IndexNow With Bing
import logging
import os
from typing import Optional
from urllib.parse import urlparse
import aiohttp
from fastapi import FastAPI, HTTPException
from fastapi.responses import RedirectResponse
from pydantic import BaseModel, HttpUrl
from abilities import apply_sqlite_migrations
from models import Base, engine
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI(
title="SEO Indexing API",
description="Submit URLs for immediate indexing to Bing search engine",
version="1.0.0"
)
# Initialize Bing API key
bing_api_key = os.environ.get('BING_API_KEY')
if not bing_api_key:
Frequently Asked Questions
What is the main purpose of the Instant URL Indexer API?
The Instant URL Indexer API is designed to streamline the process of submitting URLs for immediate indexing to search engines, specifically Bing in this implementation. It provides a fast and efficient way for businesses to ensure their web pages are quickly discovered and indexed by search engines, potentially improving their SEO performance.
How can businesses benefit from using the Instant URL Indexer API?
Businesses can leverage the Instant URL Indexer API to: - Accelerate the indexing of new or updated content on their websites - Improve their search engine visibility more quickly - Automate the process of submitting URLs to search engines - Potentially enhance their SEO strategy by ensuring timely indexing of important pages
Is the Instant URL Indexer API limited to Bing, or can it be extended to other search engines?
While the current implementation of the Instant URL Indexer API focuses on Bing, the modular design of the template allows for easy extension to other search engines. Developers can add additional submission functions for other search engines, following a similar pattern to the submit_to_bing
function, to create a comprehensive multi-engine indexing solution.
How can I add a new model to the Instant URL Indexer API for tracking submitted URLs?
To add a new model for tracking submitted URLs, you can modify the models.py
file. Here's an example of how you might add a SubmittedURL
model:
```python from sqlalchemy import Column, Integer, String, DateTime from sqlalchemy.sql import func from models import Base
class SubmittedURL(Base): tablename = 'submitted_urls'
id = Column(Integer, primary_key=True)
url = Column(String, nullable=False)
submitted_at = Column(DateTime(timezone=True), server_default=func.now())
status = Column(String)
```
After adding this model, you'll need to create a new migration file in the migrations
folder to apply this change to your database schema.
How can I extend the Instant URL Indexer API to include rate limiting for submissions?
To implement rate limiting in the Instant URL Indexer API, you can use FastAPI's dependencies along with a third-party library like slowapi
. Here's an example of how you might modify the submit_url
endpoint to include rate limiting:
```python from fastapi import Depends from slowapi import Limiter from slowapi.util import get_remote_address
limiter = Limiter(key_func=get_remote_address) app.state.limiter = limiter
@app.post("/submit-url") @limiter.limit("5/minute") async def submit_url(submission: URLSubmission, request: Request): # Existing function code here ```
This example limits submissions to 5 per minute per IP address. You'll need to add slowapi
to your requirements.txt
file and import the necessary components. The Instant URL Indexer API can thus be enhanced with rate limiting to prevent abuse and ensure fair usage of the service.
Created: | Last Updated:
Here's a step-by-step guide for using the Instant URL Indexer API template:
Introduction
The Instant URL Indexer API is a FastAPI-based backend service that allows you to submit URLs for immediate indexing to the Bing search engine. This template provides a simple and efficient way to ensure your web pages are quickly indexed and discoverable through Bing's search results.
Getting Started
- Click "Start with this Template" to begin using the Instant URL Indexer API template in your Lazy project.
Initial Setup
Before you can use the API, you need to set up your Bing API key:
- Go to the Bing Webmaster Tools website (https://www.bing.com/webmaster/home/mysites) and sign in or create an account.
- Navigate to the "API Access" section.
- Generate a new API key if you don't already have one.
- In the Lazy Builder interface, go to the "Environment Secrets" tab.
- Add a new secret with the key
BING_API_KEY
and paste your Bing API key as the value.
Test the API
- Click the "Test" button in the Lazy Builder interface to deploy and start your API.
- Once the deployment is complete, you'll receive a server link to access your API.
Using the API
After deployment, you can interact with your API using the provided server link. The API offers a single endpoint for URL submission:
- Endpoint:
/submit-url
- Method: POST
- Request body: JSON object with a
url
field containing the URL to be indexed
Sample Request
```http POST /submit-url Content-Type: application/json
{ "url": "https://example.com/my-new-page" } ```
Sample Response
json
{
"url": "https://example.com/my-new-page",
"bing_indexing": {
"status": "success",
"response": {
"status": "success",
"message": "URL submitted successfully to Bing"
}
}
}
Integrating the API
To integrate this API into your workflow:
- Use the server link provided by Lazy to make HTTP requests to your API.
- Implement a mechanism in your content management system or publishing workflow to automatically submit new or updated URLs to this API.
- You can use any programming language or tool that supports making HTTP requests to interact with this API.
Example integration using Python and the requests
library:
```python import requests
api_url = "YOUR_LAZY_SERVER_LINK/submit-url" url_to_index = "https://example.com/my-new-page"
response = requests.post(api_url, json={"url": url_to_index}) print(response.json()) ```
Replace YOUR_LAZY_SERVER_LINK
with the actual server link provided by Lazy after deployment.
By following these steps, you'll have a functioning URL indexing API that submits URLs to Bing for faster indexing. This can significantly improve the discoverability of your web pages in Bing search results.
Here are 5 key business benefits for this template:
Template Benefits
-
Improved Search Engine Visibility: By providing instant URL submission to search engines like Bing, businesses can accelerate the indexing process, ensuring their web content appears in search results more quickly. This can lead to increased organic traffic and better online visibility.
-
SEO Optimization: The template offers a streamlined way to submit new or updated content for indexing, which is crucial for maintaining an up-to-date search engine presence. This can significantly enhance a company's SEO efforts and improve their search rankings.
-
API Integration Capability: With its FastAPI backend, this template can be easily integrated into existing content management systems or publishing workflows. This allows businesses to automate the indexing process, saving time and ensuring consistency in their SEO practices.
-
Scalable Infrastructure: Built on FastAPI and using asynchronous programming, this template provides a scalable solution that can handle high volumes of URL submissions efficiently. This makes it suitable for businesses of all sizes, from small startups to large enterprises with extensive web presences.
-
Error Handling and Logging: The template includes robust error handling and logging features, which can help businesses troubleshoot issues quickly and maintain reliable operations. This can lead to improved uptime and a better understanding of their indexing processes.
Technologies
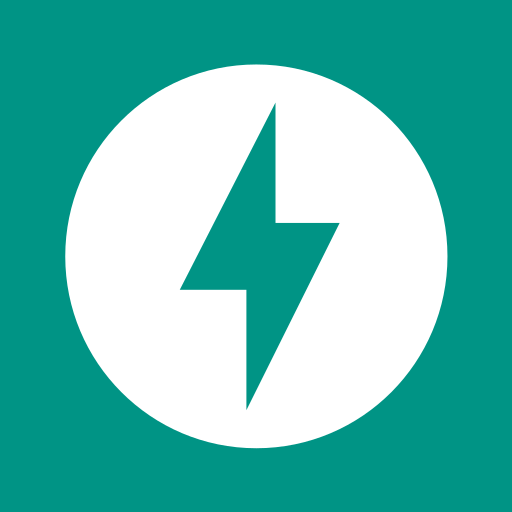