidas en "Mi Planificador Semanal de Comidas".
Created: | Last Updated:
Here's a step-by-step guide for using the Mi Planificador Semanal de Comidas template:
Introduction
The Mi Planificador Semanal de Comidas template provides a foundation for building a weekly meal planner application in Spanish. It includes features for searching videos, displaying search results, and showing lyrics for selected songs. This guide will walk you through the process of setting up and using the template.
Getting Started
- Click "Start with this Template" to begin using the Mi Planificador Semanal de Comidas template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the Application
Once the application is deployed, you can access it through the provided server link. The application includes the following features:
- Home Page:
- The home page displays a search bar where users can enter queries to search for videos.
-
Enter a search query and click the "Buscar" button or press Enter to perform a search.
-
Search Results:
- After performing a search, the results will be displayed as video cards.
- Each video card shows the video thumbnail, title, and channel name.
-
Click the "Seleccionar" button on a video card to play the selected video.
-
Video Player:
- When a video is selected, it will be displayed in an embedded YouTube player.
-
The video player allows users to watch the selected video directly within the application.
-
Lyrics Display:
- After selecting a video, the application will attempt to fetch and display lyrics for the chosen song.
- If lyrics are available, they will be shown below the video player.
- If lyrics are not available, a message indicating this will be displayed.
Customization and Extension
To customize and extend the application:
- Modify the HTML templates:
- Edit the
home.html
file to change the layout and structure of the home page. -
Update the
_header.html
,_desktop_header.html
, and_mobile_header.html
files to modify the header and navigation components. -
Enhance the JavaScript functionality:
- Modify the
karaoke.js
file to add new features or change existing behavior. -
Update the search functionality, video selection process, or lyrics display as needed.
-
Customize the styling:
- Edit the
styles.css
file to change the appearance of the application. -
Adjust colors, fonts, layouts, and other visual elements to match your desired design.
-
Implement the YouTube API search:
- In the
routes.py
file, replace the mock data in thesearch_videos
function with actual YouTube API integration. - You'll need to obtain YouTube API credentials and use a library like
google-api-python-client
to make API requests.
- In the
-
Add lyrics fetching functionality:
- In the
routes.py
file, implement the logic to fetch lyrics in theget_lyrics
function. - You may need to integrate with a lyrics API or service to retrieve actual song lyrics.
- In the
By following these steps, you can set up, use, and customize the Mi Planificador Semanal de Comidas template to create a functional weekly meal planner application with video search and lyrics display capabilities.
Here are 5 key business benefits for this template:
Template Benefits
-
Karaoke Platform: This template provides the foundation for building a web-based karaoke application, allowing businesses to create an interactive music experience for users.
-
Video Search Integration: The search functionality can be leveraged to integrate with YouTube's API, enabling businesses to offer a vast library of music videos to their users.
-
Lyrics Display: The template includes a lyrics panel, which can be expanded to provide synchronized lyrics, enhancing the karaoke experience and potentially attracting more users.
-
Scalable Architecture: Built with Flask and SQLAlchemy, the template offers a scalable backend architecture that can handle growth in user base and feature set.
-
Mobile-Responsive Design: The inclusion of both desktop and mobile header components ensures the application is accessible across various devices, maximizing potential user reach and engagement.
Technologies
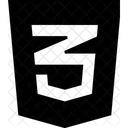
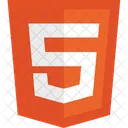