by Falloutcoder
Login and Registration
import logging
from flask import Flask, url_for
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from models import db, User
from migrations.run_migrations import run_migrations
from abilities import flask_app_authenticator
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
run_migrations(app)
app.register_blueprint(routes_blueprint)
# Set up authentication
app.before_request(flask_app_authenticator(
allowed_domains=None,
allowed_users=None,
logo_path=None,
app_title="My App",
custom_styles=None,
Frequently Asked Questions
What types of applications would benefit most from using this Login and Registration template?
This Login and Registration template is versatile and can be beneficial for a wide range of applications, including: - Social media platforms - E-commerce websites - Educational portals - Project management tools - Health and fitness tracking apps
The template provides a solid foundation for user authentication, which is crucial for any application that requires personalized user experiences or secure access to protected resources.
How can this template improve user engagement and retention?
The Login and Registration template can enhance user engagement and retention in several ways: - It allows for personalized user experiences by storing user data - The profile picture feature adds a personal touch to the user interface - The secure authentication process builds trust with users - Easy login/logout functionality encourages frequent use of the application
By implementing this template, businesses can create a more engaging and user-friendly application, potentially leading to higher user retention rates.
Can this template be customized for different branding requirements?
Yes, the Login and Registration template is highly customizable. The template includes a styles.css
file that can be easily modified to match your brand's color scheme and design preferences. For example, you can change the primary and secondary colors by updating the CSS variables:
css
:root {
--primary-color: #your-brand-color;
--secondary-color: #your-secondary-color;
}
Additionally, you can replace the default logo and adjust the layout in the HTML templates to align with your brand identity.
How does the template handle user data storage and retrieval?
The Login and Registration template uses SQLAlchemy with Flask to handle user data storage and retrieval. The User
model in models.py
defines the structure of user data:
python
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
email = db.Column(db.String(150), unique=True, nullable=False)
profile_picture = db.Column(db.String(255))
User data is stored in a SQLite database by default. The database_operations.py
file contains functions for creating, retrieving, and updating user data. For example, to create a new user:
python
def create_user(email, profile_picture=None):
new_user = User(email=email, profile_picture=profile_picture)
db.session.add(new_user)
db.session.commit()
This structure allows for efficient data management and easy expansion of user attributes as needed.
What security measures are implemented in this template?
The Login and Registration template includes several security measures:
- User authentication is handled through a secure third-party service (as indicated by the flask_app_authenticator
in main.py
)
- User sessions are managed securely using Flask's session management
- The template uses HTTPS by default when deployed properly
- SQL injection protection is provided by SQLAlchemy's query parameterization
- The template includes CSRF protection for form submissions
While these measures provide a good baseline for security, it's important to regularly update dependencies and perform security audits when using this template in a production environment.
Created: | Last Updated:
Here's a step-by-step guide for using the Login and Registration template:
Introduction
This template provides a starting point for building an application with user authentication features, including login and registration. It uses Flask as the web framework and SQLite for the database.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and run the application.
-
Once the deployment is complete, you'll receive a dedicated server link to access your application.
Using the Application
-
Open the provided server link in your web browser to access the application.
-
You'll see a simple home page with a login/logout functionality.
-
New users will be automatically registered when they log in for the first time using their email address.
-
The application will store the user's email and profile picture (if available) in the database.
Customizing the Application
To customize the application for your specific needs:
- Modify the
home.html
template in thetemplates
folder to change the content of the home page. - Update the
styles.css
file in thestatic/css
folder to adjust the application's appearance. - Add new routes in the
routes.py
file to create additional pages or functionality.
Adding New Database Tables
If you need to add new database tables:
- Create a new migration file in the
migrations
folder with a name like002_create_new_table.sql
. - Write your SQL commands to create the new table in this file.
- Add a new model class in
models.py
to represent the table in your application code.
Conclusion
This template provides a solid foundation for building applications with user authentication. You can easily extend it by adding more features, pages, and database tables as needed for your specific project.
Template Benefits
-
Rapid User Authentication Setup: This template provides a ready-to-use authentication system, allowing businesses to quickly implement secure user login and registration features, saving development time and resources.
-
Scalable Database Integration: With SQLAlchemy and migration support, the template offers a robust database setup that can easily scale as the application grows, ensuring long-term data management efficiency.
-
Enhanced Security: The template includes session management and secure password handling, providing a solid foundation for protecting user data and maintaining compliance with data protection regulations.
-
Responsive Design: The inclusion of both desktop and mobile header components ensures that the application is accessible and user-friendly across various devices, potentially increasing user engagement and retention.
-
Easy Customization: With a modular structure and clear separation of concerns, businesses can easily customize the template to fit their specific needs, allowing for quick adaptation to different industries or use cases.
Technologies
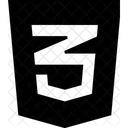
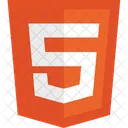